Answered step by step
Verified Expert Solution
Question
1 Approved Answer
How to make GUI for this program? import java.util.Scanner; /********************************************************************************************************************************************* * *description: ContactManagerDemo is a program with a phone book menu where user can add
How to make GUI for this program?
import java.util.Scanner; /********************************************************************************************************************************************* * *description: ContactManagerDemo is a program with a phone book menu where user can add entry, modify entry, delete entry, view all entries, *save book, and load book. *input: user inputs the menu options *output: Successfully completed the action based on the selected menu option by user. * Add user contact details to the address book * Modify the existing contact details by selecting name * Delete the existing contact details * View all contact details * load all contact details * save contact details * exit from the address book * @author Hitesh Kathi * @version 1.0 * @Date 06/04/2022 ***********************************************************************************************************************************************/ public class ContactManagerDemo { /******************************************************************************************************************************** * This is the main method which is responsible for running the program. * @param args command line arguments * *************************************************************************************************************************************/ public static void main(String[] args) { // a Scanner object for user input for menu choices Scanner scanner = new Scanner(System.in); ContactManager manager = new ContactManager(); int choice; // integer variable for recording user choice of the menu // a do-while loop for the menu to repeat do { displayMenu(); // display the menu choice = Integer.parseInt(scanner.nextLine().trim()); // take user input for the menu option switch (choice) { // implement a switch case for the option selected by the user case 1: { // if user chooses option 1 System.out.println(" ADD CONTACT: ------------"); // heading for the option manager.addContact(); // call the addContact() method of the ContactManager class System.out.println(); // an extra newline for proper output visibility break; } case 2 : { // if user chooses option 2 System.out.println(" MODIFY CONTACT: ---------------"); // heading for the option manager.modifyContact(); // call the modifyContact() method of the ContactManager class System.out.println(); // an extra newline for proper output visibility break; } case 3 : { // if user chooses option 3 System.out.println(" DELETE CONTACT: ---------------"); // heading for the option manager.deleteContact(); // call the deleteContact() method of the ContactManager class System.out.println(); // an extra newline for proper output visibility break; } case 4 : { // if user chooses option 4 System.out.println(" ALL CONTACTS: -------------"); // heading for the option manager.viewAllContacts(); // call the viewAllContacts() method of the ContactManager class System.out.println(); // an extra newline for proper output visibility break; } case 5: { // if user chooses option 5 System.out.println(" LOAD PHONEBOOK: ----------------"); // heading for the option manager.loadBook(); // call the loadBook() method of the ContactManager class System.out.println(); // an extra newline for proper output visibility break; } case 6: { // if user chooses option 6 System.out.println(" SAVE PHONEBOOK: ----------------"); // heading for the option manager.saveBook(); // call the saveBook() method of the ContactManager class System.out.println(); // an extra newline for proper output visibility break; } case 7: { // if user chooses to exit System.out.println(" Thanks..Goodbye! "); // display a goodbye message System.exit(0); // and exit the program successfully } default: // in case user enters an invalid menu option System.out.println(" [ERROR]: Invalid option! "); } } while (choice != 7); // loop continues until user chooses to exit by entering the option 7 } /*********************************************************************************************************************************** * This displayMenu() method displays a phone book menu to the user. * ********************************************************************************************************************************/ private static void displayMenu() { System.out.print("Choose from the following options: " + "1. Add contact 2. Modify contact 3. Delete contact 4. View all contacts" + " 5.Load phone book 6. Save phone book 7. Exit Your selection: "); } }
import java.io.File; import java.io.FileNotFoundException; import java.io.FileWriter; import java.io.IOException; import java.io.PrintWriter; import java.util.Scanner; /************************************************************************************************************************************** * ContactManager.java * This class represents the Contact manager, a phone book which manages multiple Contacts with ease. Users can add entry, * modify entry, delete entry, view all entries, save book, and load book. * @author Hitesh Kathi * @version 1.0 * @Date 06/04/2022 * ************************************************************************************************************************************/ public class ContactManager { // instance variables private Contact[] contacts; // an array containing private int size; // current size of the phone book /* creates an instance of ContactManager with the default values for all its attributes. */ public ContactManager() { contacts = new Contact[100]; // initializing the array to hold 100 Contacts, at max size = 0; // initialize the current size of the phone book to 0 } /************************************************************************************************************************************ * This getIndexOf(String name) method takes in a Contact name, searches the name in the phone book, and returns the index of it if found, * otherwise returns -1. * @param name of the Contact to be searched * ***********************************************************************************************************************************/ public int getIndexOf(String name) { int index = -1; // the index of the Contact to be searched, by default it is -1(if not found) for(int i = 0; i < 100; i++) { // a simple for-loop to search for the Contact in the phone book // if the Contact is not null and is found if(contacts[i] != null && contacts[i].getName().equalsIgnoreCase(name)) { index = i; // save the index of the Contact matched break; // and exit the loop immediately, if found } } // finally, return the index return