Question
How to scale the base and height of triangles in an array so that it consequently scales all other aspects of the triangle (ie hypotenuse,
How to scale the base and height of triangles in an array so that it consequently scales all other aspects of the triangle (ie hypotenuse, area, perimeter etc. )
With the code that I have, it scales the hypotenuse and the perimeter, but when all of the triangles are displayed, only the hypotenuse and the perimeter are scaled by the user inputted scale factor. For this program, I have two classes, RightTriangles.java and Lab7.java.
Any help would be greatly appreciated.
The instructions for this assignment are below:
Add three instance variables to your class RightTriangle from lab 5. The first two are of type int and are called xLoc and yLoc and the third is of type int called ID. Also add a static variable of type double called scaleFactor. This should be initialized to a default value of 1. Update the constructor to set the three new instance variables and add appropriate get and set methods for the four new variables. All set methods including those from lab 5 should verify that no invalid data is set. Also define a new method ScaleShape() which multiplies the base and height instance variables by the scaleFactor and then updates the hypotenuse.
Write an application which shows the user the following menu and implements all options. The program should continue to run and process requests until the user selects 9. The program should double-check that the user really wants to exit. You may use a limit of 10 possible triangles to simplify implementation. The program should assign new ids to assure their uniqueness, the user should not enter an id for a new object in option 1. All input must be validated and appropriate feedback given for invalid input. Option 4 should display all info about the object. This is 8 method calls.
1 Enter a new right triangle
2 Delete a right triangle
3 Delete all right triangles
4 Display all right triangles
5 Move a triangle
6 Resize a triangle
7 Enter a scale factor
8 Scale all triangles
9 Exit program
My code is below:
package edu.iupui.cit27.application;
import java.util.Scanner;
public class Lab7 {
public static void main(String[] args)
{
// DATA
RightTriangle [] triangles = new RightTriangle[10];
int nextIDNumber = 1;
boolean exit = false;
int selection = 0;
Scanner input = new Scanner(System.in);
int id = 0; //managed by program
int x = 0, y = 0;
double base=0, height=0;
boolean found = false;
//Could have all variables in right triangle class and use get and set methods to access them. Avoid confusion
// ALGORITHM
// loop until user exits
do
{
// display options
System.out.println("1 - Enter a new right triangle " + " " + "2 - Delete a right triangle" + " "
+ "3 Delete all right triangles" + " " + "4 Display all right triangles" + " " + "5 Move a triangle"
+ " " + "6 - Resize a triangle" + " " + "7 Enter a scale factor" +" " + "8 Scale all triangles"
+" " +"9 - Exit program" + " " + "Enter a selection");
selection = input.nextInt();
if (selection < 0 || selection > 9)
{
System.out.println("Invalid selection." + " " + "Enter another selection" );
selection = input.nextInt();
}
// switch on selection
switch(selection)
{
case 1:
// get size from user (two variables only, calculate the hypotenuse)
System.out.print("Enter base: ");
base = input.nextDouble();
System.out.print("Enter height: ");
height = input.nextDouble();
System.out.print("Enter x location: ");
x = input.nextInt();
System.out.print("Enter y location: ");
y = input.nextInt();
found = false;
// get location from user (two variables, X,Y location)
// set found to false
// loop through array
for (int i = 0; i < triangles.length; i++)
{
// if this is an empty spot
if (triangles[i] == null)
{
// create new RightTriangle object and assign to current array element
triangles[i] = new RightTriangle(nextIDNumber++, base, height, x, y);
// set found to true
found = true;
// break out of loop
break;
}
else if (i == 9) {
// if not found, give error message
System.out.println("Error: No space found");
break;
}
}
// break out of switch statement
break;
case 2:
// get id number to delete
System.out.println("Enter the ID of the triangle you want to delete.");
id = input.nextInt();
// set found to false
found = false;
// loop through array
for (int i = 0; i < triangles.length; i++)
{
// if this is a valid object and the correct object
if (triangles[i] != null && triangles[i].GetID() == id)
{
// delete object
triangles[i] = null;
// set found to true
found = true;
// break out of loop
break;
}
}
break;
case 3:
// loop through array
for (int i = 0; i < triangles.length; i++)
{
// if this is a valid object
if (triangles[i] != null )
{
// delete object
triangles[i] = null;
}
}
// break out of switch statement
break;
case 4:
// display header
System.out.println("Display all right triangles");
// loop through array
for (int i = 0; i < triangles.length; i++)
{
// if this is a valid object
// display all info about object.
