Question
Homework Assignment 4 In this homework, you need to design a linked list to keep track of a telephone directory. Eachnode in the list holds
Homework Assignment 4
In this homework, you need to design a linked list to keep track of a telephone directory. Eachnode in the list holds a persons data, such as first name, last name, and phone number. The nodes in the list are sorted by a persons last name. You need to design two classes:
- Class Node has the following private data:
string firstName; string lastName; string phoneNumber;
- Class LL has the following private data: Node * head; // head pointer points to beginning of linked list
Class LL should be made friend of class Node so public functions of LL can access a nodes private attributes without having to write getters and setters for class Node.
You also need to implement the following public methods for class LL:
LL::LL()
The default constructor sets the head to nullptr
void LL::append(string fName, string lName, string phone)
This function creates a node, initializes its data to the values sent as arguments, and inserts it at the end of the list (after the last node)
void LL::insert(string fName, string lName, string phone)
This function creates a node, initializes its data to the values sent as arguments, and inserts it in a way to maintain the linked list order
void LL::insertAtPos(string fName, string lName, string phone, int pos)
This function creates a node, initializes its data to the values sent as arguments, and inserts it at position pos in the list. If pos is 1, insert it as the first node. If pos is 2, insert it as the second node, and so on.
void LL::print()
This function traverses the list printing the data in each node preceded by the node number. For example:
1. Peter Anderson, 352-654-2000
2. Joe Didier, 352-654-1983
...
void LL::printReverse() This function prints the data in each node in reverse order. In other words, it prints the data of the last node first,
followed by the data of the second to last node and so on... There is no need to print the record/node number.
void LL::searchByName(string fName, string lName) This function searches for a particular persons record in the list, whose first name is fName and last name is lName. If it finds a record for this person, it prints the node number or position of that record in the list. The first node in the list is at position 1, the second node at position 2, and so on. If no matching record exists, the function prints an error message
void LL::updateNumber(string fName, string lName, string newPhone)
This function updates a specific person's record. If there exists a person whose first name is fName and last name is lName, then the corresponding phone number should be updated to newPhone. If no such person is found, an error message is displayed
void LL::removeRecord(string fName, string lName) This functions searches for a specific persons record, whose first name is fName and last name is lName, and removes it from the list if it exists
LL::~LL() The destructor destroys (deletes) all nodes in the list and sets head back to null. Have the destructor call another private function destroy that does the deleting. You need to implement that function too:
void LL:destroy()
LL::LL(const LL& source) The copy constructor performs a deep copy of the list named source
LL LL::operator=(const LL& source) This function overloads the assignment operator to perform a deep copy of the list named source. Make sure to free all nodes in list (calling object) before making its head point to a copy of the list source
void LL::reverse() This function rearranges the nodes in the list so that their order is reversed (stored in descending order of last names). It traverses the list and reverses the list nodes so the last node becomes the first and the first node becomes the last one
After designing your classes, you can test your functions using the main program below:
int main() {
LL list1;
LL list2;
cout << ------------------------------------------ << endl;
cout << Adding records to list1 and printing list1 << endl;
list1.append ("Mayssaa", "Najjar", "878-635-1234");
list1.insert ("Jim", "Meyer", "337-465-2345");
list1.insert ("Joe", "Didier", "352-654-1983");
list1.insert ("Adam", "James", "202-872-1010");
list1.insert ("Yara", "Mendiola, "667-277-1454");
list1.insert ("Xavier", "Perez", "352-654-1983");
list1.insertAtPos ("Henry", "Hatch", "617-227-5452", 2);
list1.insertAtPos ("Peter", "Anderson", "352-654-2000", 1);
list1.insertAtPos ("Jamie", "Roberts", "202-832-1560", 9);
list1.insertAtPos("Nancy", "Garcia", "617-227-5454", 3);
list1.insertAtPos("Kevin", "Etheredge", "617-437-5454", 3);
list1.print();
cout << ------------------------------------------ << endl;
cout << Printing list1 backwards << endl;
list1.reversePrint();
cout << ------------------------------------------ << endl;
cout << Searching for specific people in list1 << endl;
list1.searchByName(Nancy, Garcia);
list1.searchByName (Kevin, Etheredge);
list1.searchByName (Jamie, Roberts);
list1.searchByName (Julia, Sarkis);
cout << ------------------------------------------ << endl;
cout << Updating records in list1 and printing list << endl;
list1.updateNumber ("Peter", "Anderson", "989-876-1234");
list1.updateNumber ("Nancy", "Garcia", "345-467-1234");
list1.updateNumber ("Jamie", "Roberts", "202-447-1234");
list1.updateNumber ("Jamie", "Garcia", "345-467-1224");
list1.print();
cout << ------------------------------------------ << endl;
cout << Adding nodes to list2 and printing list 2 << endl;
list2.insert ("Mary", "Mitchell", "617-227-5454");
list2.append ("Adam", "Sage", "202-857-1510");
list2.print();
list2 = list1; LL list3(list1);
list1.removeRecord ("Mayssaa", "Najjar");
list1.removeRecord ("Peter", "Anderson");
list1.removeRecord ("Jamie", "Roberts");
list1.removeRecord ("Jamie", "Najjar");
cout << ------------------------------------------ << endl;
cout << Printing list1 after removing some records << endl;
list1.print();
cout << ------------------------------------------ << endl;
cout << Printing list2 after calling operator= << endl;
list2.print();
cout << ------------------------------------------ << endl;
cout << Printing list3 << endl; list3.print();
cout << ------------------------------------------ << endl;
cout << Printing list3 after reversing it << endl;
list3.reverse(); list3.print();
cout << ------------------------------------------ << endl;
cout << Searching for specific person in list3 << endl;
list3.searchByName (Jamie, Roberts);
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
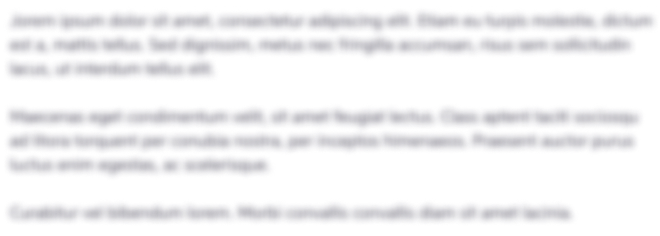
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started