Question
How would I test this code using constructors in my main program. // Code to find a student based on id // Returns a Student
How would I test this code using constructors in my main program.
// Code to find a student based on id
// Returns a Student object
Student Student::findStudent(int id) {
std::vector
students.push_back(*this);
for (int i = 0; i < students.size(); i++)
{
if (students[i].getId() == id) {
return students[i];
}
}
return Student();
}
// Code to list all students with gpa greater than the passed gpa value
// Displays appropriate message if no students are found
void Student::listStudents(double gpa) {
std::vector
students.push_back(*this);
for (int i = 0; i < students.size(); i++) {
if (students[i].getGpa() >= gpa) {
std::cout << "Student ID: " << students[i].getId() << " Name: " << students[i].getFirstName() << " "
<< students[i].getLastName() << " Major: " << students[i].getMajor() << " GPA: "
<< students[i].getGpa() << std::endl;
}
}
}
// Code to list all students with same major as one passed
// Displays appropriate message if no students are found
void Student::listStudents(string major) {
std::vector
students.push_back(*this);
for (int i = 0; i < students.size(); i++) {
if (students[i].getMajor() == major) {
std::cout << "Student ID: " << students[i].getId() << " Name: " << students[i].getFirstName() << " "
<< students[i].getLastName() << " Major: " << students[i].getMajor() << " GPA: "
<< students[i].getGpa() << std::endl;
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
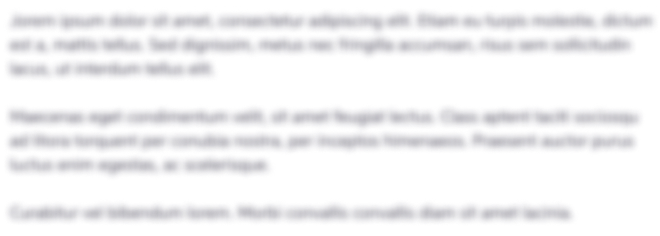
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started