Question
How do I fix the compiler errors at the end of the main function and in the insert function? Here are requirements: Store the file
How do I fix the compiler errors at the end of the main function and in the "insert" function?
Here are requirements:
Store the file contents in a linked list. o Add the node to the beginning of the list All manipulation of the data will happen in the linked list. o Convert each piece of data to its unlisted counterpart Write the contents of the linked list back to the file at the end of the program. The file may contain invalid data. o If invalid data is identified, ignore the entry in the file. Invalid data: o Arabic numeral outside the given rage. o Invalid characters in Roman numeral. o Invalid characters in Arabic numeral. User will be able to search and sort the data. Use a linear search o Search can be for Arabic or Roman numeral o Do not ask user what type of numeral to search for (-5 points if user is asked) User can type in Arabic or Roman numeral You have to figure out what they typed Implement sorting algorithm of your choice o Sorting algorithm will move nodes (-10 points) o Do not exchange node data o All sorts will sort in ascending order Arabic: smallest to largest number Roman: alphabetical
#include #include #include #include #include #include #include #include
using namespace std;
struct Node { int data; struct Node *next; }*head;
// Number struct struct Number { char roman[16]; char arabic[5]; };
// function prototypes typedef Number* NumberPtr; int convertRomanToArabic(string); string convertArabicToRoman(int); void sort(); void linearSearch(struct Number, int); void insertFirstElement(NumberPtr&, char, char); void insert(NumberPtr&, char, char);
// main function int main() { // open the file to read ifstream inFile; ofstream outFile; inFile.open("numbers.txt", ios::in);
// exit from the program if the input file does not exist if (inFile.fail()) { cout << "Input file could not be opened." << endl; exit(1); }
//Declared variables list lst; Number num; string str; int si; //Space index int count = 0; int choice;
inFile.seekg(0); //Go to first line
while (!inFile.eof())//While not end of file { num.roman[15] = '\0'; num.arabic[4] = '\0';
inFile.read((char *)&num, sizeof(Number)); //Read characters from input file if (num.roman[0] == ' ') { str = num.arabic; si = str.find(' '); str = str.substr(0, si);
string rom = convertArabicToRoman(atoi(str.c_str())); //Call to arabic-to-roman conversion function
strcpy(num.roman, rom.c_str()); }
else { str = num.roman; si = str.find(' '); str = str.substr(0, si);
for(int i=0;i num.roman[i] = str[i]; } for(int i=si;i<15;i++){ num.roman[i] ='\0'; }
int arab = convertRomanToArabic(str);//Call to roman-to-arabic conversion function
stringstream ss; ss << arab; string s = ss.str();
strcpy(num.arabic, s.c_str()); cout< }
lst.push_back(num);
count++;
}
//Close file inFile.close();
//Open file to write to outFile.open("output.txt", ios::out);
//Write data to file list::iterator itr = lst.begin(); while (itr != lst.end()) { outFile << left << setw(15) << (*itr).roman << " " << setw(4) << (*itr).arabic;//Write to output file itr++;
if(itr != lst.end()) outFile << endl; }
//Close file outFile.close();
//Output options menu cout<<"1. Search"< cout<<"2. Sort"< cout<<"3. Exit"< cin >> choice;//Get selection from user
if (choice == 1) { linearSearch(num,count);//Call to search function } else if (choice == 2) { sort();//Call to sort function } else if (choice == 3) { return 0; //Return 0 } else { cout << "Invalid option" << endl; //If invalid option is entered }
char rom; char arab;
NumberPtr head = new Number;
//Write numbers to linked list while (inFile >> num.roman >> num.arabic) { cout << num.roman << endl; cout << num.arabic << endl; insertFirstElement (Number*, rom, arab); }
return 0;
}
//function that converts roman numerals to arabic numbers int convertRomanToArabic(string str) { int arabic = 0; char ch; //Current character char nch; //Next character
if (str.length() == 0) { return 0; }
for (unsigned int i = 0; i < str.length() - 1; i++) { ch = str[i]; nch = str[i + 1];
