Question
https://imgur.com/a/8YZFS instructions can be found in this picture. And the 4 classes are below but only SinglyLinkedList should be edited.And a Junit class has been
https://imgur.com/a/8YZFS instructions can be found in this picture. And the 4 classes are below but only SinglyLinkedList should be edited.And a Junit class has been provided
/** * Your implementation of a circular singly linked list. * * @author YOUR NAME HERE * @userid YOUR USER ID HERE (e.g. gburdell3) * @GTID YOUR GT ID HERE (e.g. 900000000) * @version 1.0 */ public class SinglyLinkedList
@Override public void addAtIndex(T data, int index) {
}
@Override public void addToFront(T data) {
}
@Override public void addToBack(T data) {
}
@Override public T removeAtIndex(int index) {
}
@Override public T removeFromFront() {
}
@Override public T removeFromBack() {
}
@Override public T removeLastOccurrence(T data) {
}
@Override public T get(int index) {
}
@Override public Object[] toArray() {
}
@Override public boolean isEmpty() {
}
@Override public void clear() {
}
@Override public int size() { // DO NOT MODIFY! return size; }
@Override public LinkedListNode
/** * This interface describes the expected behavior of the public methods * needed for a circular singly linked list with a head pointer. The required * efficiencies have also been provided. * * DO NOT ALTER THIS FILE!! * * @author CS 1332 TAs * @version 1.0 */ public interface LinkedListInterface
/** * Adds the element to the index specified. * * Adding to indices 0 and {@code size} should be O(1), all other cases are * O(n). * * @param index the requested index for the new element * @param data the data for the new element * @throws java.lang.IndexOutOfBoundsException if index is negative or * index > size * @throws java.lang.IllegalArgumentException if data is null */ void addAtIndex(T data, int index);
/** * Adds the element to the front of the list. * * Must be O(1) for all cases. * * @param data the data for the new element * @throws java.lang.IllegalArgumentException if data is null. */ void addToFront(T data);
/** * Adds the element to the back of the list. * * Must be O(1) for all cases. * * @param data the data for the new element * @throws java.lang.IllegalArgumentException if data is null. */ void addToBack(T data);
/** * Removes and returns the element from the index specified. * * Removing from index 0 should be O(1), all other cases are * O(n). * * @param index the requested index to be removed * @return the data formerly located at index * @throws java.lang.IndexOutOfBoundsException if index is negative or * index >= size */ T removeAtIndex(int index);
/** * Removes and returns the element at the front of the list. If the list is * empty, return {@code null}. * * Must be O(1) for all cases. * * @return the data formerly located at the front, null if empty list */ T removeFromFront();
/** * Removes and returns the element at the back of the list. If the list is * empty, return {@code null}. * * Must be O(n) for all cases. * * @return the data formerly located at the back, null if empty list */ T removeFromBack();
/** * Removes the last copy of the given data from the list. * * Must be O(n) for all cases. * * @param data the data to be removed from the list * @return the removed data occurrence from the list itself (not the data * passed in), null if no occurrence * @throws java.lang.IllegalArgumentException if data is null */ T removeLastOccurrence(T data);
/** * Returns the element at the specified index. * * Getting index 0 should be O(1), all other cases are O(n). * * @param index the index of the requested element * @return the object stored at index * @throws java.lang.IndexOutOfBoundsException if index < 0 or * index >= size */ T get(int index);
/** * Returns an array representation of the linked list. * * Must be O(n) for all cases. * * @return an array of length {@code size} holding all of the objects in * this list in the same order */ Object[] toArray();
/** * Returns a boolean value indicating if the list is empty. * * Must be O(1) for all cases. * * @return true if empty; false otherwise */ boolean isEmpty();
/** * Clears the list of all data. * * Must be O(1) for all cases. */ void clear();
/** * Returns the number of elements in the list. * * Runs in O(1) for all cases. * * @return the size of the list */ int size();
/** * Returns the head node of the linked list. * Normally, you would not do this, but it's necessary for testing purposes. * * DO NOT USE THIS METHOD IN YOUR CODE. * * @return node at the head of the linked list */ LinkedListNode
/** * Node class used for implementing the circular SinglyLinkedList. * * DO NOT ALTER THIS FILE!! * * @author CS 1332 TAs * @version 1.0 */
public class LinkedListNode
