Hi, please help me with the following question and code it in C++. And please if you are not getting the question don't answer it because I already received 3 incorrect answers and this is 4th time that I am posting this question.
NOTE: This question has a pre coded file that i am attaching the screenshots of that with this question. In order to make your work easier i post the precode file here that you can copy and paste it in your system as well. it is a request please make sure that the program output is exactly what the system asks because, the code is so lengthy, it will be difficult to find what is wrong. the system will check this code by 2 file name. they are rawgrades-starwars.csv and rawgrades-bw101.csv i am attaching the screenshots of those excel files along with the question as well. Thank you in advance
// PRECODE FILE
// grader.cpp
// -----------------------------------------------------------------------
#include
#include
#include
#include
using namespace std;
// -----------------------------------------------------------------------
// global constants
const string INFILE_PREFIX = "rawgrades-";
const string OUTFILE_PREFIX = "grade-report-";
const string IN_FILE_EXT = ".csv";
const string OUT_FILE_EXT = ".txt";
const size_t LEN_PREFIX = INFILE_PREFIX.length();
const size_t LEN_F_EXT = IN_FILE_EXT.length();
///this will be used for extra space between fields in the output file
const string SPACER = " ";
const double A_GRADE = 90.0;
const double B_GRADE = 80.0;
const double C_GRADE = 70.0;
const double D_GRADE = 60.0;
// anything below D_GRADE is an 'F'
// output field widths
const int FW_NAME = 24;
const int FW_SCORE = 4;
const int FW_LOW = 3;
const int FW_TOTAL = 5;
const int FW_AVG = 7;
const int FW_GRADE = 3;
const int FW_SCORE_X4 = FW_SCORE * 4;
// -----------------------------------------------------------------------
// function prototypes
// top-level functions
string getInputFilename();
string deriveOutputFilename(const string &inFname);
bool openFiles(const string &inName, const string &outName, ifstream &fin, ofstream &fout);
void processGradeFile(ifstream &fin, ofstream &fout);
// helper functions
// TODO (0): declare function prototypes for the helper functions
// -----------------------------------------------------------------------
// program entry point
int main() {
// main() has been implemented for you; no need to change anything here
ifstream fin;
ofstream fout;
string inFilename = getInputFilename();
string outFilename = deriveOutputFilename(inFilename);
if (!openFiles(inFilename, outFilename, fin, fout)) {
return EXIT_FAILURE;
}
cout
processGradeFile(fin, fout);
cout
fin.close();
fout.close();
return EXIT_SUCCESS;
}
// -----------------------------------------------------------------------
// helper function definitions
// Returns true if `fname` of the form "rawgrades-.csv"
bool validInputFilename(const string &fname) {
size_t nameLen = fname.length();
// TODO (1): implement validation on the given filename
///look for the presence of INFILE_PREFIX
///look for the presence of IN_FILE_EXT
/// ensure the name length is MORE than just the prefix and extension
// currently this assumes that all names are valid
// but this will fail the unit tests
return true;
}
// Parses a record, placing the substrings into `name`, `id`, and `s1...s4`
void parseStudentRecord(const string &line,
string &name, string &id, int &s1, int &s2, int &s3, int &s4) {
// TODO (2): carve up the input line into substrings
// placing the results into the appropriate reference variables
}
// Returns the smaller of the two values
int min(int a, int b) {
// TODO (3): return minimum value
return 0;
}
// Returns the smallest of all the values
int min(int a, int b, int c, int d) {
// TODO (4): return minimum value
// HINT: use min(int, int)
return 0;
}
// Returns a letter grade for the given score
char gradeFromScore(double score) {
char grade = 'F';
// TODO (5): adjust grade based on score
return grade;
}
// Processes a line read from the raw-grades input file
void processLine(ostream &out, const string &line) {
string studentName, studentId, scores;
int score1, score2, score3, score4;
int total, lowest;
double average;
char letterGrade;
// TODO (6): process the raw record...
parseStudentRecord(line, studentName, studentId, score1, score2, score3, score4);
// - compute total (but subtract the lowest score)
// - compute the average (of 3 scores)
letterGrade = gradeFromScore(average);
// TODO (7): write the formatted record to the report file
//For the low score field, use:
}
// Writes the report header to the given output stream
void writeReportHeader(ofstream &out) {
// Lucky you! I've implemented this one for you. Don't change it! :)
out
out
out
out
out
}
// -----------------------------------------------------------------------
// top-level function definitions
// Returns a validated input filename from the user
string getInputFilename() {
string fname;
// TODO (8): Repeatedly prompt the user for a filename
// until it matches the required form.
