Question
https://github.com/Seneca-244200/OOP-Workshops/tree/main/WS06/DIY Part 2 - DIY (50%) The Book class Your task for this part of your workshop is to complete the implementation of the Book
https://github.com/Seneca-244200/OOP-Workshops/tree/main/WS06/DIY
Part 2 - DIY (50%) The Book class
Your task for this part of your workshop is to complete the implementation of the Book class. The Book class is used to keep track of the location of a book in a library. This class encapsulates a book with respect to its title, author, shelf and bookcase number.
It is strongly recommended to use what you have learnt in part 1 of this workshop. You are free to use the code or logic of part 1 with no citation.
- Book title is a Cstring (Dynamically allocated); the maximum possible name length should be kept in constant integer (global variable) and set to 40 characters
- Author name is a Cstring(Dynamically allocated); the maximum possible title length should be kept in constant integer (global variable) and set to 25 characters
- Bookcase number; the maximum valid value for bookcase number should be kept in constant integer (global variable) and set to 132 characters
- Shelf number; the maximum valid value for a shelf number should be kept in constant integer (global variable) and set to 7 characters
Based on the maximum valid values of bookcases and shelves, the library in this program has 132 bookcases and each bookcase has 7 shelves
Validation
The attributes of the book class are validated (considered valid) as follows:
- A valid book title and author name is not null and not empty
- Bookcase number can be between 1 and the number of bookcases in the library inclusive.
- Shelf number can be between 1 and the number of bookcase shelves in the library inclusive.
The mandatory constant integer global values
- NoOfBookCases set to 132
- NoOfShelves set to 7
- MaxTitleLen set to 40
- MaxAuthorLen set to 25
The Book class should validate and store the member variables (attributes) via initialization and data entry from istream.
Also, the Book class must comply with the rule of three not to cause a crash or memory leak.
Although the title and the author of the Book are dynamically held, note that the maximum amount of memory allocated is dictated by the constant values described in the Validation section. If any attempt is made to hold Cstring values longer than the maximum limit, the action should fail, rendering the object into an invalid safe empty state.
Finally, A Book object should reveal its status (of being valid or invalid) via a type conversion overload of the Boolean type (a true outcome means the object is valid and a false outcome means it is invalid).
Mandatory Member functions and constructors
Default constructor
Sets the Book to an empty invalid state.
Four argument constructor
Sets the object attributes in the following order: title, author, bookcase number and shelf number
Rule of three
Implement all that is needed to follow the rule of three.
ostream& write(ostream& ostr, bool onScreen = true)const;
This member function outputs the Book object (if the object is valid) in two different formats based on the onScreen flag.
The output samples assume the book name is "The Alchemist" by "Paulo Coelho" held in bookcase number 25, shelf number 1
onScreen == true
prints the book as follows:
The Alchemist | Paulo Coelho | 1/025
if the object is invalid it should print:
Invalid Book Record ................... | ........................ | .....
onScreen == false
prints the book as follows:
The Alchemist,Paulo Coelho,1/25
if the object is invalid it should print:
Invalid Book Record
insertion and extraction operator overloads
insertion operator overload
Overload the insertion operator so the Book can be printed by an ostream object like cout.
Book B; cout << B << endl;
The printing format and rules should be identical to the Book::write member function
extraction operator overload
Overload the extraction operator so the Book information can be read by an istream object like cin. The book is always received using comma separated format:
The Alchemist,Paulo Coelho,1/25
If the Cstring values are too long or empty, if any of the delimiters (like comma ',' and slash '/') are missing or misplaced, or if the integer values are out of bound, the istream object should be manually set into a fail state via the function call:setstate(ios::failbit).
hint: The first two Cstrings are read using getline with (',') as the delimiter.
