Question
How can I change this using friend operator. Overloads the following operators using friend + replicate the functionality of the add function from matrix.cpp -
How can I change this using friend operator. Overloads the following operators using friend" + replicate the functionality of the add function from matrix.cpp - replicate the functionality of the subtraction function from matrix.cpp * Overload this to replicate the functionality of both the matrix multiplication and scalar multiplication functions If the dimensions of the two matrices involved do not allow for the operation to be performed, skip performing the calculation, and display a message.
#include
using namespace std;
#include
using namespace std;
class Matrix{
private:
int rows, columns;
public:
int **arr; // Creating a 2D array dynamically
bool Check(Matrix a, Matrix b); //Check function
Matrix(int r, int c);
Matrix add(Matrix a, Matrix b);
Matrix subtract(Matrix a, Matrix b);
Matrix multiply(Matrix a, Matrix b);
Matrix scalar(Matrix a, int b);
void printMatrix(Matrix a);
int getRows()const{
return rows;
}
int getColumns()const{
return columns;
}
~Matrix(); //Destructor
};
Matrix::Matrix(int r, int c){
this->rows = r;
this->columns = c;
arr = new int*[this->rows];
for(int i=0; i < this->rows; i++)
arr[i]= new int[this->columns];
}
bool Matrix::Check(Matrix a, Matrix b){
if(a.rows == b.rows && a.columns == b.columns)
return true;
return false;
}
Matrix Matrix:: add(const Matrix a, Matrix b){
Matrix c(a.rows, a.columns);
if(Check(a, b))
{
if((a.rows == b.rows)&&(a.columns == b.columns)){
for(int i =0; i < a.rows; i++){
for (int j =0; j < a.columns; j++){
c.arr[i][j] = a.arr[i][j]+b.arr[i][j];
}
}
}
}return c;
}
Matrix Matrix:: subtract(const Matrix a, Matrix b){ //subtraction
if(a.rows != b.rows || a.columns != b.columns){
cout<<"The subtraction is not possible!"< } Matrix c(a.rows, a.columns); { for(int i =0; i < a.rows; i++){ for (int j =0; j < a.columns; j++) c.arr[i][j] = a.arr[i][j]-b.arr[i][j]; } } return c; } Matrix Matrix::multiply(const Matrix a, Matrix b) { int ar=a.rows; int ac=a.columns; int br=b.rows; int bc=b.columns; if(ac != br) { cout<<"Matrix multiplication is not possible. "; Matrix d(0,0); return d; } Matrix c(ar,bc); //create a new object for (int i=0; i < ar; i++) { for(int j =0; j < bc; j++){ c.arr[i][j] =0; for(int k =0; k c.arr[i][j] += a.arr[i][k] * b.arr[k][j]; } } } return c; } Matrix Matrix::scalar(Matrix a, int s){ //Scalar Matrix c(a.rows, a.columns); for(int i=0; i < c.rows; i++) { for (int j =0; j < c.columns; j++){ c.arr[i][j] = a.arr[i][j]*s; } } return c; } void Matrix::printMatrix(Matrix a){ for (int i =0; i < a.rows; i++){ for (int j = 0; j < a.columns; j++){ cout< } cout< } } Matrix::~Matrix(){ //Free each sub-array //delete arr; //Free the array of pointers this->rows = 0; this->columns = 0; //cout<<"Destroyed"< } int main() { int row1, column1, row2,column2; //Let the user enter the values for first Matrix1 cout<<"Please enter the Rows and Columns in Matrix1."< cin>>row1>>column1; Matrix a(row1, column1); // Creating matrix 1 cout<<"Enter the values of Matrix 1. "< for(int i=0; i < row1; i++){ for(int j=0; j< column1; j++){ cin>>a.arr[i][j]; } } //Let the user enter the values for first Matrix2 cout<<"Please enter the Rows and Columns in Matrix2."< cin>>row2>>column2; Matrix b(row2, column2); // Creating matrix 2 cout<<"Enter the values of Matrix 2. "< for(int i=0; i < row2; i++){ //Let the user to enter the values for(int j=0; j< column2; j++){ cin>>b.arr[i][j]; } } // Print Martix 1 cout<<" Matrix 1 "< a.printMatrix(a); // Print Martix 2 cout<<" Matrix 2 "< b.printMatrix(b); cout<<" The addition of 1 and 2 is "< if(a.Check(a, b)) { Matrix c = a.add(a,b); c.printMatrix(c); } else cout<<"This cannot be add!"< cout<<" The subtraction of 1 and 2 is "< if(a.Check(a, b)){ Matrix d = a.subtract(a,b); d.printMatrix(d); } else cout<<"This cannot be subtract!"< cout<<" The multiplication of 1 and 2 is "< Matrix e = a.multiply(a,b); e.printMatrix(e); cout<<" The scalar multiplication of 1 and 2 of 3 is "< Matrix f = a.scalar(a,3); f.printMatrix(f); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
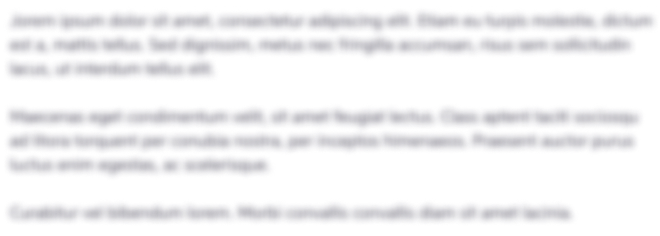
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started