return fname;
}
// Derives the output filename from the given input filename
string deriveOutputFilename(const string &inFname) {
// TODO (9): Generate the output file name
///grade-report-.txt
///use .replace to replace the prefix and the extension
return "FIXME-" + inFname;
}
// Returns true only if both files opened without error
bool openFiles(const string &inName, const string &outName, ifstream &fin, ofstream &fout) {
// TODO (10): open input and output files, associate with the given streams
// HINT:
// open input; if fails, write message and return false
// open output; if fails, write message and return false
// return true
return false;
}
// Read input records, process, write formatted output records
void processGradeFile(ifstream &fin, ofstream &fout) {
// This function, too, has been implemented for you... nothing to do here
string rawLine;
writeReportHeader(fout);
while (getline(fin, rawLine)) {
processLine(fout, rawLine);
}
}
// -----------------------------------------------------------------------
// PRECODE FILE SCREENSHOTS
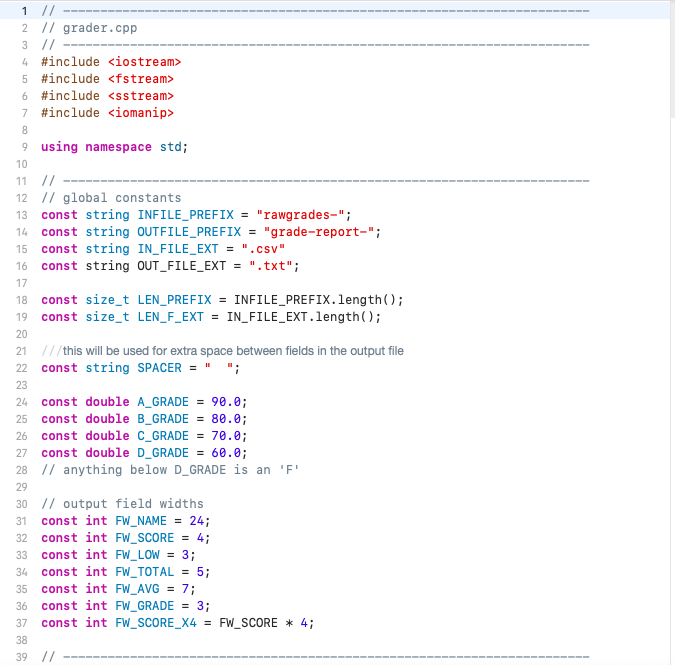
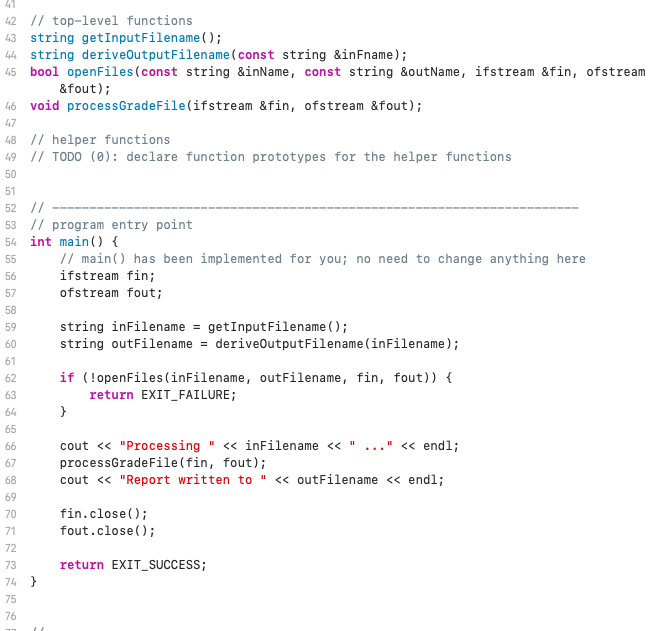
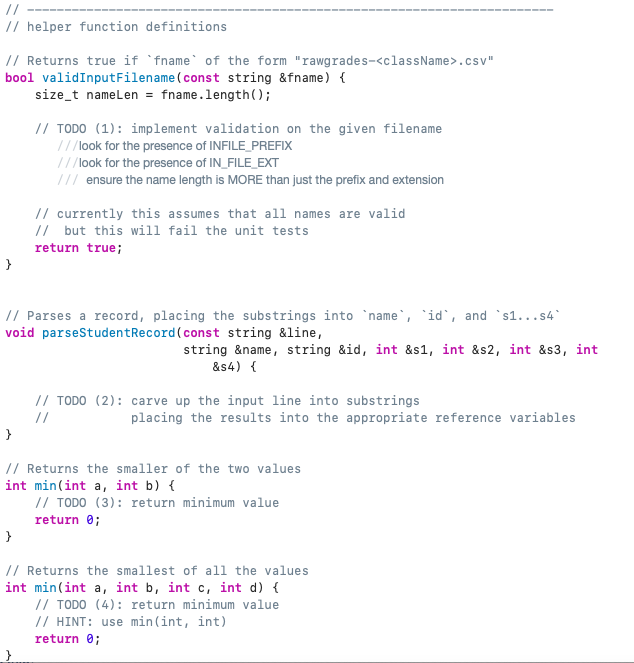
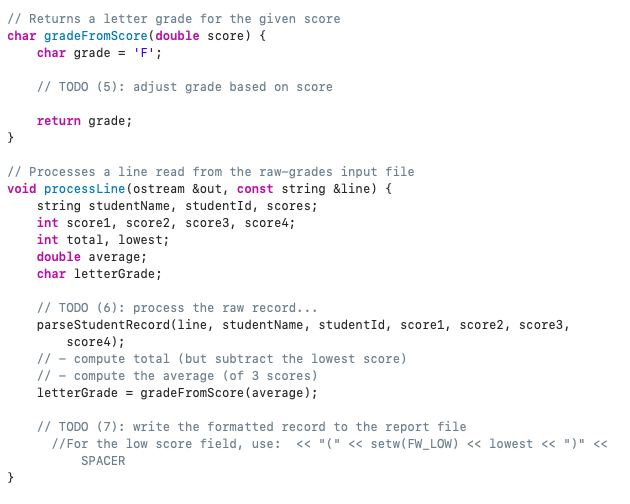
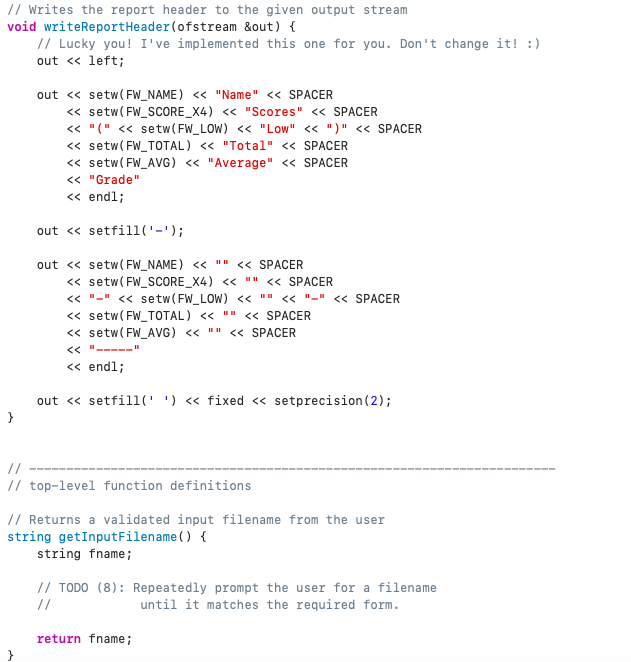
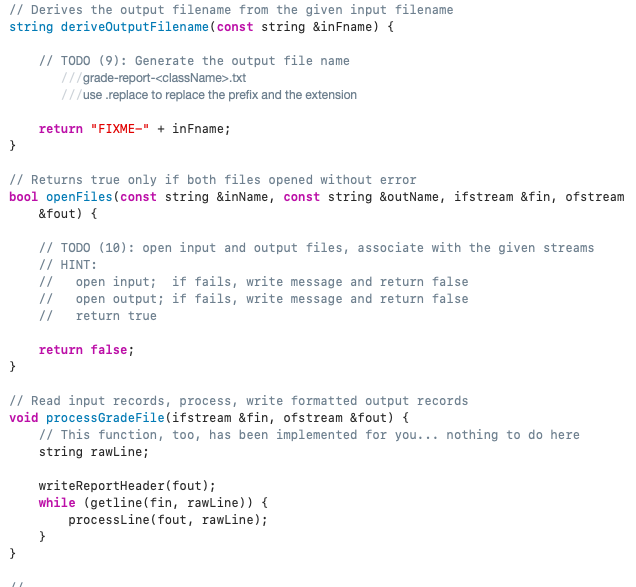
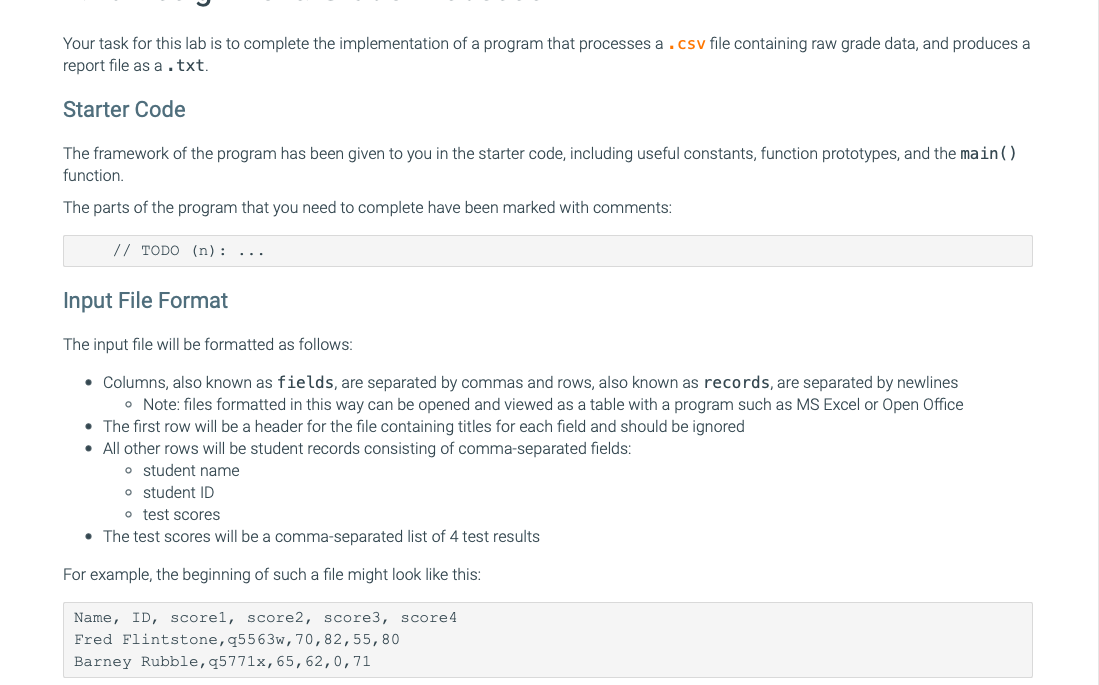
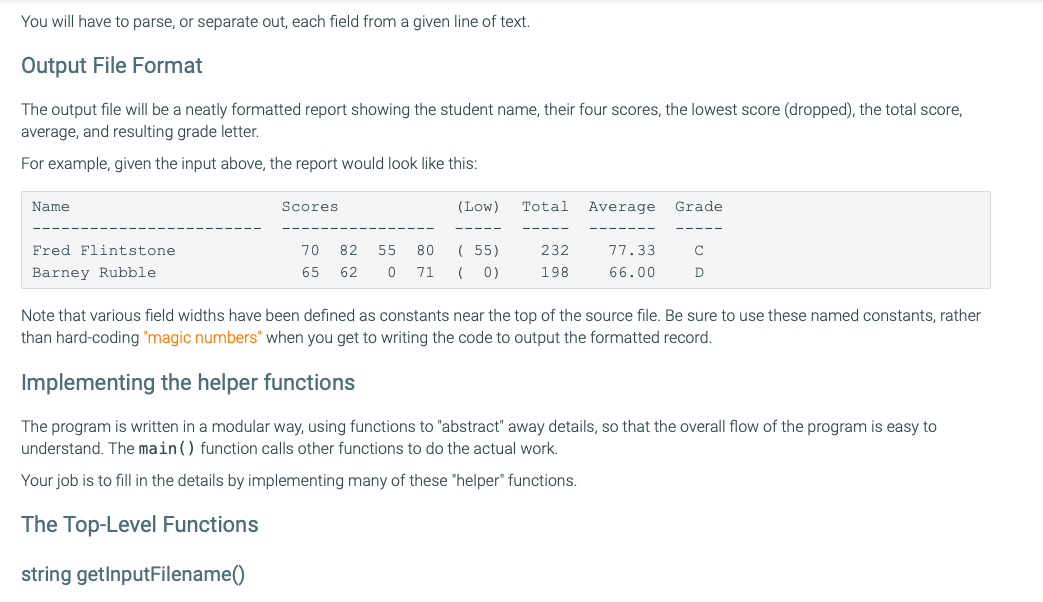
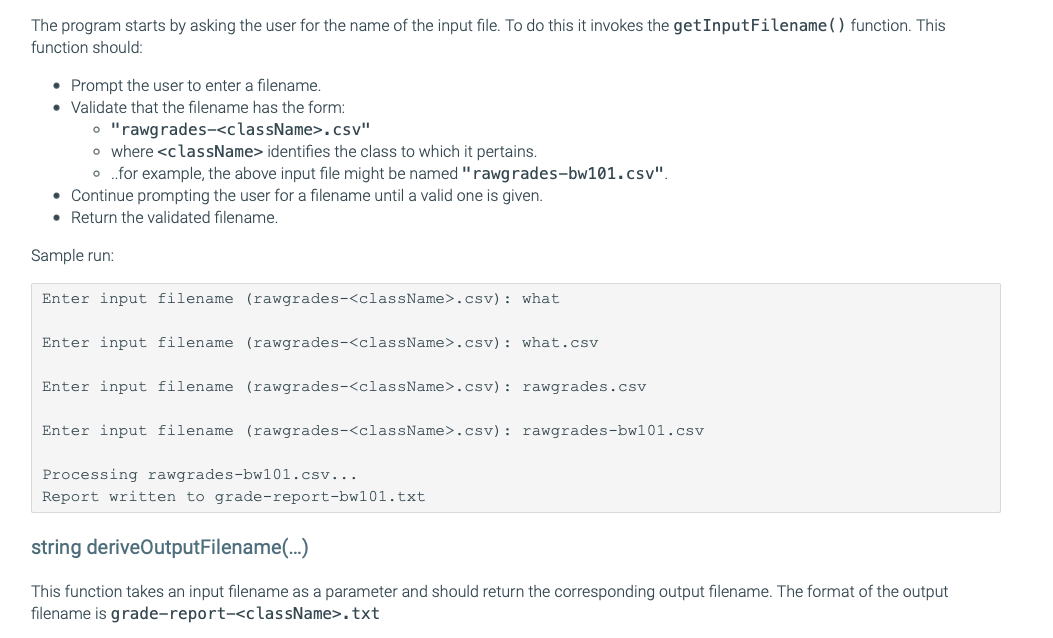
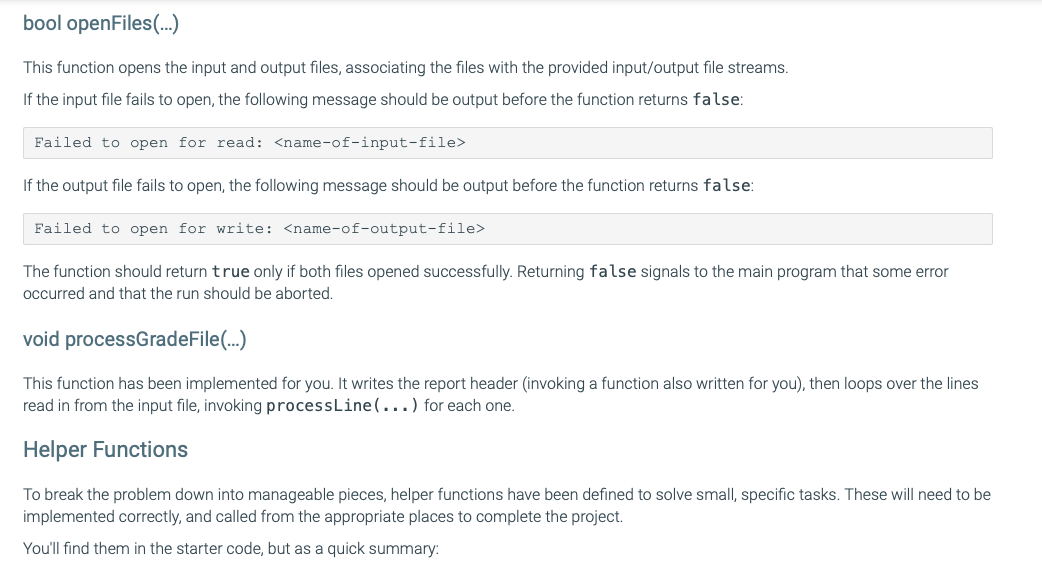
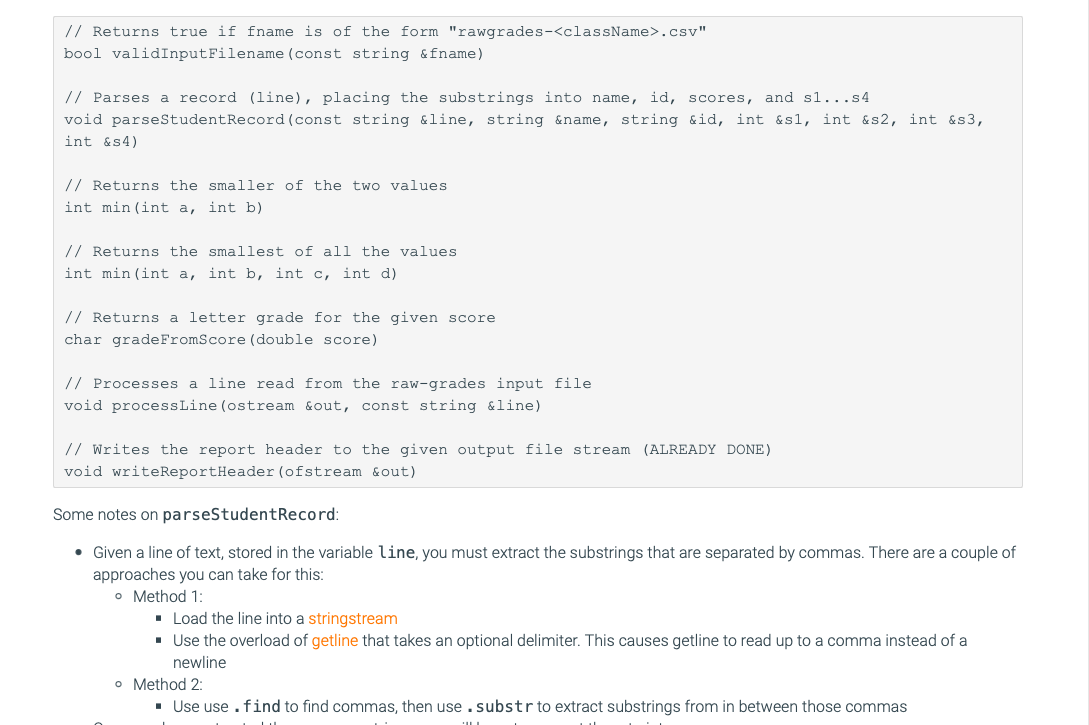
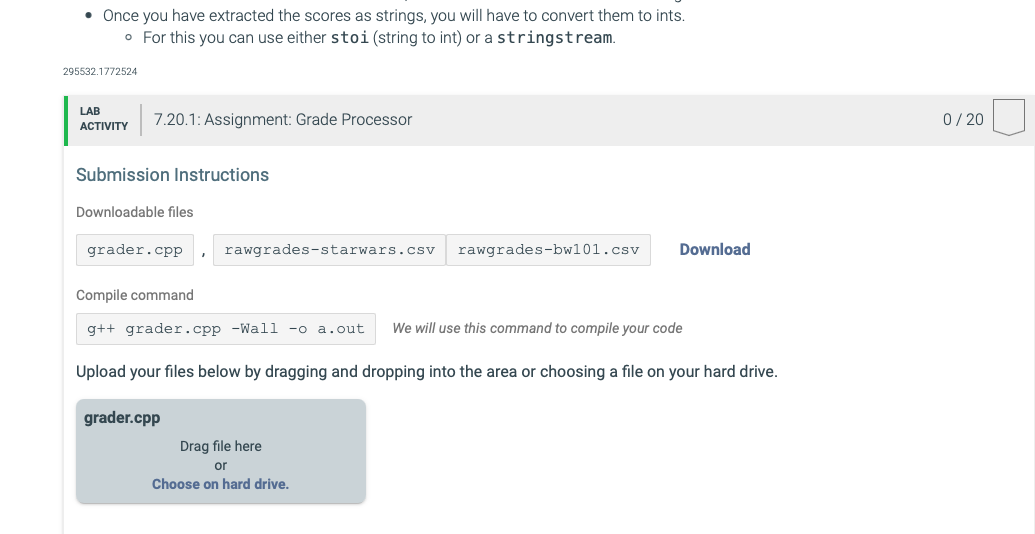
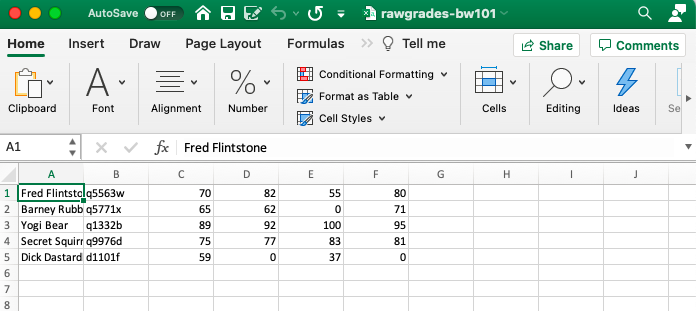
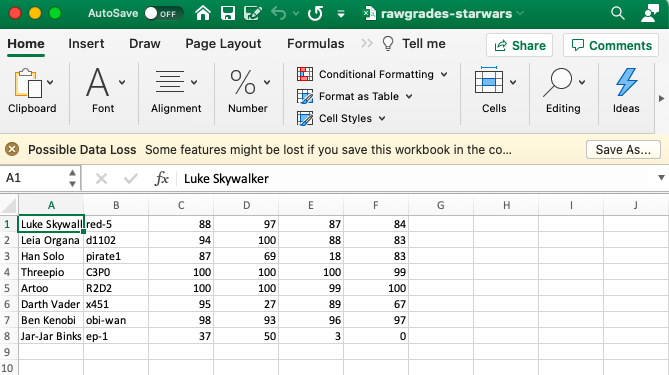
1 // 2 // grader.cpp 3 // 4 #include
