Question
Hi, I'm stuck on the reserverAnimals and printAnimals method they are the last sections of code. Any help would be appreciated, I've tried everything I
Hi, I'm stuck on the reserverAnimals and printAnimals method they are the last sections of code. Any help would be appreciated, I've tried everything I can think of and nothing works.
import java.util.ArrayList; import java.util.Scanner;
public class Driver { private static Scanner scanner = new Scanner(System.in);
private static ArrayList dogList = new ArrayList(); // ArrayList for monkeys private static ArrayList monkeyList = new ArrayList();
// Instance variables (if needed)
public static void main(String[] args) {
String menuChoice = "";
initializeDogList(); initializeMonkeyList();
while ( !menuChoice.equalsIgnoreCase("q")) { displayMenu(); menuChoice = scanner.nextLine(); // gets menu choice switch(menuChoice) { case "1": intakeNewDog(scanner); break; case "2": intakeNewMonkey(scanner); break; case "3": reserveAnimal(scanner); break; case "4": printAnimals(); break; case "5": printAnimals(); break; case "6": printAnimals(); break; default: if(!menuChoice.equalsIgnoreCase("q")) { System.out.println(" Invalid input, please try again"); } break; } } System.out.println("Goodbye. Thank youfor using Grazioso Salvare Rescue Animals"); } // This method prints the menu options public static void displayMenu() { System.out.println(" "); System.out.println("\t\t\t\tRescue Animal System Menu"); System.out.println("[1] Intake a new dog"); System.out.println("[2] Intake a new monkey"); System.out.println("[3] Reserve an animal"); System.out.println("[4] Print a list of all dogs"); System.out.println("[5] Print a list of all monkeys"); System.out.println("[6] Print a list of all animals that are not reserved"); System.out.println("[q] Quit application"); System.out.println(); System.out.println("Enter a menu selection"); }
// Adds dogs to a list for testing public static void initializeDogList() { Dog dog1 = new Dog("Spot", "German Shepherd", "male", "1", "25.6", "05-12-2019", "United States", "intake", false, "United States"); Dog dog2 = new Dog("Rex", "Great Dane", "male", "3", "35.2", "02-03-2020", "United States", "Phase I", false, "United States"); Dog dog3 = new Dog("Bella", "Chihuahua", "female", "4", "25.6", "12-12-2019", "Canada", "in service", true, "Canada");
dogList.add(dog1); dogList.add(dog2); dogList.add(dog3); }
// Adds monkeys to a list for testing //Optional for testing public static void initializeMonkeyList() { Monkey monkey1 = new Monkey("Clyde", "Guenon", "5.1", "8.4", "19.6", "male", "2", "15.3", "08-21-2019", "Australia", "Phase III", true, "Canada"); Monkey monkey2 = new Monkey("Chia", "Macaque", "4.8", "10.2", "19.3", "female", "1", "13.4", "11-15-2018", "United Kingdom", "in service", false, "United Kingdom"); Monkey monkey3 = new Monkey("Pat", "Tamarin", "5.5", "10.6", "13.7", "female", "3", "18.2", "01-04-2021", "United States", "intake", false, "United States");
monkeyList.add(monkey1); monkeyList.add(monkey2); monkeyList.add(monkey3); }
// Complete the intakeNewDog method // The input validation to check that the dog is not already in the list // is done for you public static void intakeNewDog(Scanner scanner) { System.out.println("What is the dog's name?"); String name = scanner.nextLine(); for(Dog dog: dogList) { if(dog.getName().equalsIgnoreCase(name)) { System.out.println(" This dog is already in our system "); return; //returns to menu } } System.out.println("What is " + name + "'s breed?"); String breed = scanner.nextLine(); System.out.println("What is " + name + "'s gender?"); String gender = scanner.nextLine(); System.out.println("What is " + name + "'s age?"); String age = scanner.nextLine(); System.out.println("What is " + name + "'s weight?"); String weight = scanner.nextLine(); System.out.println("What is " + name + "'s acquisition date?"); String acqDate = scanner.nextLine(); System.out.println("What is " + name + "'s acquisition country?"); String acqCountry = scanner.nextLine(); System.out.println("What is " + name + "'s training status?"); String ts = scanner.nextLine(); System.out.println("Is " + name + " reserved?"); boolean res = scanner.nextBoolean();scanner.nextLine(); System.out.println("What is " + name + "'s in Service Country?"); String isc = scanner.nextLine(); Dog newdog=new Dog(name,breed,gender,age,weight,acqDate,acqCountry,ts,res,isc); dogList.add(newdog); }