index; } /************************************************************************************************************************************ * This viewAllContacts() method displays all the Contacts in the phone book, in a tabular format. * *****************************************************************************************************************************/ public void viewAllContacts() { // if the size of the phone book is 0, it means the phone book is empty if(size == 0) System.out.println("[ERROR]: No contacts to display!"); // else display all the contacts else { // print the table heading System.out.printf("%-25s %-15s %-25s %-1s ", "NAME", "PHONE NUMBER", "ADDRESS", "EMAIL"); // print all the contacts using a simple for-loop for(int i = 0; i < 100; i++) { if(contacts[i] != null) // print the Contact only if it is not null System.out.println(contacts[i]); } } } /************************************************************************************************************************************* * This addContact() method prompts user for all the details of a Contact, construct a Contact object using those data and adds * it to the phone book only if it is not present in the book. * ********************************************************************************************************************************/ public void addContact() { Scanner scanner = new Scanner(System.in); // a Scanner object for user input // check if size of the phone book has reached the maximum limit of 100 if(size >= 100) { // current phone book size is equal to or greater than 100 System.out.println("[ERROR]: Phone book has reached its maximum limit of 100 contacts!"); return; } System.out.print("Enter the contact name: "); // prompt for the contact name String name = scanner.nextLine().trim(); // take user input for the contact name // check if the contact with the 'name' exists in the phone book by calling the method 'getIndexOf()' if(getIndexOf(name) == -1) { // if the contact is not present in the phone book // prompt user to enter the remaining contact details System.out.print("Enter the phone number: "); // prompt for the phone number String phoneNumber = scanner.nextLine().trim(); // take user input for the phone number System.out.print("Enter the address: "); // prompt for the address String address = scanner.nextLine().trim(); // take user input for the address System.out.print("Enter the email: "); // prompt for the email String email = scanner.nextLine().trim(); // take user input for the email // construct a Contact object using the above data and add it to the phone book contacts[size++] = new Contact(name, phoneNumber, address, email); // display a success message System.out.println("[SUCCESS]: Contact added to the phone book."); } // else display message stating that contact is already present else System.out.println("[ERROR]: Contact with name " + name + " is already present in the phone book!"); } /*********************************************************************************************************************************** * This modifyContact() method takes in the name of the Contact to be modified, searches it in the phone book, and if found, asks * user for the new data, and modifies the Contact data. * ************************************************************************************************************************************/ public void modifyContact() { Scanner scanner = new Scanner(System.in); // a Scanner object for user input System.out.print("Enter the contact name: "); // prompt for the contact name String name = scanner.nextLine().trim(); // take user input for the contact name // check if the contact with the 'name' exists in the phone book by calling the method 'getIndexOf()' if(getIndexOf(name) != -1) { // if the contact is present in the phone book int index = getIndexOf(name); // record the index of the Contact found // prompt user for all data except for the Contact name System.out.print("Enter the new phone number: "); // ask for the new phone number String newPhoneNumber = scanner.nextLine().trim(); // take input from the user System.out.print("Enter the new address: "); // ask for the new address String newAddress = scanner.nextLine().trim(); // take input from the user System.out.print("Enter the new email: "); // ask for the new email address String newEmail = scanner.nextLine().trim(); // take input from the user // set all the above data to the contact contacts[index].setPhoneNumber(newPhoneNumber); // set the new phone number to the contact contacts[index].setAddress(newAddress); // set the new address to the contact contacts[index].setEmail(newEmail); // set the new phone number to the contact // display a success message to the user System.out.println("[SUCCESS]: Contact modified successfully."); } // else the contact is not present in the phone book - display a message else System.out.println("[ERROR]: Contact with name " + name + " is not present in the phone book!"); } /************************************************************************************************************************************* * This deleteContact() method takes in the name of the Contact to be deleted, searches it in the phone book, and if found, deletes * the contact by setting 'null' at that index in the phone book. * ******************************************************************************************************************************/ public void deleteContact() { Scanner scanner = new Scanner(System.in); // a Scanner object for user input System.out.print("Enter the contact name: "); // prompt for the contact name String name = scanner.nextLine().trim(); // take user input for the contact name // check if the 'contact' exists in the phone book by calling the method 'getIndexOf()' if(getIndexOf(name) != -1) { // if the contact is present in the phone book int index = getIndexOf(name); // record the index of the Contact found contacts[index] = null; // set 'null' at that index of the phone book size--; // reduce the current phone book size by 1 // display a success message to the user System.out.println("[SUCCESS]: Contact deleted successfully."); } // else the contact is not present in the phone book - display a message else System.out.println("[ERROR]: Contact with name " + name + " is not present in the phone book!"); } /*************************************************************************************************************************** * This loadBook() method reads all the Contacts from a text file "PhoneBook.txt" and puts them in the phone book. * *************************************************************************************************************************/ public void loadBook() { Scanner scanner = new Scanner(System.in); // a Scanner object for user input Scanner fileReader; //a Scanner object for reading from the file contacts = new Contact[100]; //re-initialize the phone book size = 0; //reset the phone book size String fileName = "PhoneBook.txt"; //declare a variable for the file name // a try-catch block to read from the file try { fileReader = new Scanner(new File(fileName)); // initialize the file reader to read from the file while (fileReader.hasNextLine()) { // read till the end of the file String line = fileReader.nextLine().trim(); // a line from the input file String[] tokens = line.split(","); // tokenize the lines using comma(,) as the delimiter // extract all the data into separate variables String name = tokens[0]; // get the name of the Contact String phoneNumber = tokens[1]; // get the phone number of the Contact String address = tokens[2]; // get the address of the Contact String email = tokens[3]; // get the email address of the Cont contacts[size++] = new Contact(name, phoneNumber,address, email); // construct a Contact and add it to the phone book } fileReader.close(); // System.out.println("[SUCCESS]: Phone book successfully loaded from the file."); // display a success message }catch (FileNotFoundException fnfe) { // if the file is not found, display an error message and exit the program System.out.println("[ERROR]: File " + fileName + " does not exist! Exiting the program.."); System.exit(1); } } /*********************************************************************************************************************************** * This saveBook() method writes all the Contacts in the phone book to the text file "PhoneBook.txt", all the data fields being * separated by comma(,). * **************************************************************************************************************************************/ public void saveBook() { String fileName = "PhoneBook.txt"; // declare a variable for the file name FileWriter fileWriter; // a FileWriter object PrintWriter printWriter; // a PrintWriter object // a try-catch block to write to the file try { fileWriter = new FileWriter(new File(fileName)); // initialize the FileWriter object to write to the file printWriter = new PrintWriter(fileWriter); // initialize the PrintWriter object using the FileWriter object created above for(int i = 0; i < 100; i++) { if(contacts[i] != null) // write the contact only if it is not null printWriter.write(contacts[i].getName() + "," + contacts[i].getPhoneNumber() + "," + contacts[i].getAddress() + "," + contacts[i].getEmail() + System.lineSeparator()); } // release all the resources after all the writing has been done printWriter.flush(); fileWriter.close(); printWriter.close(); // display a success message to the user System.out.println("[SUCCESS]: Phone book has been successfully saved to " + fileName + "."); }catch (IOException ioe) { // display a message in case of an error in writing data to the file, and exit the program System.out.println("[ERROR]: Error in writing contacts information to file " + fileName + ".Exiting the program.."); System.exit(1); } } }
/****************************************************************************************************************************************************** * Contact.java * This class simply represents a model of a Contact in our smartphones, with attributes: name(String), phoneNumber * * (String), address(String), and email(String). * @author Hitesh Kathi * @version 1.0 * @Date 06/04/2022 * */ public class Contact { // instance variables private String name, phoneNumber, address, email; /* creates an instance of Contact using the default value for the attributes. */ public Contact() { name = address = phoneNumber = email = ""; } /** * creates an instance of Contact using the values passed in as parameters. * @param name the name of the Contact * @param phoneNumber the phone number of the Contact * @param address the address of the Contact * @param email the email address of the Contact * */ public Contact(String name, String phoneNumber, String address,String email) { setName(name); setPhoneNumber(phoneNumber); setAddress(address); setEmail(email); } /* ACCESSOR METHODS FOR ALL ITS ATTRIBUTES */ /* This method returns the name of the Contact */ public String getName() { return name; } /* This method returns the phone number of the Contact */ public String getPhoneNumber() { return phoneNumber; } /* This method returns the address of the Contact */ public String getAddress() { return address; } /* This method returns the email address of the Contact */ public String getEmail() { return email; } /* SETTER METHODS FOR ALL ITS ATTRIBUTES */ /************************************************************************************************************************* * This setName(String name)method sets the name of the Contact. * @param name the name of the Contact to be set * ************************************************************************************************************************/ public void setName(String name) { this.name = name; } /**************************************************************************************************************************** * This setPhoneNumber(String phoneNumber) method sets the phone number of the Contact. * @param phoneNumber the phone number of the Contact to be set * ************************************************************************************************************************/ public void setPhoneNumber(String phoneNumber) { this.phoneNumber = phoneNumber; } /************************************************************************************************************************* * This setAddress(String address) method sets the address of the Contact. * @param address the address of the Contact to be set * *************************************************************************************************************************/ public void setAddress(String address) { this.address = address; } /****************************************************************************************************************************** * This setEmail(String email) method sets the email address of the Contact. * @param email the email address of the Contact to be set * ********************************************************************************************************************************/ public void setEmail(String email) { this.email = email; } /************************************************************************************************************************************** * This String toString() method returns a description of the Contact object created, which contains all the details of the Contact * object in a tabular format. * @return the details of the Contact object * **********************************************************************************************************************************/ @Override public String toString() { return (String.format("%-25s %-15s %-25s %-1s", name,phoneNumber, address, email)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
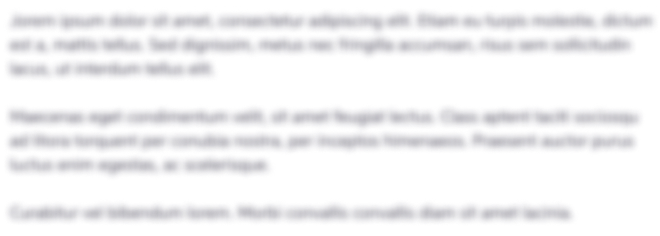
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started