5 #include 6 #include 7 #include 8 13 9 using namespace std; 10 11 // 12 // global constants const string INFILE_PREFIX = "rawgrades-"; 14 const string OUTFILE_PREFIX "grade-report-"; 15 const string IN_FILE_EXT = ".csv" 16 const string OUT_FILE_EXT = ".txt"; 17 18 const size_t LEN_PREFIX = INFILE_PREFIX. length(); 19 const size_t LEN_F_EXT = IN_FILE_EXT.length(); 20 21 ///this will be used for extra space between fields in the output file 22 const string SPACER = " "; 23 24 const double A_GRADE = 90.0; 25 const double B_GRADE = 80.0; 26 const double C_GRADE = 70.0; 27 const double D_GRADE = 60.0; 28 // anything below D_GRADE is an 'F' 29 30 // output field widths 31 const int FW_NAME = 24; 32 const int FW_SCORE = 4; 33 const int FW_LOW = 3; 34 const int FW_TOTAL = 5; 35 const int FW_AVG = 7; const int FW_GRADE = 3; 37 const int FW_SCORE_X4 = FW_SCORE * 4; 38 3911 36 42 // top-level functions 43 string getInputFilename(); 44 string deriveOutput Filename (const string &inFname); 45 bool openFiles(const string &inName, const string &outName, ifstream &fin, ofstream &fout); 46 void processGradeFile(ifstream &fin, ofstream &fout); 47 48 // helper functions 49 // TODO (): declare function prototypes for the helper functions 50 51 52 17 53 // program entry point 54 int main() { // main() has been implemented for you; no need to change anything here ifstream fin; 57 ofstream fout; 58 59 string inFilename = getInput Filename(); string outFilename = deriveOutputFilename(inFilename); 55 56 60 61 62 63 64 if (!openFiles(inFilename, outFilename, fin, fout)) { return EXIT_FAILURE; } 65 66 67 68 69 70 cout .CSV bool validInputFilename (const string &fname) { size_t namelen = fname.length(); // TODO (1): implement validation on the given filename ///look for the presence of INFILE_PREFIX ///look for the presence of IN_FILE_EXT /// ensure the name length is MORE than just the prefix and extension // currently this assumes that all names are valid // but this will fail the unit tests return true; } // Parses