Answered step by step
Verified Expert Solution
Question
1 Approved Answer
How can I make the delete function work properly where it deletes a node based on its ID #include #include using namespace std; struct Node
How can I make the delete function work properly where it deletes a node based on its ID
#include
#include
using namespace std;
struct Node
int id;
string name;
float gpa;
Node next;
;
Node createNodeint id const string& name, float gpa
Node newNode new Node;
newNodeid id;
newNodename name;
newNodegpa gpa;
newNodenext nullptr;
return newNode;
void addNodeNode& head, int id const string& name, float gpa
Node newNode createNodeid name, gpa;
if head nullptr
head newNode;
return;
if headid id
newNodenext head;
head newNode;
return;
Node current head;
while currentnext nullptr && currentnextid id
current currentnext;
if currentnext nullptr && currentnextid id
cout "Duplicate ID found. Please enter other ID endl;
delete newNode;
return;
newNodenext currentnext;
currentnext newNode;
void deleteNodeNode& head, int id
if head nullptr
cout "List is empty. Cannot delete." endl;
return;
if headid id
Node temp head;
head headnext;
delete temp;
return;
Node current head;
while currentnext nullptr && currentnextid id
current currentnext;
if currentnext nullptr
cout ID not found. Cannot delete." endl;
return;
Node temp currentnext;
currentnext currentnextnext;
delete temp;
void displayListconst Node head
if head nullptr
cout "List is empty." endl;
return;
const Node current head;
while current nullptr
cout ID: currentid Name: currentname GPA: currentgpa endl;
current currentnext;
Other functions like Modify, Purge, Search can be implemented similarly
int main
Node head nullptr;
bool created false;
while true
cout "Enter command" endl;
cout Create
Add
Delete
Display
Modify
Purge entire list
Search for node
Exit the program" endl;
string command;
cin command;
if command
created true;
cout "Linked list created." endl;
else if command
if created
cout "Create Linked list first." endl;
continue;
int id;
string name;
float gpa;
cout "Enter ID: ;
cin id;
cout "Enter Name: ;
cin name;
cout "Enter GPA: ;
cin gpa;
cout "Node added." endl;
addNodehead id name, gpa;
else if command
if created
cout "Create Linked list first." endl;
continue;
Implement delete functionality
else if command
if created
cout "Create Linked list first." endl;
continue;
displayListhead;
else if command
if created
cout "Create Linked list first." endl;
continue;
Implement modify functionality
else if command
if created
cout "Create Linked list first." endl;
continue;
Implement purge functionality
else if command
if created
cout "Create Linked list first." endl;
continue;
Implement search functionality
else if command
break;
else
cout "Invalid command. Please try again." endl;
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
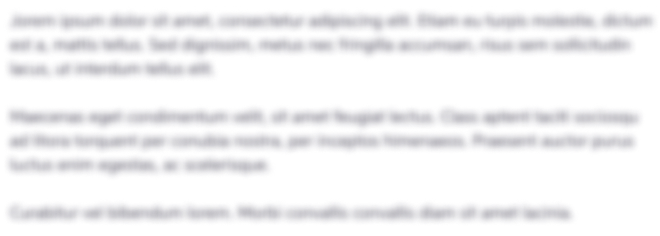
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started