Question
https://www.chegg.com/homework-help/questions-and-answers/class-workoutclass-workout-class-offered-gym-private-attributes-name-name-workoutclass-req-q44040301 def offerings_at(self, time_point: datetime) -> list[dict[str, str | int]]: Return a list of dictionaries, each representing a workout offered at this Gym at .
https://www.chegg.com/homework-help/questions-and-answers/class-workoutclass-workout-class-offered-gym-private-attributes-name-name-workoutclass-req-q44040301
def offerings_at(self, time_point: datetime) -> list[dict[str, str | int]]: """Return a list of dictionaries, each representing a workout offered at this Gym at
The offerings should be sorted by room name, in alphabetical ascending order.
Each dictionary must have the following keys and values: 'Date': the weekday and date of the class as a string, in the format 'Weekday, year-month-day' (e.g., 'Monday, 2022-11-07') 'Time': the time of the class, in the format 'HH:MM' where HH uses 24-hour time (e.g., '15:00') 'Class': the name of the class 'Room': the name of the room 'Registered': the number of people already registered for the class 'Available': the number of spots still available in the class 'Instructor': the name of the instructor If there are multiple instructors with the same name, the name should be followed by the instructor ID in parentheses e.g., "Diane (1)"
If there are no offerings at
NOTE: - You MUST use the helper function create_offering_dict from gym_utilities to create the dictionaries, in order to make sure you match the format specified above. - You MUST use the helper method _is_instructor_name_unique when deciding how to format the instructor name.
>>> ac = Gym('Athletic Centre') >>> diane1 = Instructor(1, 'Diane') >>> diane1.add_certificate('Cardio 1') True >>> diane2 = Instructor(2, 'Diane') >>> david = Instructor(3, 'David') >>> david.add_certificate('Strength Training') True >>> ac.add_instructor(diane1) True >>> ac.add_instructor(diane2) True >>> ac.add_instructor(david) True >>> ac.add_room('Dance Studio', 50) True >>> ac.add_room('Room A', 20) True >>> boot_camp = WorkoutClass('Boot Camp', ['Cardio 1']) >>> ac.add_workout_class(boot_camp) True >>> kickboxing = WorkoutClass('KickBoxing', ['Strength Training']) >>> ac.add_workout_class(kickboxing) True >>> t1 = datetime(2022, 9, 9, 12, 0) >>> ac.schedule_workout_class(t1, 'Dance Studio', boot_camp.name, 1) True >>> ac.schedule_workout_class(t1, 'Room A', kickboxing.name, 3) True >>> ac.offerings_at(t1) == [ ... { 'Date': 'Friday, 2022-09-09', 'Time': '12:00', ... 'Class': 'Boot Camp', 'Room': 'Dance Studio', 'Registered': 0, ... 'Available': 50, 'Instructor': 'Diane (1)' }, ... { 'Date': 'Friday, 2022-09-09', 'Time': '12:00', ... 'Class': 'KickBoxing', 'Room': 'Room A', 'Registered': 0, ... 'Available': 20, 'Instructor': 'David' } ... ] True """ pass
def to_schedule_list(self, week: datetime = None) -> list[dict[str, str | int]]: """Return a list of dictionaries for the Gym's entire schedule, with each dictionary representing a workout offered (in the format specified by the docstring for offerings_at).
The dictionaries should be in the list in ascending order by their date and time (not the string representation of the date and time). Offerings occurring at exactly the same date and time should be in alphabetical order based on their room names.
If
Hint: The helper function
>>> ac = Gym('Athletic Centre') >>> diane1 = Instructor(1, 'Diane') >>> diane1.add_certificate('Cardio 1') True >>> diane2 = Instructor(2, 'Diane') >>> david = Instructor(3, 'David') >>> david.add_certificate('Strength Training') True >>> ac.add_instructor(diane1) True >>> ac.add_instructor(diane2) True >>> ac.add_instructor(david) True >>> ac.add_room('Studio 1', 20) True >>> boot_camp = WorkoutClass('Boot Camp', ['Cardio 1']) >>> ac.add_workout_class(boot_camp) True >>> kickboxing = WorkoutClass('KickBoxing', ['Strength Training']) >>> ac.add_workout_class(kickboxing) True >>> t1 = datetime(2022, 9, 9, 12, 0) >>> ac.schedule_workout_class(t1, 'Studio 1', boot_camp.name, 1) True >>> t2 = datetime(2022, 9, 8, 13, 0) >>> ac.schedule_workout_class(t2, 'Studio 1', kickboxing.name, 3) True >>> ac.to_schedule_list() == [ ... { 'Date': 'Thursday, 2022-09-08', 'Time': '13:00', ... 'Class': 'KickBoxing', 'Room': 'Studio 1', 'Registered': 0, ... 'Available': 20, 'Instructor': 'David' }, ... { 'Date': 'Friday, 2022-09-09', 'Time': '12:00', ... 'Class': 'Boot Camp', 'Room': 'Studio 1', 'Registered': 0, ... 'Available': 20, 'Instructor': 'Diane (1)' }, ... ] True """ pass
please write the code in python and use the helper functions thankyou
def _is_instructor_name_unique(self, instructor: Instructor) -> bool: """Return True iff the name of
>>> ac = Gym('Athletic Centre') >>> first_hire = Instructor(1, 'Diane') >>> ac.add_instructor(first_hire) True >>> ac._is_instructor_name_unique(first_hire) True >>> second_hire = Instructor(2, 'Diane') >>> ac.add_instructor(second_hire) True >>> ac._is_instructor_name_unique(first_hire) False >>> ac._is_instructor_name_unique(second_hire) False >>> third_hire = Instructor(3, 'Tom') >>> ac._is_instructor_name_unique(third_hire) True """ name_count = 0 for inst in self._instructors.values(): if inst.name == instructor.name: name_count += 1 return name_count <= 1
def in_week(date: datetime, week: datetime = None) -> bool: """Return True iff
A week is defined as the period from Monday 0:00 to Sunday 23:59. Return True if no week is provided.
Hint: You may find this helper function useful in your own code.
>>> # Note: You can create a datetime that has only year, month, day, or >>> # you can optionally specify hour, minute, etc. >>> in_week(datetime(2022, 9, 1, 12, 0), datetime(2022, 8, 31)) True >>> in_week(datetime(2022, 9, 1, 12, 0), datetime(2022, 9, 7)) False >>> in_week(datetime(2022, 9, 1, 12, 0), datetime(202, 9, 8)) False >>> in_week(datetime(2023, 1, 1), datetime(2022, 12, 31)) True >>> in_week(datetime(2023, 1, 1)) True """ if not week: return True # find the first and last day of the calendar week containing
def create_offering_dict(date: str, time: str, workout_class_name: str, room_name: str, num_registered_participants: int, num_available_seats: int, instructor_name: str) -> dict[str, str | int]: """Return a dictionary that represents all the given attributes of a workout offering in a standardized format.
Hint: You should use this helper function in the
Step by Step Solution
There are 3 Steps involved in it
Step: 1
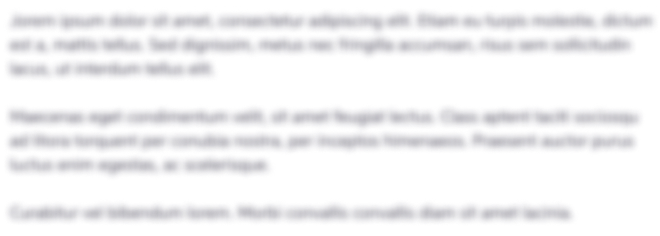
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started