//Should call/display all 8 get methods that return information.
if (triangles[i] != null )
{
System.out.print(" ID : "+triangles[i].GetID() + " "+ " Base : "+triangles[i].GetBase() + " "+
" Height: "+ triangles[i].GetHeight() + " "+ " Area : "+triangles[i].GetArea() + " "+
" Hypotenuse: " + triangles[i].GetHypotenuse() + " "+ " Perimeter : " + triangles[i].GetPerimeter() + " "+ " X : " +
triangles[i].GetX() + " "+ " Y : "+triangles[i].GetY());
System.out.println();
}
}
// break out of switch statement
break;
case 5:
// get id number to move
System.out.println("Enter the ID of the Right triangleto be moved: ");
id = input.nextInt();
// get location from user (two variables new X,Y location)
System.out.println("Enter the X location: ");
x = input.nextInt();
System.out.println("Enter the Y location: ");
y = input.nextInt();
// set found to false
found = false;
// loop through array
for (int i = 0; i < triangles.length; i++) {
// if this is a valid object and the correct object
// call two set methods
if(triangles[i] != null && triangles[i].GetID() == id)
{
triangles[i].SetXandY(x, y );
// set found to true
found = true;
// break out of loop
break;
}
else
System.out.println("Error: ");
}
// if not found, give error message
// break out of switch statement
break;
case 6:
System.out.println("Enter the ID of the triangle you would like to resize : ");
// get id number to resize
id = input.nextInt();
// get size from user (two variables)
System.out.println("Enter base and height: ");
base = input.nextDouble();
height = input.nextDouble();
found = false;
// loop through array
for (int i = 0; i < triangles.length; i++)
{
// if this is a valid object and the correct object
if (triangles[i] != null && triangles[i].GetID() == id) {
// call SetBaseAndHeight
triangles[i].SetBaseAndHeight(base, height);
// set found to false
found = true;
// break out of loop
break;
}
}
// if not found, give error message
// break out of switch statement
break;
case 7:
// get new scale factor (validate) NEED TO VALIDATE
System.out.println("Enter Scale Factor :");
double scaleFactor = input.nextDouble();
// call SetScaleFactor to set the new scale factor
RightTriangle.setScaleFactor(scaleFactor);
// break out of switch statement
break;
case 8:
// loop through array
for (int i = 0; i < triangles.length; i++)
{
// if this is a valid object
if (triangles[i] != null )
{
// call ScaleShape method on object
triangles[i].scaleShape(triangles[i].GetBase(),
triangles[i].GetHeight(), RightTriangle.scaleFactor);
}
}
// break out of switch statement
break;
case 9:
// confirm user wants to exit
System.out.println("Do you want to exit? Press 1 if you would like to exit.");
int answer = input.nextInt();
if(answer == 1)
{
exit = true;
}
else
continue;
// set variable to break out of loop
// break out of switch statement
break;
}
// End loop
} while (!exit);
}
}
package edu.iupui.cit27.application;
import java.util.Scanner;
class RightTriangle
{
// with three instance variables of type double called base, height, and hypotenuse.
private double base;
private double height;
private double hypotenuse;
static double scaleFactor = 1;
private int id = 0;
private int xloc;
private int yloc;
// add three new instance variables
// add static scaleShape
// update constructor to take the new instance variables
public RightTriangle(int i, double b, double h, int x, int y)
{
SetBaseAndHeight(b, h);
// set the other instance variables
SetXandY(x, y);
SetID(i);
}
//Set method to take new values of x and y
public RightTriangle()
{
SetXandY(0, 0);
SetID(0);
}
// a single set method which takes new values for base and height and calculates the hypotenuse,
public void SetBaseAndHeight(double b , double h)
{
if (b > 0.0 && h > 0.0)
{
base = b;
height = h;
hypotenuse = Math.sqrt(base * base + height * height);
}
}
// add set methods for the three new instance variables
public void SetXandY(int x, int y)
{
xloc = x;
yloc = y;
}
public void SetID(int i) {
id = i;
}
// get methods for all three instance variables
public double GetBase()
{
return base;
}
public double GetHeight()
{
return height;
}
public double GetHypotenuse()
{
return hypotenuse;
}
// add get methods for the three new instance variables
public int GetX()
{
return xloc;
}
public int GetY()
{
return yloc;
}
public int GetID() {
return id;
}
// and methods getArea and getPerimeter.
public double GetArea()
{
return 0.5 * base * height;
}
public double GetPerimeter() {
return base + height + hypotenuse;
}
// add ScaleShape method
public void scaleShape(double base, double height, double scaleFactor)
{
base = base * scaleFactor;
height = height * scaleFactor;
hypotenuse = Math.sqrt(Math.pow(base, 2) + Math.pow(height, 2));
}
public static double getScaleFactor()
{
return scaleFactor;
}
public static void setScaleFactor(double scaleFactor)
{
RightTriangle.scaleFactor = scaleFactor;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
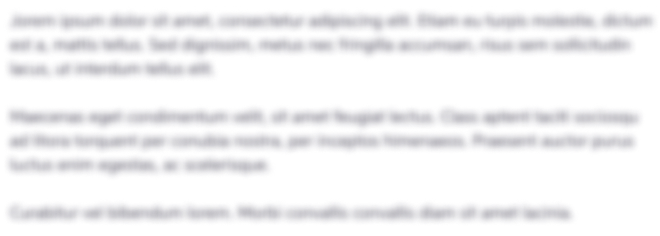
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started