Question
I am creating a game of Set with Java. It requires multiple classes/methods which I will paste below. But my two specific questions revolve around
I am creating a game of Set with Java. It requires multiple classes/methods which I will paste below. But my two specific questions revolve around the "Game" and "GameText" classes.
1. When I print the ArrayList of Selected BaordSquares (the function "list selected") it is returning to me a value such as [BoardSquare@3d4eac69] rather than the value of the card, how do I fix this to print the card?
2. I am getting an issue with the line of code in the TestSelected method BoardSquare bs2 = selected.get(1); where it says "Exception in thread "main" java.lang.IndexOutOfBoundsException: Index: 1, Size: 1" how do I fix this?
Game class
import java.util.ArrayList;
public class Game{ //intialize variable, deck and board, arraylist private int selectedCards; private Deck deck; private Board board; private ArrayList
/* Constructor creates a new game with a new shuffled deck and board, selected starts empty */ public Game(){ selectedCards = 0; deck = new Deck(); deck.shuffle(); board = new Board(deck); selected = new ArrayList
/* method outOfCards determines if the board is out of new cards @return boolean out */ public boolean outOfCards(){ boolean out; if(deck.isEmpty() == true){ out = true; } else { out = false; } return out; } /* method addToSelected takes the BoardSquare the user selects and saves it to compare later @param row @param column */ public void addToSelected(int row, int col){ //add card to an arraylist of selected selected.add(board.getBoardSquare(row, col)); selectedCards++; if (selectedCards == 3){ testSelected(); } } /* method numSelected returns the number of selected cards being held @return selectedCards */ public int numSelected(){ return selectedCards; } /* method testSelected tests whether the three cards in the selected ArrayList are a Set */ public void testSelected(){ //test if the cards from selected are a Set, method is in Card Class BoardSquare bs1 = selected.get(0); BoardSquare bs2 = selected.get(1); BoardSquare bs3 = selected.get(2); //Card.isSet(bs1.getCard(), bs2.getCard(), bs2.getCard()); if (Card.isSet(bs1.getCard(), bs2.getCard(), bs3.getCard())){ board.replaceCard(deck.getTopCard(), bs1.getRow(), bs1.getColumn()); board.replaceCard(deck.getTopCard(), bs2.getRow(), bs2.getColumn()); board.replaceCard(deck.getTopCard(), bs3.getRow(), bs3.getColumn()); //print SET System.out.print("It's a SET "); //empty ArrayList for (int i = 0; i < selected.size(); i++) { selected.remove(i); } } else{ //print NOT a SET System.out.print("Not a SET "); //empty ArrayList for (int i = 0; i < selected.size(); i++) { selected.remove(i); } } } /* method removeSelected de-selects a boardsquare from the arraylist @param row @param column */ public void removeSelected(int row, int col){ boolean status = false; if (selected.get(0).getRow() == row && selected.get(0).getColumn() == col){ selected.remove(0); selectedCards--; } else if (selected.get(1).getRow() == row && selected.get(1).getColumn() == col){ selected.remove(1); selectedCards--; } else if (selected.get(2).getRow() == row && selected.get(2).getColumn() == col){ selected.remove(2); selectedCards--; } else{ status = false; } } /* method getSelected returns the cards that have been selected @return selected */ public ArrayList
}
Game Text
import java.util.Scanner; import java.util.ArrayList;
public class GameText {
public static void main(String [] args) { // create game Game g = new Game(); // display board System.out.println(g); // connect Scanner to keyboard Scanner keyboard = new Scanner(System.in); // variables for user input int row, col; String input; // flag for ending boolean stop = false; // while the game isn't over and user doesn't want to quit while (!g.outOfCards() && !stop) { // give user their choices System.out.print("(s)elect, (u)nselect, (a)dd3, (l)ist selected, (e)nd: "); // get user choice input = keyboard.nextLine(); // act on choice // select if (input.equalsIgnoreCase("s")) { System.out.print("row col ? "); row = keyboard.nextInt(); col = keyboard.nextInt(); g.addToSelected(row,col); System.out.println(g.getSelected()); // if it's the 3rd card selected if (g.numSelected() == 3) { // is it a set? // if it is a set, the cards are replaced // if it is NOT a set, all selected cards are unselected g.testSelected(); // re-display board System.out.println(g); } } // unselect else if (input.equalsIgnoreCase("u")) { System.out.print("row col ? "); row = keyboard.nextInt(); col = keyboard.nextInt(); g.removeSelected(row,col); } // add 3 cards (1 to each row), redisplay else if (input.equalsIgnoreCase("a")) { g.add3(); System.out.println(g); } // list all cards currently selected else if (input.equalsIgnoreCase("l")) { ArrayList
}
Board
import java.util.ArrayList;
public class Board{ //initialize arraylist and variables private ArrayList
