Question
How can I complete my code (stock_market.py) ? Instruction getStock() function The code includes a function getStock(). It takes (as aparameter) the symbol for a
How can I complete my code (stock_market.py) ?
Instruction
getStock() function
The code includes a function getStock(). It takes (as aparameter) the symbol for a stock, and calls requests.get() with arequest for prices of the specified stock from the AlphaVantageAPI. This function is complete except it needs an API key. Copyyour AlphaVantage API key into the getStock() function whereindicated. (Note: Your key must be inside the quotes.) Other thanadding your API key, do not edit the getStock()function.
The stock data returned from Alpha Vantage's Web-API includes alot of data you don?t need. The only data you need for this stockapp is in the text part, so getStock() extracts that. If a stockwith the specified symbol exists, the text part of the data is twolines of text like that shown below for MSFT, the symbol forMicrosoft stock:
symbol,open,high,low,price,volume,latestDay,previousClose,change,changePercentMSFT,107.7900,108.6600,107.7800,108.4100,10957711,2019-02-19,108.2200,0.1900,0.1756%
The first line is a comma-separated list of the names of all thepieces of data in the results. The second line is a comma-separatedlist of the data corresponding to each name. You can think of thisas a table with ten columns, a row of headings, and a row of data,e.g.,
symbol | open | high | low | price | volume | latest Day | previousClose | change | changePercent |
MSFT | 107.7900 | 108.6600 | 107.7800 | 108.2200 | 10957711 | 2019-02-19 | 108.2200 | 0.1900 | 0,1756 |
In this data, ?open? is today?s opening price of the stock,?high? is today?s highest price, ?low? is today?s lowest price,?price? is the current price, and ?previousClose? is yesterday?sclosing price.
main() function
The starter code includes a main() function that is called whenthe app starts. Add code to make it:
1. Start by displaying this message: ?Check stock prices.?
2. Ask the user for a stock symbol.
3. Call getStock() with the stock symbol, and save the returnedstock-price data.
4. Split the data (two text lines) into a list of two strings(hint: use string method .split()).
5. If the split data is a list of two strings (each in CSVformat), the data is good and the stock was found. (Hint: if thestock symbol was valid, the length of the split data should be 2.)Extract the stock?s previous closing price, opening price, highprice, low price, Prof Jeff Johnson CS 110 and current price. Do itin a way that will still work if AlphaVantage changes the order ofitems in the returned data. E.g., don?t assume that the stock?sopening price is in the second position (index 1) in the list,because if your program assumes that and tomorrow AlphaVantagemoves the opening price to another position, your app won?t workanymore. Write your app so it finds each price no matter how theyare ordered, and uses the appropriate index to get each price.
6. Print the previous closing price, opening price, high price,current price, and low price.
7. If the split data is not a list of exactly 2 items (CSVstrings), that means the stock symbol was not found. In that case,tell the user the stock symbol was not found.
8. When the app displays prices for a stock (but not when itcan?t find a stock), it should also write the same information toan output file stock_prices.txt. Each time the app successfullydisplays the prices for a stock, the app should append theinformation to the file. E.g., if the user requests prices forthree stocks, when the application quits, stock_prices.txt shouldcontain info for those three stocks, with a line like thisseparating them: ======================
9. The app should not clear and replace the data instock_prices.txt each time it is run. It should keep appendingstock prices to the file.
10. The program should ask the user ?Check another stock price?(Y or N)?, and should perform input validation to make users entera valid response. If the response is ?Y? or ?y?, the program shouldloop back to Step 2 (not Step 1) and ask for another stock symbol.If the response is ?N? or ?n?, the program closes the output fileand quits.
Example of program?s screen output
Check stock prices.
Enter stock symbol: IBM
Stock Symbol: IBM
Last Close: 133.2600
Open: 133.7600
High: 133.9300
Low: 132.2700
Current: 133.2300
Check another stock price? (Y or N): y
Enter stock symbol: aapl
Stock Symbol: AAPL
Last Close: 122.1500
Open: 123.6600
High: 124.1800
Low: 122.4900
Current: 123.0000
Check another stock price? (Y or N): x
Please enter Y or N.
Check another stock price? (Y or N): n
Example of program?s file output
Stock Symbol:IBM
Last Close: 133.2600
Open: 133.7600
High: 133.9300
Low: 132.2700
Current: 133.2300
====================
Stock Symbol:AAPL
Last Close: 122.1500
Open: 123.6600
High: 124.1800
Low: 122.4900
Current: 123.0000
====================
My current output :
Check stock prices.
Enter stock symbol:AMZN
Stock Symbol: AMZN
Traceback (most recent call last):
File "/Users/alice/Desktop/CS50/stock_market.py", line46, in
main()
File "/Users/alice/Desktop/CS50/stock_market.py", line24, in main
getStock(symbol)
NameError: name 'symbol' is not defined
TA's advice:
"It looks like you saved the stock symbol into the variable namestring and then tried to call getStock on the variable symbol,which doesn?t contain anything. If you call it on string instead,it should get you past that error. As for the price part, youshould be extracting all of the price information from the list oftwo strings that you already have. You?re saving multiple prices(opening price, closing price, etc.) so take that intoconsideration."
My current code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41234 45 46 F 47 stock_market.py # Web App to Get Stock Market Data from AlphaVantage Service import requests #Function that gets stock info from API # Returns results in list of two strings # DO NOT EDIT THIS FUNCTION EXCEPT TO INSERT YOUR API KEY WHERE INDICATED def getStock(symbol): baseURL = "https://www.alphavantage.co/query? function=GLOBAL_QUOTE&datatype=csv" keyPart "&apikey=" + "2QPEAS3W0V7W3U0Y" #Add API key symbolPart "&symbol=" + symbol stockResponse requests.get(baseURL+keyPart+symbolPart) return stockResponse.text #Return only text part of response #Function that computes and displays results def main(): # Add code that meets the program specifications (see instructions in Canvas) flag = True while(True): if (flag==False): break main() print('Check stock prices.') string=input("Enter stock symbol:") print("Stock Symbol:", string) getStock(symbol) priceLabel print (stockdata.split()) list of prices "Stock Symbol, lastClose, Open, High, Low, Current" datastringlist[0].split(',') print (prices.split()) print (priceLabel, prices) result = open("stock_prices.txt","w") result.write(priceLabel+str(prices)) result.close() while(True): user_answer = input("Check another stock? (Y or N):") if(user_answer == 'Y'): break elif(user_answer == 'N'): .ag = False break #Code that starts the app
Step by Step Solution
3.49 Rating (159 Votes )
There are 3 Steps involved in it
Step: 1
import requests Function to get stock data from AlphaVantage API def getStocksymbol url httpswwwalphavantagecoquery params function GLOBALQUOTE symbol ...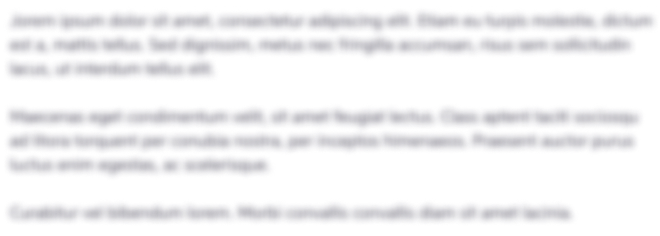
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started