Question
How does exception handling work in Java? What are exception handling keywords in Java? How can you catch multiple exceptions? What is the difference between
- How does exception handling work in Java?
- What are exception handling keywords in Java?
- How can you catch multiple exceptions?
- What is the difference between throw and throws keyword in Java?
- Explain the Finally keyword in Java.
Practical:
- Write Java Program code to solve dividebyzero error with exception handling.
- Write program to illustrate the concept of multiple catch statements.
- Write sample code using Finally keyword in Java.
Correct the code exercises:
1. Add the missing code and generate the correct output.
________{
int[] myNumbers = {1, 2, 3};
System.out.println(myNumbers[10]);
} _________ (Exception e) {
System.out.println("Something went wrong.");
}
2. Add the missing code to the program below in order to generate the given output.
public class MyClass {
public static void main(String[] args) {
_______
int[] myNumbers = {1, 2, 3};
System.out.println(myNumbers[10]);
} catch (________) {
System._________._______ ("_________.");
}
}
}
Output: Something went wrong.
3.Add the missing code to the program in order to generate the given output.
public class MyClass {
public static void main(String[] args) {
_________{
int[] myNumbers = {1, 2, 3};
System.out.println(myNumbers[10]);
} __________{
System.out.println("____________");
} ________{
System.out.println("_____________");
}
}
}
Output:
Something went wrong.
The 'try catch' is finished.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Heres a comprehensive response covering all aspects of exception handling in Java Exception Handling in Java How it works When an error occurs an exce...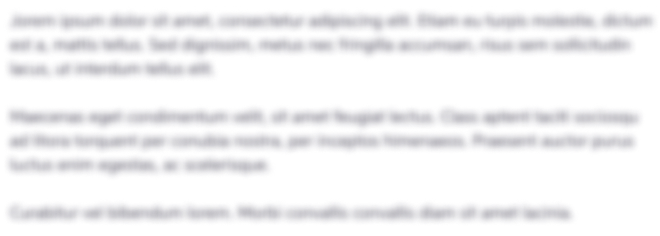
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started