Book B; cout << "Please enter book information: "; cin >> B; cout << "Thanks, you entered:" << endl << B < Output: // Workshop #6: // Version: 0.9 // Date: 2021/10/17 // Author: Fardad Soleimanloo // Description: // This file tests the DIY section of your workshop /////////////////////////////////////////////////// #include int main() { constructionTest(); readAndRuleOfThreeTest(); return 0; } void constructionTest() { Book B[]{ {"","abc", 48, 6}, {nullptr,"abc", 48, 6}, {"abc", "", 48, 6}, {"abc", nullptr, 48, 6}, {"abc","abc", 0, 6}, {"abc","abc", 48, 0}, {"Huckleberry Finn","Mark Twain", NoOfBookCases,NoOfShelves}, {"Huckleberry Finn","Mark Twain", NoOfBookCases + 1,NoOfShelves}, {"Huckleberry Finn","Mark Twain",NoOfBookCases,NoOfShelves + 1} }; hr("Constructor test"); header(); for (int i = 0; i < 8; cout << B[i++] << endl); hr("End Constructor test"); } void readAndRuleOfThreeTest() { ifstream bookFile("books.csv"); ofstream goodBooks("goodBooks.csv"); Book B; int noOfRecs = ::noOfRecs("books.csv"); int noOfReads = 0; int i; hr("Read and Rule of three"); header(); for (i = 0; i < noOfRecs; i++) { B = readBook(bookFile); printbookRow(i, B); if (bookFile) { B.write(goodBooks, false) << endl; } else { bookFile.clear(); } bookFile.ignore(MaxAuthorLen + MaxTitleLen + 7, ' '); } goodBooks.close(); bookFile.close(); bookFile.open("goodBooks.csv"); hr("+++ Good Books"); header(); for (i = 0; bookFile >> B; i++) { bookFile.ignore(MaxAuthorLen + MaxTitleLen + 7, ' '); printbookRow(i, B); } hr("End Read and Rule of three"); } Book readBook(ifstream& file) { Book B; file >> B; return B; } void hr(const char* title) { int len = 0; if (title) cout << title << " "; while (title && *title++)len++; cout.width(78 - len + !title); cout.fill('-'); cout << '-' << endl; cout.fill(' '); } int noOfRecs(const char* filename) { int num = 0; ifstream file(filename); while (file) num += (file.get() == ' '); return num; } void header() { cout << "Row: Book Title | Author(s) | Loc" << endl << "---------------------------------------------+--------------------------+------" << endl; } void printbookRow(int row, Book B) { cout.width(3); cout.fill('0'); cout.setf(ios::right); cout << (row + 1) << ": "; cout.fill(' '); cout.unsetf(ios::right); cout << B << endl;; } /* Example of the output of a book row with a ruler to help for counting. 1 2 3 4 5 6 7 8 12345678901234567890123456789012345678901234567890123456789012345678901234567890 Huckleberry Finn | Mark Twain | 7/132 The Alchemist | Paulo Coelho | 1/025 The Great Gatsby | F. Scott Fitzgerald | 1/033 */ Constructor test -------------------------------------------------------------- Row: Book Title | Author(s) | Loc ---------------------------------------------+--------------------------+------ Invalid Book Record ................... | ........................ | ..... Invalid Book Record ................... | ........................ | ..... Invalid Book Record ................... | ........................ | ..... Invalid Book Record ................... | ........................ | ..... Invalid Book Record ................... | ........................ | ..... Invalid Book Record ................... | ........................ | ..... Huckleberry Finn | Mark Twain | 7/132 Invalid Book Record ................... | ........................ | ..... End Constructor test ---------------------------------------------------------- Read and Rule of three -------------------------------------------------------- Row: Book Title | Author(s) | Loc ---------------------------------------------+--------------------------+------ 001: Harry Potter and the Chamber of Secrets | J. K. Rowling | 2/056 002: Harry Potter and the Prisoner of Azkaban| J. K. Rowling | 4/126 003: Harry Potter and the Goblet of Fire | J. K. Rowling | 4/107 004: Invalid Book Record ................... | ........................ | ..... 005: Harry Potter and the Half-Blood Prince | J. K. Rowling | 4/061 006: Harry Potter and the Deathly Hallows | J. K. Rowling | 6/080 007: The Alchemist | Paulo Coelho | 1/025 008: The Catcher in the Rye | J. D. Salinger | 3/121 009: The Bridges of Madison County | Robert James Waller | 6/021 010: Ben-Hur: A Tale of the Christ | Lew Wallace | 5/132 011: You Can Heal Your Life | Louise Hay | 1/016 012: One Hundred Years of Solitude | Gabriel Garca Mrquez | 3/086 013: Lolita | Vladimir Nabokov | 6/127 014: Heidi | Johanna Spyri | 5/054 015: Invalid Book Record ................... | ........................ | ..... 016: Anne of Green Gables | Lucy Maud Montgomery | 6/010 017: Black Beauty | Anna Sewell | 2/073 018: The Name of the Rose | Umberto Eco | 5/123 019: The Eagle Has Landed | Jack Higgins | 6/129 020: Watership Down | Richard Adams | 5/122 021: The Hite Report | Shere Hite | 3/126 022: Invalid Book Record ................... | ........................ | ..... 023: The Tale of Peter Rabbit | Beatrix Potter | 6/078 024: Jonathan Livingston Seagull | Richard Bach | 6/102 025: The Very Hungry Caterpillar | Eric Carle | 2/116 026: A Message to Garcia | Elbert Hubbard | 3/118 027: To Kill a Mockingbird | Harper Lee | 5/032 028: Flowers in the Attic | V. C. Andrews | 7/073 029: Cosmos | Carl Sagan | 1/030 030: Sophie's World | Jostein Gaarder | 5/049 031: Angels & Demons | Dan Brown | 4/069 032: Kane and Abel | Jeffrey Archer | 4/121 033: How the Steel Was Tempered | Nikolai Ostrovsky | 6/060 034: War and Peace | Leo Tolstoy | 3/064 035: The Diary of Anne Frank | Anne Frank | 4/099 036: Your Erroneous Zones | Wayne Dyer | 7/055 037: The Thorn Birds | Colleen McCullough | 2/020 038: The Purpose Driven Life | Rick Warren | 1/097 039: The Kite Runner | Khaled Hosseini | 6/007 040: Valley of the Dolls | Jacqueline Susann | 3/028 041: The Great Gatsby | F. Scott Fitzgerald | 1/033 042: Gone with the Wind | Margaret Mitchell | 2/005 043: Rebecca | Daphne du Maurier | 6/064 044: Nineteen Eighty-Four | George Orwell | 1/020 045: The Revolt of Mamie Stover | William Bradford Huie | 2/075 046: The Girl with the Dragon Tattoo | Stieg Larsson | 2/044 047: The Lost Symbol | Dan Brown | 4/062 048: The Hunger Games | Suzanne Collins | 7/100 049: James and the Giant Peach | Roald Dahl | 6/099 050: Invalid Book Record ................... | ........................ | ..... 051: Who Moved My Cheese? | Spencer Johnson | 6/048 052: A Brief History of Time | Stephen Hawking | 4/086 053: Invalid Book Record ................... | ........................ | ..... 054: Lust for Life | Irving Stone | 3/046 055: The Wind in the Willows | Kenneth Grahame | 3/092 056: The Happy Hooker: My Own Story | Xaviera Hollander | 6/090 057: Jaws | Peter Benchley | 6/020 058: Love You Forever | Robert Munsch | 6/066 059: The Women's Room | Marilyn French | 5/016 060: Invalid Book Record ................... | ........................ | ..... 061: Adventures of Huckleberry Finn | Mark Twain | 3/114 062: Invalid Book Record ................... | ........................ | ..... 