a record, placing the substrings into 'name; 'id', and 's1...54 void parseStudentRecord(const string &line, string &name, string &id, int &s1, int &s2, int &s3, int &s4) { // TODO (2): carve up the input line into substrings placing the results into the appropriate reference variables // Returns the smaller of the two values int min(int a, int b) { // TODO (3): return minimum value return 0; } // Returns the smallest of all the values int min(int a, int b, int c, int d) { // TODO (4): return minimum value // HINT: use min(int, int) return 0; } // Returns a letter grade for the given score char gradeFromScore (double score) { char grade = 'F'; // TODO (5): adjust grade based on score return grade; } // Processes a line read from the raw-grades input file void processLine (ostream &out, const string &line) { string studentName, studentId, scores; int score1, score2, score3, score4; int total, lowest; double average; char letterGrade; // TODO (6): process the raw record... parseStudentRecord(line, studentName, studentId, score1, score2, score3, score); // compute total (but subtract the lowest score) 11 - compute the average (of 3 scores) letterGrade = gradeFromScore (average); // TODO (7): write the formatted record to the report file //For the low score field, use: .txt ///use .replace to replace the prefix and the extension return "FIXME=" + in Fname; } // Returns true only if both files opened without error bool openFiles(const string &inName, const string &outName, ifstream &fin, ofstream &fout) { // TODO (10): open input and output files, associate with the given streams // HINT: // open input; if fails, write message and return false open output; if fails, write message and return false // return true return false; } // Read input records, process, write formatted output records void processGradeFile(ifstream &fin, ofstream &fout) { // This function, too, has been implemented for you... nothing to do here string rawline; writeReportHeader(fout); while (getline(fin, rawLine)) { processLine(fout, rawline); } } // Your task for this lab is to complete the implementation of a program that processes a .csv file containing raw grade data, and produces a report file as a .txt. Starter Code The framework of the program has been given to you in the starter code, including useful constants, function prototypes, and the main() function. The parts of the program that you need to complete have been marked with comments: // TODO (n): ... Input File Format The input file will be formatted as follows: Columns, also known as fields, are separated by commas and rows, also known as records, are separated by newlines Note: files formatted in this way can be opened and viewed as a table with a program such as MS Excel or Open Office The first row will be a header for the file containing titles for each field and should be ignored All other rows will be student records consisting of comma-separated fields: o student name o student ID o test scores The test scores will be a comma-separated list of 4 test results For example, the beginning of such a file might look like this: Name, ID, scorel, score2, score3, score4 Fred Flintstone, q5563w, 70,82,55,80 Barney Rubble, q5771x, 65, 62,0,71 You will have to parse, or separate out, each field from a given line of text. Output File Format The output file will be a neatly formatted report showing the student name, their four scores, the lowest score (dropped), the total score, average, and resulting grade letter. For example, given the input above, the report would look like this: Name Scores (Low) Total Average Grade 70 82 55 232 Fred Flintstone Barney Rubble 80 71 (55) 0) 77.33 66.00 65 62 0 198 