//Arabic conversions if (ch == 'M') arabic += 1000; else if (ch == 'D') arabic += 500; else if (ch == 'C' && (nch == 'D' || nch == 'M')) arabic -= 100; else if (ch == 'C') arabic += 100; else if (ch == 'L') arabic += 50; else if (ch == 'X' && (nch == 'L' || nch == 'C')) arabic -= 10; else if (ch == 'X') arabic += 10; else if (ch == 'V') arabic += 5; else if (ch == 'I' && (nch == 'V' || nch == 'X')) arabic -= 1; else if (ch == 'I') arabic += 1; else { cout << "Invalid roman numeral." << ch << endl; exit(1); } }
ch = str[str.length() - 1];
//Arabic conversions if (ch == 'M') arabic += 1000; else if (ch == 'D') arabic += 500; else if (ch == 'C') arabic += 100; else if (ch == 'L') arabic += 50; else if (ch == 'X') arabic += 10; else if (ch == 'V') arabic += 5; else if (ch == 'I') arabic += 1; else { cout << "Invalid roman numeral." << ch << endl; exit(1); }
return arabic; }
//function that coverts arabic numbers to roman numerals string convertArabicToRoman(int arabic) { string roman; int curr;
//Roman conversoins if (arabic >= 5000) { curr = arabic / 1000;
for (int i = 0; i < curr; i++) { roman += 'M'; }
arabic = arabic % 1000; }
//Roman conversions if (arabic >= 100) { curr = arabic / 100;
if (curr == 9) { roman += "CM"; } else if (curr >= 5) { roman += 'D';
for (int i = 0; i < curr - 5; i++) { roman += 'C'; } } else if (curr == 4) { roman += "CD"; } else if (curr >= 1) { for (int i = 0; i < curr; i++) { roman += 'C'; } }
arabic = arabic % 100; }
//Roman conversions if (arabic >= 10) { curr = arabic / 10; if (curr == 9) { roman += "XC"; } else if (curr >= 5) { roman += 'L'; for (int i = 0; i < curr - 5; i++) { roman += 'X'; } } else if (curr == 4) { roman += "XL"; } else if (curr >= 1) { for (int i = 0; i < curr; i++) { roman += 'X'; } }
arabic = arabic % 10; }
if (arabic >= 1) { curr = arabic; if (curr == 9) { roman += "IX"; } else if (curr >= 5) { roman += 'V'; for (int i = 0; i < curr - 5; i++) { roman += 'I'; } } else if (curr == 4) { roman += "IV"; } else if (curr >= 1) { for (int i = 0; i < curr; i++) { roman += 'I'; } } }
return roman; }
//Function to insert first element void insertFirstElement(int number, struct Node **head)
{
struct Node* newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->data = number;
newNode->next = NULL;
if(*head == NULL)
{
*head = newNode;
}
else
{
struct Node *current = *head;
while(current->next != NULL)
{
current = current->next;
}
current->next = newNode;
}
}
void insert(Node **head)
{
if(head == NULL)
return;
struct Node* temp = (struct Node*) malloc(sizeof(struct Node));
*temp = *head;
*head = temp->next;
delete temp;
}
//Sort function void sort() { struct Node *ptr, *s, *prev;
if (head == NULL) { cout << "List is empty" << endl; return; } ptr = head;
while ( ptr && ptr->next ) //Check preset and next { if ( ptr->data > ptr->next->data ) //Check if next element is greater than or not { if ( prev == NULL ) //For first condition, when head needs to change { s = ptr->next; //Save next ptr->next = ptr->next->next; //Connect next to next next s->next = ptr; //Connect previous head = s; //Change the head ptr = head; //Reset the ptr } else if ( prev && ptr->next->next ) //If swap is not for first element { s = ptr->next; ptr->next = ptr->next->next; s->next = ptr; prev->next = s; ptr = head; prev = NULL; //Reset previous } else if ( prev && ptr->next ) //Condition for last elements { s = ptr->next; ptr->next = NULL; //Last element connect to null s->next = ptr; prev->next = s; ptr = head; prev = NULL; } } else //Executes first elements need not to be swap { prev = ptr; ptr = ptr->next; } } }
//Function for linear search void linearSearch(Number num, int size) { //Declared and initialized variables int value, position = 0;
cout << "Enter the value to be searched: "; cin >> value; //Value entered by user struct Node *s; s = head;
while (s != NULL) //While head is not null { position++;//Increment position
if (s->data == value) { cout<<"Element "<
Step by Step Solution
There are 3 Steps involved in it
Step: 1
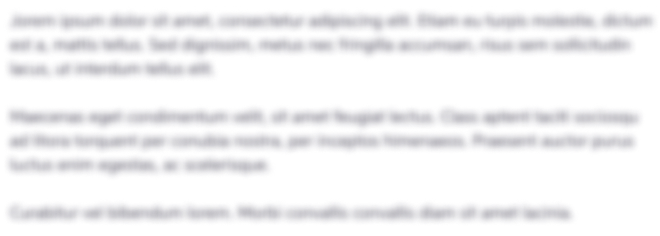
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started