063: Pride and Prejudice | Jane Austen | 5/021 064: Kon-Tiki: Across the Pacific in a Raft | Thor Heyerdahl | 4/027 065: The Good Soldier Svejk | Jaroslav Haek | 7/090 066: Where the Wild Things Are | Maurice Sendak | 6/019 067: The Power of Positive Thinking | Norman Vincent Peale | 2/008 068: The Secret | Rhonda Byrne | 2/040 069: Fear of Flying | Erica Jong | 5/012 070: Dune | Frank Herbert | 3/083 071: Charlie and the Chocolate Factory | Roald Dahl | 2/020 072: The Naked Ape | Desmond Morris | 5/036 073: Invalid Book Record ................... | ........................ | ..... 074: Matilda | Roald Dahl | 6/055 075: The Total Woman | Marabel Morgan | 3/094 076: Knowledge-value Revolution | Taichi Sakaiya | 6/027 077: Problems in China's Socialist Economy | Xue Muqiao | 6/060 078: What Color Is Your Parachute? | Richard Nelson Bolles | 5/130 079: The Dukan Diet | Pierre Dukan | 1/115 080: Invalid Book Record ................... | ........................ | ..... 081: The Gospel According to Peanuts | Robert L. Short | 7/053 082: Life of Pi | Yann Martel | 2/037 083: The Giver | Lois Lowry | 5/064 084: The Front Runner | Patricia Nell Warren | 2/119 085: Invalid Book Record ................... | ........................ | ..... 086: Fahrenheit 451 | Ray Bradbury | 2/108 087: Angela's Ashes | Frank McCourt | 1/078 088: Invalid Book Record ................... | ........................ | ..... 089: Bridget Jones's Diary | Helen Fielding | 5/075 090: Harry Potter | J. K. Rowling | 1/045 091: Invalid Book Record ................... | ........................ | ..... 092: Perry Mason | Erle Stanley Gardner | 7/005 093: Berenstain Bears | Stan and Jan Berenstain | 5/093 094: Choose Your Own Adventure | Various authors | 4/008 095: Diary of a Wimpy Kid | Jeff Kinney | 2/041 096: Invalid Book Record ................... | ........................ | ..... 097: Noddy | Enid Blyton | 4/097 098: Invalid Book Record ................... | ........................ | ..... 099: Invalid Book Record ................... | ........................ | ..... 100: San-Antonio | Frdric Dard | 5/073 101: Robert Langdon | Dan Brown | 4/019 102: The Baby-sitters Club | Ann Martin | 5/002 103: Twilight | Stephenie Meyer | 4/025 104: Star Wars | Various authors | 2/113 105: Little Critter | Mercer Mayer | 3/115 106: Peter Rabbit | Beatrix Potter | 5/036 107: Fifty Shades | E. L. James | 3/063 108: American Girl | Various authors | 5/020 109: Invalid Book Record ................... | ........................ | ..... 110: Invalid Book Record ................... | ........................ | ..... 111: Clifford the Big Red Dog | Norman Bridwell | 5/108 112: Frank Merriwell | Gilbert Patten | 2/077 113: Dirk Pitt | Clive Cussler | 6/030 114: Musashi | Eiji Yoshikawa | 5/081 115: The Chronicles of Narnia | C. S. Lewis | 4/100 116: Invalid Book Record ................... | ........................ | ..... 117: The Hunger Games trilogy | Suzanne Collins | 5/051 118: James Bond | Ian Fleming | 3/071 119: Invalid Book Record ................... | ........................ | ..... 120: Invalid Book Record ................... | ........................ | ..... 121: A Song of Ice and Fire | George R. R. Martin | 2/121 122: Discworld | Terry Pratchett | 6/039 123: Nijntje | Dick Bruna | 6/007 124: Alex Cross | James Patterson | 2/096 125: Invalid Book Record ................... | ........................ | ..... 126: Captain Underpants | Dav Pilkey | 1/072 127: Fear Street | R. L. Stine | 3/078 128: Pippi Longstocking | Astrid Lindgren | 7/022 129: The Vampire Chronicles | Anne Rice | 3/030 130: Invalid Book Record ................... | ........................ | ..... 131: OSS 117 | Jean Bruce | 3/020 132: Invalid Book Record ................... | ........................ | ..... 133: Magic Tree House series | Mary Pope Osborne | 4/096 134: Invalid Book Record ................... | ........................ | ..... 135: Invalid Book Record ................... | ........................ | ..... 136: Little House on the Prairie | Laura Ingalls Wilder | 3/114 137: Jack Reacher | Lee Child | 5/118 138: Invalid Book Record ................... | ........................ | ..... 139: Where's Wally? | Martin Handford | 7/015 140: Men Are from Mars- Women Are from Venus | John Gray | 3/020 141: Invalid Book Record ................... | ........................ | ..... 142: Invalid Book Record ................... | ........................ | ..... 143: Tarzan | Edgar Rice Burroughs | 3/093 +++ Good Books ---------------------------------------------------------------- Row: Book Title | Author(s) | Loc ---------------------------------------------+--------------------------+------ 001: Harry Potter and the Chamber of Secrets | J. K. Rowling | 2/056 002: Harry Potter and the Prisoner of Azkaban| J. K. Rowling | 4/126 003: Harry Potter and the Goblet of Fire | J. K. Rowling | 4/107 004: Harry Potter and the Half-Blood Prince | J. K. Rowling | 4/061 005: Harry Potter and the Deathly Hallows | J. K. Rowling | 6/080 006: The Alchemist | Paulo Coelho | 1/025 007: The Catcher in the Rye | J. D. Salinger | 3/121 008: The Bridges of Madison County | Robert James Waller | 6/021 009: Ben-Hur: A Tale of the Christ | Lew Wallace | 5/132 010: You Can Heal Your Life | Louise Hay | 1/016 011: One Hundred Years of Solitude | Gabriel Garca Mrquez | 3/086 012: Lolita | Vladimir Nabokov | 6/127 013: Heidi | Johanna Spyri | 5/054 014: Anne of Green Gables | Lucy Maud Montgomery | 6/010 015: Black Beauty | Anna Sewell | 2/073 016: The Name of the Rose | Umberto Eco | 5/123 017: The Eagle Has Landed | Jack Higgins | 6/129 018: Watership Down | Richard Adams | 5/122 019: The Hite Report | Shere Hite | 3/126 020: The Tale of Peter Rabbit | Beatrix Potter | 6/078 021: Jonathan Livingston Seagull | Richard Bach | 6/102 022: The Very Hungry Caterpillar | Eric Carle | 2/116 023: A Message to Garcia | Elbert Hubbard | 3/118 024: To Kill a Mockingbird | Harper Lee | 5/032 025: Flowers in the Attic | V. C. Andrews | 7/073 026: Cosmos | Carl Sagan | 1/030 027: Sophie's World | Jostein Gaarder | 5/049 028: Angels & Demons | Dan Brown | 4/069 029: Kane and Abel | Jeffrey Archer | 4/121 030: How the Steel Was Tempered | Nikolai Ostrovsky | 6/060 031: War and Peace | Leo Tolstoy | 3/064 032: The Diary of Anne Frank | Anne Frank | 4/099 033: Your Erroneous Zones | Wayne Dyer | 7/055 034: The Thorn Birds | Colleen McCullough | 2/020 035: The Purpose Driven Life | Rick Warren | 1/097 036: The Kite Runner | Khaled Hosseini | 6/007 037: Valley of the Dolls | Jacqueline Susann | 3/028 038: The Great Gatsby | F. Scott Fitzgerald | 1/033 039: Gone with the Wind | Margaret Mitchell | 2/005 040: Rebecca | Daphne du Maurier | 6/064 041: Nineteen Eighty-Four | George Orwell | 1/020 042: The Revolt of Mamie Stover | William Bradford Huie | 2/075 043: The Girl with the Dragon Tattoo | Stieg Larsson | 2/044 044: The Lost Symbol | Dan Brown | 4/062 045: The Hunger Games | Suzanne Collins | 7/100 046: James and the Giant Peach | Roald Dahl | 6/099 047: Who Moved My Cheese? | Spencer Johnson | 6/048 048: A Brief History of Time | Stephen Hawking | 4/086 049: Lust