public static void intakeNewMonkey(Scanner scanner) { System.out.println("What is the monkey's name?"); //User input Monkey's name String name = scanner.nextLine(); for(Monkey monkey: monkeyList) { // Avoids duplicate monkey entry if(monkey.getName().equalsIgnoreCase(name)) { System.out.println(" This monkey is already in our system "); return; //returns to menu } } System.out.println("What is " + name + "'s species?"); String species = scanner.nextLine(); if(!(species.equalsIgnoreCase("Capuchin")) && !(species.equalsIgnoreCase("Guenon")) && !(species.equalsIgnoreCase("Macaque")) && !(species.equalsIgnoreCase("Marmoset")) && !(species.equalsIgnoreCase("Squirrel Monkey")) && !(species.equalsIgnoreCase("Tamarin"))){ System.out.println(" This monkey's species is not allowed "); // Only allows correct monkey return; } System.out.println("What is " + name + "'s tail length?"); // user input tail length String tailLength = scanner.nextLine(); System.out.println("What is " + name + "'s height?"); // user input height String height = scanner.nextLine(); System.out.println("What is " + name + "'s body length?"); // user input body length String bodyLength = scanner.nextLine(); System.out.println("What is " + name + "'s gender?"); // user input gender String gender = scanner.nextLine(); System.out.println("What is " + name + "'s age?"); // user input age String age = scanner.nextLine(); System.out.println("What is " + name + "'s weight?"); // user input weight String weight = scanner.nextLine(); System.out.println("What is " + name + "'s acquisition date?"); // user input acquired date String acquisitionDate = scanner.nextLine(); System.out.println("What is " + name + "'s acquisition country?"); // user input where monkey came from String acquisitionCountry = scanner.nextLine(); System.out.println("What is " + name + "'s training status?"); // user input training level String trainingStatus = scanner.nextLine(); System.out.println("Is " + name + " reserved?"); // user input reserved boolean reserved = scanner.nextBoolean();scanner.nextLine(); System.out.println("What is " + name + "'s in Service Country?"); // user input where is monkey working String inServiceCountry = scanner.nextLine(); Monkey newmonkey=new Monkey(name,species,tailLength,height,bodyLength,gender,age,weight,acquisitionDate,acquisitionCountry,trainingStatus,reserved,inServiceCountry); monkeyList.add(newmonkey); }
// Complete reserveAnimal // You will need to find the animal by animal type and in service country public static void reserveAnimal(Scanner scanner) { }
// Complete printAnimals // Include the animal name, status, acquisition country and if the animal is reserved. // Remember that this method connects to three different menu items. // The printAnimals() method has three different outputs // based on the listType parameter // dog - prints the list of dogs // monkey - prints the list of monkeys // available - prints a combined list of all animals that are // fully trained ("in service") but not reserved // Remember that you only have to fully implement ONE of these lists. // The other lists can have a print statement saying "This option needs to be implemented". // To score "exemplary" you must correctly implement the "available" list. public static void printAnimals() { System.out.println("The method printAnimals needs to be implemented"); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
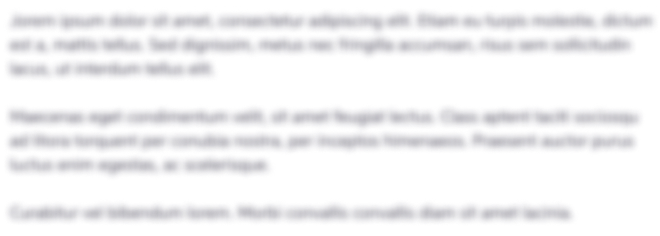
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started