Question
How do you code this. private intgetSumDiceValues () - return the sum of the values of the three dice.Use a loop to navigate/iterate the array
How do you code this.
private intgetSumDiceValues () - return the sum of the values of the three dice.Use a loop to navigate/iterate the array of GVdie objects
private boolean isDoubles (int num) - return true if two of the dice values match num, return false otherwise. Use a loop to navigate the array of GVdie objects
private boolean isTriplets ( ) - return true if all three values of the dice are identical, return false otherwise.
private void checkLarge( ) - invoke the getSumDiceValues() method to get the sum of the values of the three dice, check if the sum is greater than 10 and there is no three-of-a-kind (triplet). If so, increase the credits by 2 and update the message to indicate that the player won.Note, invoke the isTriplets()method when appropriate.
private void checkSmall( ) - invoke the getSumDiceValues() method to get the sum of the values of the three dice, check if the sum is less than 11 and there is no three-of-a-kind (triplet).If so, increase the credits by 2 and update the message to indicate that the player won.Note, invoke the private isTriplets() method when appropriate.
private void checkField( ) - invoke the getSumDiceValues() method to get the sum of the values of the three dice, check if the dice total is less than 8 or greater than 12.If so, increase the credits by 2 and update the message to indicate that the player won.
private void checkNumber(int num) - Check how many of the dice values match num.If all three dice match, increase the credits by 11.If only two dice match, increase the credits by three.If only one die matches, increase credits by 2.Invoke the isTriplets and isDoubles methods to keep this method as short as possible.For all wins, update the message to indicate that the player won.
private void checkAllBets ( ) - Initially set the message string to indicate that the player lost.The message will get reset in the helper methods if the player wins.Use a loop to navigate the array of bets.For each bet that was placed, decrease the number of credits by 1. This method invokes the appropriate helper methods to check if the bet was won. For example, if there is a bet placed associated with index 6, you need to invoke the checkField method, if there is a bet associated with the index number between 0 and 5 (inclusive) you need to invoke the checkNumber method.Note: pay attention to the input parameter that you need to pass when invoking the checkNumber method, remember that the array of bets starts at index 1.
Phase 3 (56 points) Due 11/6/2020
Step 1 Continued: The Chuck Class Mutator Methods( 18 point -- 3 points each)
public void addCredits (int amount) - add amount to the credits.Ignore the request if amount is negative.
public void placeBet (int bet) - Set the appropriate element of the array of bets to true based on the bet parameter.The valid values of the bet parameters are between 1 and 9 (inclusive).Remember that the index of the array starts at 0.
Example:A visual for an array of bets where there are bets placed for 1's, 2's and large
An Array of ten Boolean values for making nine bets, where bet[ 0] is unused
bet1s2s3s4s5s6sFieldSmallLarge
Booleanvalue
true
true
false
false
false
false
false
false
true
Index0123456789
public void cancelBet (int bet) - Set the appropriate element of the array of bets to false based on the bet parameter.The valid values of choice are between 1 and 9 (inclusive).Remember that the index of the array starts at 0.
public void clearAllBets( ) - Use a loop to set all the elements of the bets array to false.
public void roll ( ) -
oUse a loop to navigate the array of GVdie objects and roll the three dice.
oInvoke the checkAllBets() method to check each of the bets to determine which bets, if any, the player won.
oInvoke the clearAllBets()method to clear all bets to prepare for the next round.
public void reset () - reset for a fresh game.
oUse a loop to set all dice to blank.
oSet the message and credits to the appropriate values
oInvoke the clearAllBets( ) method to clear all bets
Preventing Player Errors ( 6 points - 3 points each)
The game is now functional but it is possible the player will make various errors.Make the following improvements to prevent common errors.
public boolean enoughCredits ( ) - return true if the player has enough credits to cover all bets, return false otherwise.Use a loop to navigate the array of bets and count the number of active bets and compare it with available credits.
Modify the roll() method to only roll the dice if there are enough credits to cover all current bets.Invoke the enoughCredits () when appropriate. Otherwise, update the message to inform the player that there are not enough credits to play and set the dice to blank.