D Note that various field widths have been defined as constants near the top of the source file. Be sure to use these named constants, rather than hard-coding "magic numbers" when you get to writing the code to output the formatted record. Implementing the helper functions The program is written in a modular way, using functions to "abstract" away details, so that the overall flow of the program is easy to understand. The main() function calls other functions to do the actual work. Your job is to fill in the details by implementing many of these "helper" functions. The Top-Level Functions string getInputFilename() The program starts by asking the user for the name of the input file. To do this it invokes the getInput Filename() function. This function should: Prompt the user to enter a filename. Validate that the filename has the form: o "rawgrades-.CSV" o where identifies the class to which it pertains. ...for example, the above input file might be named "rawgrades-bw101.csv". Continue prompting the user for a filename until a valid one is given. Return the validated filename. Sample run: Enter input filename (rawgrades-.csv): what Enter input filename (rawgrades-.csv): what.csv Enter input filename (rawgrades-.csv): rawgrades.csv Enter input filename (rawgrades-.csv): rawgrades-bw101.csv Processing rawgrades-bw101.csv... Report written to grade-report-bw101.txt string deriveOutputFilename ...) This function takes an input filename as a parameter and should return the corresponding output filename. The format of the output filename is grade-report-.txt bool openFiles(...) This function opens the input and output files, associating the files with the provided input/output file streams. If the input file fails to open the following message should be output before the function returns false: Failed to open for read: