Question
How do you make a head insert function with this type of linked list? #include #include #include typedef struct node { int data; struct node
How do you make a head insert function with this type of linked list?
#include
#include
#include
typedef struct node
{
int data;
struct node *next;
} node;
// A neat struct to hold the 'head' and 'tail' pointer of a linked list. This
// provides a really elegant mechanism for functions to be able to modify both
// the head and tail of a linked list without need for return values or double
// pointer parameters.
typedef struct LinkedList
{
node *head;
node *tail;
} LinkedList;
// Create a linked list. Initialize those pointers to NULL with some calloc()
// fanciness.
LinkedList *create_list(void)
{
LinkedList *new_list = malloc(sizeof(LinkedList));
new_list->head = NULL;
new_list->tail = NULL;
return new_list;
}
// Create a new node. Properly initialize all fields.
node *create_node(int data)
{
node *new_node = malloc(sizeof(node));
new_node->data = data;
new_node->next = NULL;
return new_node;
}
// A function for inserting at end of a linked list. (The LinkedList struct
// maintains pointers to both the head and tail of the list.)
void tail_insert(LinkedList *list, int data)
{
// Just in case...
if (list == NULL)
{
return;
}
// If we have an empty list, create a new node (now the only node in the
// list), and set both our head and tail pointers to that single node.
else if (list->tail == NULL)
{
list->head = list->tail = create_node(data);
}
// Otherwise, append the new node to the end of the list.
else
{
list->tail->next = create_node(data);
list->tail = list->tail->next;
}
}
void head_insert(LinkedList *list, int data)
{
}
// Print the contents of the linked list.
void print_list(node *head)
{
if (head == NULL)
return;
printf("%d%c", head->data, (head->next == NULL) ? ' ' : ' ');
print_list(head->next);
}
// Takes the head of a linked list and frees all the nodes in that list. Returns
// NULL.
node *destroy_list(node *head)
{
if (head == NULL)
return NULL;
destroy_list(head->next);
free(head);
return NULL;
}
// Takes a LinkedList struct and frees all the dynamically allocated memory
// associated with that struct -- the linked list inside it, as well as the
// struct itself.
LinkedList *destroy_linked_list(LinkedList *list)
{
if (list == NULL)
return NULL;
// Free the entire list within this struct.
destroy_list(list->head);
// Free the struct itself.
free(list);
return NULL;
}
int main(void)
{
LinkedList *list = create_list();
int i, r;
srand((unsigned int)time(NULL));
for (i = 0; i < 5; i++)
{
printf("Inserting %d... ", r = rand() % 100 + 1);
tail_insert(list, r);
}
print_list(list->head);
list = destroy_linked_list(list);
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
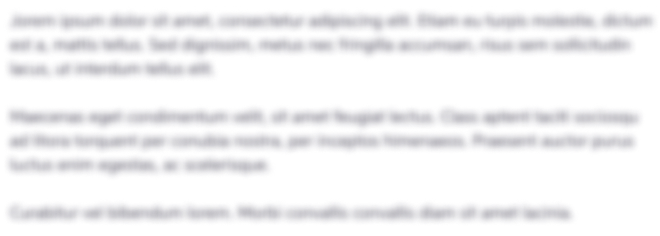
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started