Step 2: Software Testing (6 points)
Games and other applications that generate random results are difficult to test.The following methods are provided within the Chuck class to help during testing.It would normally be removed after testing is complete but you will keep it in your solution. These two methods will be invoked in the JUnit class provided to do the automatic testing.
public String diceToString ( ) - return (don't print) a formatted String of the array of GVdie objects.You can use this method in your test class to be able to see the values of your dice.
use a loop to navigate the array of dice
inside of the loop get the value of each dice and add it to the String that is being returned. Use a comma to separate the values of the dice.
Example:If the values of the dice are:
The method will return the String: [1,2,5]
public void testRoll(int [] values) - this method will roll the dice until the desired values entered as the array of 3 integer values have been rolled.This makes the testing a lot easier because you can control what numbers are rolled.
oIf there are enough credits to play, this method has to do the following:
If a requested value is not between 1 and 6 then set it to one.
Repeatedly roll each die in the array of dice until the desired value is obtained.
Notes:
This logic includes a nested loop.
Do not make any changes to the GVdie class. The GVdie class does not have a setValue method to avoid cheating.
Example:
If the array entered as input parameter is {3,1,5}, this method will roll the die in position zero until its value is 3, etc.
Now that the dice have the wanted values
-invoke the checkAllBets() method to check each of the bets to determine which bets, if any, the player won.
-invoke the clearAllBets()method to clear all bets to prepare for the next round.
Update ChuckTest - update the main method (10 points)
Update the main method in the ChuckTest. Instantiate a game and invoke the methods with a variety of parameter values to test each method.It takes careful consideration to anticipate and to test every possibility. This is an incomplete example.Your solution will be longer.
public static void main(String [] args){
int before = 0;
final int ONES = 1;
final int TWOS = 2;
final int THREES = 3;
final int FOURS = 4;
final int FIVES = 5;
final int SIXES = 6;
final int FIELD = 7;
final int SMALL = 8;
final int LARGE = 9;
Chuck game = new Chuck();
System.out.println("Testing begins...");
assert game.getCredits() == 10 : "credits should start at 10";
// wins bet on Large
before = game.getCredits();
game.placeBet(LARGE);
game.testRoll(new int [] {6,3,3});
assert game.getCredits() == before + 1 : "should have won betting on Large";
// loses bet on Large
before = game.getCredits();
game.placeBet(LARGE);
game.testRoll(new int [] {2,3,3});
assert game.getCredits() == before - 1 : "should have lost betting on Large";
System.out.println("Testing completed.");
}
JUnit Testing (16 points)
JUnit is a Java library that helps to automate software testing. A JUnit test file has been provided on Blackboard. Follow these instructions to use the test file.
1.Name your class Chuck. Exact spelling is required in the Chuck class for the class name and all the method headers.
2.Completethe requirements described above or some of the unit tests will fail.
3.Download JUnitChuck.javafrom Blackboard to the same folder as your Chuck.java
Restart BlueJ (if JUnitChuck does not show up on your BlueJ window). BlueJ should recognize JUnitChuck as a "<
4.On the BlueJ window, click the "Run Tests" button.
Example:JUnit test passes all the test cases
If your class passes all the test cases, the test results window will show a green horizontal bar and zero failures.
Example: JUnit test does not pass all the test cases:
If your class does NOT pass all the test cases, you will see a red horizontal bar and the number of failures. Click on a test result to show a longer description of the failure.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
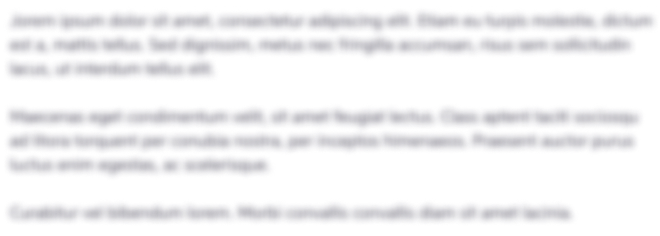
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started