Question
HomeWork Question: In the land of Westeros, Deadpool, Kingkong, and Spiderman had an argument over which one of them was the greatest software developer of
HomeWork Question:
In the land of Westeros, Deadpool, Kingkong, and Spiderman had an argument over which one of them was the greatest software developer of all time. To end the argument once and for all, they agreed on a fight to the death. Deadpool was a poor shooter and only hit his target with a probability of 1/3. Kingkong was a bit better and hit his target with a probability of 1/2. Spiderman was an expert marksman and never missed. A hit means a kill and the person hit drops out of the fight.
To compensate for the inequities in their marksmanship skills, the three decided that they would fire in turns, starting with Deadpool, followed by Kingkong, and then by Spiderman. The cycle would repeat until there was one man or creature standing, and that man or creature would be the greatest software developer of All Time.
An obvious and reasonable strategy is for each man to shoot at the most accurate shooter still alive, on the grounds that this shooter is the deadliest and has the best chance of hitting back.
1
Write a program to simulate the fight using this strategy. Your program should use random numbers and the probabilities given in the problem to determine whether a shooter hits the target. Create a class named Combatant that contains the fighters name and shooting accuracy, a Boolean indicating whether the fighter is still alive, and a method ShootAtTarget(Combatant target) that sets the target to dead if the fighter hits his target (using a random number and the shooting accuracy) and does nothing otherwise.
Once you can simulate a single fight, add a loop to your program that simulates 10,000 fights. Count the number of times that each contestant wins and print the probability of winning for each contestant (e.g., for Deadpool your program might output Deadpool won 3595/10,000 fights or 35.95 %).
A second strategy is for Deadpool to intentionally miss on his first shot. Modify the program to accommodate this new strategy and output the probability of winning for each contestant. So your program will demonstrate both strategies.
What strategy is better for Deadpool, to intentionally miss on the first shot or to try and hit the best shooter? Who has the best chance of winning, the best shooter or the worst shooter?
Note: There are multiple ways to generate Random numbers using the JAVA API.
Following is my code:
package Q2;
public class Combatant {
private Double accuracy;
private String name;
private boolean alive;
public int aliveNumber = 3;
public Combatant(String n, double accur) {
n = name;
accur = accuracy;
alive = true;
}
//create a distinct copy of the object parameter, that has the same values but is in a different memory location
public Combatant(Combatant others)
{
name = others.name;
accuracy = others.getAccuracy();
alive = others.isAlive();
}
public void ShootAtTarget(Combatant target){
double randShoot = Math.random();
if(randShoot < accuracy) {
target.killed();
}
}
public void killed() {
alive = false;
aliveNumber--;
}
public String getName()
{
return name;
}
public double getAccuracy()
{
return accuracy;
}
public boolean isAlive()
{
return alive;
}
public void setName(String name)
{
this.name = name;
}
public void setAccuracy(double accuracy)
{
this.accuracy = accuracy;
}
}
package Q2;
import Q2.Combatant;
public class Strategy1 {
int Dwins =0, Kwins =0, Swins=0;
int BattleNumbers = 10000;
int aliveNumber = 3;
public String startFire() {
Combatant Deadpool = new Combatant("DeadPool", 1/3);
Combatant Kingkon = new Combatant("Kingkon", 0.5);
Combatant Spiderman = new Combatant("Spiderman", 1);
do {
if (Deadpool.isAlive()) {
if (Spiderman.isAlive()) {
Deadpool.ShootAtTarget(Spiderman);;
} else if (Kingkon.isAlive()) {
Deadpool.ShootAtTarget(Kingkon);;
} else;
}
if (Kingkon.isAlive()) {
if (Spiderman.isAlive()) {
Kingkon.ShootAtTarget(Spiderman);
} else if (Deadpool.isAlive()) {
Kingkon.ShootAtTarget(Deadpool);
} else;
}
if (Spiderman.isAlive()) {
if (Kingkon.isAlive()) {
Spiderman.ShootAtTarget(Kingkon);
} else if (Deadpool.isAlive()) {
Spiderman.ShootAtTarget(Deadpool);
} else;
}
} while (aliveNumber > 1);
if (Deadpool.isAlive()) {
return "Deadpool win";
} else if (Kingkon.isAlive()) {
return "Kingkon win";
} else {
return "Spiderman win";
}
}
public int countWinTimes() {
String bestShooter = "";
do {
bestShooter = startFire();
if (bestShooter == "Deadpool win") {
Dwins++;
} else if (bestShooter == "Kingkon win") {
Kwins++;
} else {
Swins++;
}
BattleNumbers--;
} while (BattleNumbers > 0);
return 0;
}
public void finalOutput() {
System.out.println("Deadpool won " + Dwins + " /10,000 times for "+(double)(Dwins)/10000 + "%");
System.out.println("Kingkon won " + Kwins + " /10,000 times for "+(double)(Kwins)/10000+ "%");
System.out.println("Spiderman won " + Swins + "/10,000 times for "+(double)(Swins)/10000+ "%");
}
}
package Q2;
import Q2.Combatant;
import Q2.Strategy1;
public class TestBattle {
public static void main(String[] args) {
System.out.println("In the first strategy:");
Combatant combat = new Combatant();
combat.startFire();
combat.countWinTimes();
combat.finalOutput();
}
}
My Questions are:
1. When I want to create a new Combatant object, error shows the constructor Combatant is undefined. Also, I can not called the functions startFire, countWinTimes and finalOutput.
2. If I want to create a class called strategy2 and make a slightly different from strategy1, how can I do it?
Many thanks!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
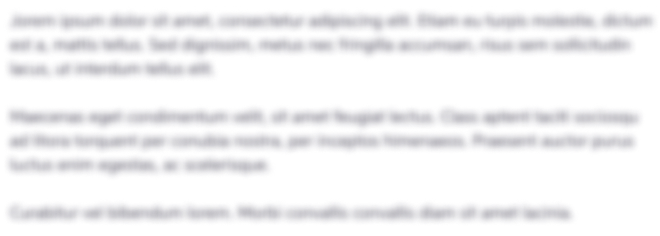
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started