for Life | Irving Stone | 3/046 050: The Wind in the Willows | Kenneth Grahame | 3/092 051: The Happy Hooker: My Own Story | Xaviera Hollander | 6/090 052: Jaws | Peter Benchley | 6/020 053: Love You Forever | Robert Munsch | 6/066 054: The Women's Room | Marilyn French | 5/016 055: Adventures of Huckleberry Finn | Mark Twain | 3/114 056: Pride and Prejudice | Jane Austen | 5/021 057: Kon-Tiki: Across the Pacific in a Raft | Thor Heyerdahl | 4/027 058: The Good Soldier Svejk | Jaroslav Haek | 7/090 059: Where the Wild Things Are | Maurice Sendak | 6/019 060: The Power of Positive Thinking | Norman Vincent Peale | 2/008 061: The Secret | Rhonda Byrne | 2/040 062: Fear of Flying | Erica Jong | 5/012 063: Dune | Frank Herbert | 3/083 064: Charlie and the Chocolate Factory | Roald Dahl | 2/020 065: The Naked Ape | Desmond Morris | 5/036 066: Matilda | Roald Dahl | 6/055 067: The Total Woman | Marabel Morgan | 3/094 068: Knowledge-value Revolution | Taichi Sakaiya | 6/027 069: Problems in China's Socialist Economy | Xue Muqiao | 6/060 070: What Color Is Your Parachute? | Richard Nelson Bolles | 5/130 071: The Dukan Diet | Pierre Dukan | 1/115 072: The Gospel According to Peanuts | Robert L. Short | 7/053 073: Life of Pi | Yann Martel | 2/037 074: The Giver | Lois Lowry | 5/064 075: The Front Runner | Patricia Nell Warren | 2/119 076: Fahrenheit 451 | Ray Bradbury | 2/108 077: Angela's Ashes | Frank McCourt | 1/078 078: Bridget Jones's Diary | Helen Fielding | 5/075 079: Harry Potter | J. K. Rowling | 1/045 080: Perry Mason | Erle Stanley Gardner | 7/005 081: Berenstain Bears | Stan and Jan Berenstain | 5/093 082: Choose Your Own Adventure | Various authors | 4/008 083: Diary of a Wimpy Kid | Jeff Kinney | 2/041 084: Noddy | Enid Blyton | 4/097 085: San-Antonio | Frdric Dard | 5/073 086: Robert Langdon | Dan Brown | 4/019 087: The Baby-sitters Club | Ann Martin | 5/002 088: Twilight | Stephenie Meyer | 4/025 089: Star Wars | Various authors | 2/113 090: Little Critter | Mercer Mayer | 3/115 091: Peter Rabbit | Beatrix Potter | 5/036 092: Fifty Shades | E. L. James | 3/063 093: American Girl | Various authors | 5/020 094: Clifford the Big Red Dog | Norman Bridwell | 5/108 095: Frank Merriwell | Gilbert Patten | 2/077 096: Dirk Pitt | Clive Cussler | 6/030 097: Musashi | Eiji Yoshikawa | 5/081 098: The Chronicles of Narnia | C. S. Lewis | 4/100 099: The Hunger Games trilogy | Suzanne Collins | 5/051 100: James Bond | Ian Fleming | 3/071 101: A Song of Ice and Fire | George R. R. Martin | 2/121 102: Discworld | Terry Pratchett | 6/039 103: Nijntje | Dick Bruna | 6/007 104: Alex Cross | James Patterson | 2/096 105: Captain Underpants | Dav Pilkey | 1/072 106: Fear Street | R. L. Stine | 3/078 107: Pippi Longstocking | Astrid Lindgren | 7/022 108: The Vampire Chronicles | Anne Rice | 3/030 109: OSS 117 | Jean Bruce | 3/020 110: Magic Tree House series | Mary Pope Osborne | 4/096 111: Little House on the Prairie | Laura Ingalls Wilder | 3/114 112: Jack Reacher | Lee Child | 5/118 113: Where's Wally? | Martin Handford | 7/015 114: Men Are from Mars- Women Are from Venus | John Gray | 3/020 115: Tarzan | Edgar Rice Burroughs | 3/093 End Read and Rule of three ---------------------------------------------------- Please enter book information: The Alchemist,Paulo Coelho,1/25 Thanks, you entered: The Alchemist | Paulo Coelho | 1/025
Step by Step Solution
There are 3 Steps involved in it
Step: 1
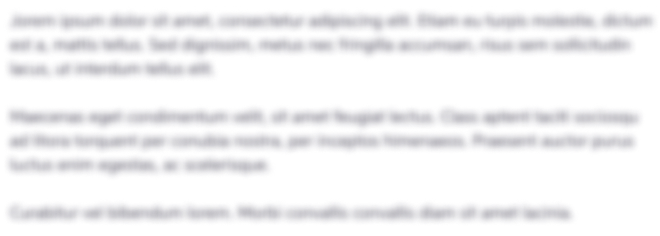
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started