Question
How do I add the following polymorphic functionality to my already existing code: Develop a polymorphic banking program using the Bank-Account hierarchy. For each account
How do I add the following polymorphic functionality to my already existing code:
Develop a polymorphic banking program using the Bank-Account hierarchy. For each account in the vector, allow the user to specify an amount of money to withdraw from the Bank-Account using member function debit and an amount of money to deposit into the Bank-Account using member function credit. As you process each Bank-Account, determine its type. If a Bank-Account is a Savings, calculate the amount of interest owed to the Bank-Account using member function calculateInterest, then add the interest to the account balance using member function credit. After processing an account, print the updated account balance obtained by invoking base-class member function getBalance.
Here is the already existing code:
#include "stdafx.h" #include
using namespace std;
class BankAccount { private: double balance; public: BankAccount(double bal) { if (bal < 0) { balance = 0.0; cout << "Error: The initial balance was invalid. "; } else { balance = bal; } } double getBalance() { return balance; } void credit(double d) { balance = balance + d; } bool debit(double d) { if (d <= balance) { balance = balance - d; return true; } else { cout << "The balance is less than the debit amount. "; return false; } } };
class Savings : public BankAccount { private: double interest; public: Savings(double bal, double inter) : BankAccount(bal) { interest = inter; } double calculateInterest() { return (interest / 100) * getBalance(); } };
class Checking : public BankAccount { private: double trans_fee; public: Checking(double bal, double fee) : BankAccount(bal) { trans_fee = fee; } void debit(double d) { if (BankAccount::debit(d)) { BankAccount::debit(trans_fee); } } void credit(double d) { BankAccount::credit(d); BankAccount::debit(trans_fee); } };
int main() {
BankAccount a(700); Savings s(800, 10); Checking c(900, 1);
cout << "Bank Account: " << a.getBalance() << endl; cout << "Savings Account: " << s.getBalance() << endl; cout << "Checking Account: " << c.getBalance() << endl; cout << endl;
a.debit(100); s.debit(200); c.debit(300); cout << "Bank Account -100: " << a.getBalance() << endl; cout << "Savings Account -200: " << s.getBalance() << endl; cout << "Checking Account -300 and transaction fee: " << c.getBalance() << endl; cout << endl;
a.credit(400); s.credit(500); c.credit(600); cout << "Bank Account +400: " << a.getBalance() << endl; cout << "Savings Account +500: " << s.getBalance() << endl; cout << "Checking Account +600 and another transaction fee: " << c.getBalance() << endl; cout << endl;
double d = s.calculateInterest(); s.credit(d); cout << "Savings Account after .01% interest: " << s.getBalance() << endl; cout << endl; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
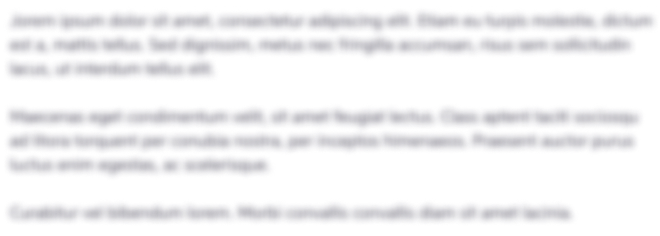
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started