/** * Creates a new LinkedListNode with the given T object and next reference. * * @param data the data stored in the new node * @param next the next node in the list */ public LinkedListNode(T data, LinkedListNode
/** * Creates a new LinkedListNode with only the given T object. * * @param data the data stored in the new node */ public LinkedListNode(T data) { this(data, null); }
/** * Gets the data stored in the node. * * @return the data in this node */ public T getData() { return data; }
/** * Sets the data stored in the node. * * @param data the new data */ public void setData(T data) { this.data = data; }
/** * Gets the next node. * * @return the next node */ public LinkedListNode
/** * Sets the next node. * * @param next the new next node */ public void setNext(LinkedListNode
@Override public String toString() { return "Node containing: " + data; } }
import org.junit.Test; import org.junit.Before;
import static org.junit.Assert.assertArrayEquals; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertNotNull; import static org.junit.Assert.assertNull; import static org.junit.Assert.assertSame;
/** * This is a basic set of unit tests for SinglyLinkedList. Passing these does * NOT guarantee any grade on this assignment. This is only a sanity check to * help you get started on the homework and writing JUnits in general. * * @author The 1332 TAs * @version 1.0 */ public class LinkedListStudentTests { private LinkedListInterface
public static final int TIMEOUT = 200;
@Before public void setUp() { list = new SinglyLinkedList
@Test(timeout = TIMEOUT) public void testAddStrings() { assertEquals(0, list.size()); assertNull(list.getHead());
list.addAtIndex("0a", 0); //0a list.addAtIndex("1a", 1); //0a 1a list.addAtIndex("2a", 2); //0a 1a 2a list.addAtIndex("3a", 3); //0a 1a 2a 3a
assertEquals(4, list.size());
LinkedListNode
current = current.getNext(); assertNotNull(current); assertEquals("1a", current.getData());
current = current.getNext(); assertNotNull(current); assertEquals("2a", current.getData());
current = current.getNext(); assertNotNull(current); assertEquals("3a", current.getData());
current = current.getNext(); assertNotNull(current); assertSame(list.getHead(), current); }
@Test(timeout = TIMEOUT) public void testAddStringsFront() { assertEquals(0, list.size());
list.addToFront("0a"); list.addToFront("1a"); list.addToFront("2a"); list.addToFront("3a"); list.addToFront("4a"); list.addToFront("5a"); //5a 4a 3a 2a 1a 0a
assertEquals(6, list.size());
LinkedListNode
current = current.getNext(); assertNotNull(current); assertEquals("4a", current.getData());
current = current.getNext(); assertNotNull(current); assertEquals("3a", current.getData());
current = current.getNext(); assertNotNull(current); assertEquals("2a", current.getData());
current = current.getNext(); assertNotNull(current); assertEquals("1a", current.getData());
current = current.getNext(); assertNotNull(current); assertEquals("0a", current.getData());
current = current.getNext(); assertNotNull(current); assertSame(list.getHead(), current); }
@Test(timeout = TIMEOUT) public void testRemoveStrings() { assertEquals(0, list.size());
list.addAtIndex("0a", 0); list.addAtIndex("1a", 1); list.addAtIndex("2a", 2); list.addAtIndex("3a", 3); list.addAtIndex("4a", 4); list.addAtIndex("5a", 5); //0a 1a 2a 3a 4a 5a
assertEquals(6, list.size());
assertEquals("2a", list.removeAtIndex(2)); //0a 1a 3a 4a 5a
assertEquals(5, list.size());
LinkedListNode
current = current.getNext(); assertNotNull(current); assertEquals("1a", current.getData());
current = current.getNext(); assertNotNull(current); assertEquals("3a", current.getData());
current = current.getNext(); assertNotNull(current); assertEquals("4a", current.getData());
current = current.getNext(); assertNotNull(current); assertEquals("5a", current.getData());
current = current.getNext(); assertNotNull(current); assertSame(list.getHead(), current); }
@Test(timeout = TIMEOUT) public void testGetGeneral() { list.addAtIndex("0a", 0); list.addAtIndex("1a", 1); list.addAtIndex("2a", 2); list.addAtIndex("3a", 3); list.addAtIndex("4a", 4); //0a 1a 2a 3a 4a
assertEquals("0a", list.get(0)); assertEquals("1a", list.get(1)); assertEquals("2a", list.get(2)); assertEquals("3a", list.get(3)); assertEquals("4a", list.get(4)); }
@Test(timeout = TIMEOUT) public void testToArray() { String[] expectedItems = new String[10];
for (int x = 0; x < expectedItems.length; x++) { expectedItems[x] = "a" + x; list.addToBack(expectedItems[x]); }
Object[] array = list.toArray(); assertArrayEquals(expectedItems, array); }
@Test(timeout = TIMEOUT, expected = IllegalArgumentException.class) public void removeLastOccurrenceNullItemPassed() { list.removeLastOccurrence(null); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
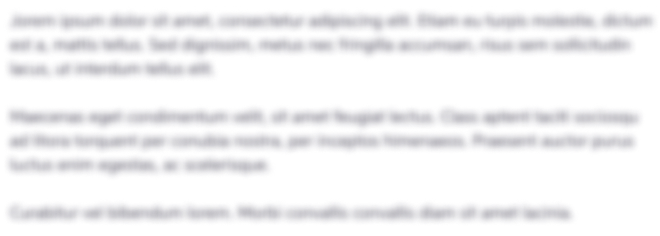
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started