If the output file fails to open, the following message should be output before the function returns false: Failed to open for write: The function should return true only if both files opened successfully. Returning false signals to the main program that some error occurred and that the run should be aborted. void processGradeFile (...) This function has been implemented for you. It writes the report header (invoking a function also written for you), then loops over the lines read in from the input file, invoking processLine(...) for each one. Helper Functions To break the problem down into manageable pieces, helper functions have been defined to solve small, specific tasks. These will need to be implemented correctly, and called from the appropriate places to complete the project. You'll find them in the starter code, but as a quick summary: // Returns true if fname is of the form "rawgrades-.csv" bool validInput Filename (const string &fname) // Parses a record (line), placing the substrings into name, id, scores, and sl...54 void parseStudentRecord (const string &line, string &name, string &id, int &sl, int &s2, int &s3, int & S4) // Returns the smaller of the two values int min (int a, int b) // Returns the smallest of all the values int min(int a, int b, int c, int d) // Returns a letter grade for the given score char gradeFromScore (double score) // Processes a line read from the raw-grades input file void processLine (ostream &out, const string &line) // Writes the report header to the given output file stream (ALREADY DONE) void writeReportHeader (ofstream sout) Some notes on parseStudentRecord: Given a line of text, stored in the variable line, you must extract the substrings that are separated by commas. There are a couple of approaches you can take for this: o Method 1: Load the line into a stringstream . Use the overload of getline that takes an optional delimiter. This causes getline to read up to a comma instead of a newline Method 2: Use use . find to find commas, then use .substr to extract substrings from in between those commas Once you have extracted the scores as strings, you will have to convert them to ints. . For this you can use either stoi (string to int) or a stringstream. 295532.1772524 LAB ACTIVITY 7.20.1: Assignment: Grade Processor 0/20 Submission Instructions Downloadable files grader.cpp rawgrades-starwars.csv rawgrades-bw101.csv Download Compile command g++ grader.cpp -Wall -o a.out We will use this command to compile your code Upload your files below by dragging and dropping into the area or choosing a file on your hard drive. grader.cpp Drag file here or Choose on hard drive. AutoSave OFF HESU = 1 rawgrades-bw101 Page Layout Formulas >> Tell me Home Insert Draw Share Comments A. % Conditional Formatting Format as Table Cell Styles Ou Clipboard Font Alignment Number Cells Editing Ideas Se A1 fx Fred Flintstone D E F G H 1 A B 1 Fred Flintstolq5563w 2 Barney Rubb 95771x 3 Yogi Bear 41332b 4 Secret Squirr 49976d 5 Dick Dastard d1101f 6 70 65 89 75 59 82 62 92 77 0 55 0 100 83 37 80 71 95 81 0 7 8 AutoSave OFF BESU = rawgrades-starwars Page Layout Formulas >> Tell me Share Home Insert Draw Comments V A. % Conditional Formatting Format as Table Cell Styles Or Clipboard Font Alignment Number Cells Editing Ideas Save As... Possible Data Loss Some features might be lost if you save this workbook in the co... fx Luke Skywalker A1 D E F G H 1 A B 1 Luke Skywalilred-5 2 Leia Organa d1102 3 Han Solo pirate1 4 Threeplo C3PO 5 Artoo R2D2 6 Darth Vader x451 7 Ben Kenobi obi-wan 8 Jar Jar Binks ep-1 9 10 88 94 87 100 100 95 98 37 97 100 69 100 100 27 93 50 87 88 18 100 99 89 96 3 84 83 83 99 100 67 97 0