//Constructor that accepts a Deck object and populates //The ArrayLists public Board(Deck deck){ board = new ArrayList BoardSquare public class BoardSquare{ private int column;//column of the card private int row; //row of the card private Card card; private boolean selected=false; /*Constructor @param card, a card object @param column, column of the card @param row, row of the card */ public BoardSquare(Card card, int column, int row){ this.column = column; this.row = row; this.card = card; } /* method getColumn returns column @return column */ public int getColumn(){ return column; } /* method setColumn updates the column @param c */ public void setColumn(int c){ column = c; } /* method getRow returns row @return row */ public int getRow(){ return row; } /* method setRow updates the row @param r */ public void setRow(int r){ row = r; } /* method getCard returns card @return card */ public Card getCard(){ return card; } /* method setCard updates the card @param c */ public void setCard(Card c){ card = c; } /* method isSelected @returns boolean selected */ public boolean isSelected(){ return selected; } } Deck import java.util.ArrayList; import java.util.Random; public class Deck{ //initialize deck size, create ArrayList, and initialize count public final static int CARDS_IN_DECK = 81; private ArrayList for (int number=0; number<3; number++){ for (int shape=0; shape<3; shape++){ for (int shading=0; shading<3; shading++) { for (int color=0; color<3; color++) { deck.add(new Card(number, shape, shading, color)); } } } } } /* method shuffle randomizes the order of the cards */ public void shuffle() { int random; Card temp; Random r = new Random(); for (int i = 0; i < deck.size(); i++) { random = r.nextInt(deck.size()); temp = deck.get(i); deck.set(i,deck.get(random)); deck.set(random,temp); } } /* method isEmpty determines if the deck has cards in it */ public boolean isEmpty() { return (deck.size() == 0); } /* method getTopCard deals a card, returns it, and removes it from the deck @return c */ public Card getTopCard() { Card c = deck.remove(deck.size()-1); return c; } /* method toString turns the deck into a string and returns it @return str */ public String toString(){ String str="Current Deck: "; for (int i=0; i < deck.size() ; i++){ str+= deck.get(i).toString(); } return str; } } Card public class Card{ //Create enumerated values for each trait public final static int OVAL = 0; public final static int SQUARE = 1; public final static int DIAMOND = 2; public final static int RED = 0; public final static int PURPLE = 1; public final static int GREEN = 2; public final static int SOLID = 0; public final static int STRIPE = 1; public final static int OUTLINE = 2; public final static int ONE = 1; public final static int TWO = 2; public final static int THREE = 3; //Initialize traits private final int number; private final int shape; private final int color; private final int shading; //Constructor creates a card with the four characteristics public Card(int number, int shape, int shading, int color) { this.number = number; this.shape = shape; this.shading = shading; this.color = color; } /* method getNumber returns number @return number */ public int getNumber(){ return number; } /* method getShape returns shape @return shape */ public int getShape(){ return shape; } /* method getColor returns color @return color */ public int getColor(){ return color; } /* method getShading returns shading @return shading */ public int getShading(){ return shading; } /* method toString returns a string of the card characteristics @return str */ public String toString(){ String[] numbers = {"1", "2", "3"}; String[] shapes = {"Oval", "Square", "Diamond"}; String[] colors = {"Red", "Purple", "Green"}; String[] shades = {"Solid", "Striped", "Outlined"}; String str = numbers[this.number] + shapes[this.shape] + colors[this.color] + shades[this.shading]; return str; } /* method isSet returns whether or not the cards are a set @param card objects @return true or false */ public static boolean isSet(Card card1, Card card2, Card card3){ if (!((card1.number == card2.number) && (card2.number == card3.number) || (card1.number != card2.number) && (card1.number != card3.number) && (card2.number != card3.number))) { return false; } if (!((card1.shape == card2.shape) && (card2.shape == card3.shape) || (card1.shape != card2.shape) && (card1.shape != card3.shape) && (card2.shape != card3.shape))) { return false; } if (!((card1.shading == card2.shading) && (card2.shading == card3.shading) || (card1.shading != card2.shading) && (card1.shading != card3.shading) && (card2.shading != card3.shading))) { return false; } if (!((card1.color == card2.color) && (card2.color == card3.color) || (card1.color != card2.color) && (card1.color != card3.color) && (card2.color != card3.color))) { return false; } return true; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
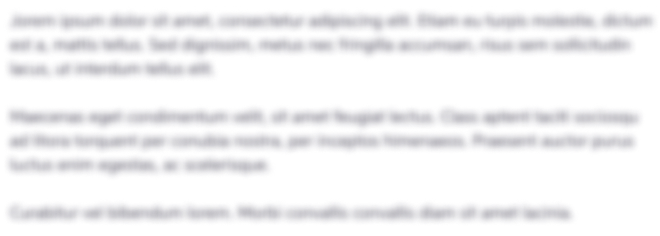
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started