Question
I am currently creating a python jupytert notebooks program that allows for people to see their paycheck rundown. I am now trying to check to
I am currently creating a python jupytert notebooks program that allows for people to see their paycheck rundown. I am now trying to check to make sure the user inputs a value that is acceptable. Like lets say I say enter(1) if married and enter(2) if not married. I want to check to make sure that the user inputs either one or two. I was just wondering if you could help me figure out how to test for equality for example is the number entered equal to 1 or 2 and if the name entered is a string?
Here is my code:
import matplotlib.pyplot as plt class employe(): def __init__(self,deductions): self.kids = 0 self.health = 0 self.money = 0 self.money2 = 0 self.medicare = 1.45 self.social = 6.3 self.mmoney = 0 self.mstax = 0 self.mftax = 0 self.htotal = 0 self.sstax = 0 self.sftax = 0 self.employename = 0 self.money3 = 0 print("If you are currently employed with our company, enter (1), if not, enter (2)?") choice = input() if choice == "1": print("Congrats, you are a proud member of our company.") self.name() elif choice =="2": print("If you worked for our company in the past year enter (1), if not, enter (2)?") choice = input() if choice == "1": print("Congrats our tax calculator can help you out.") self.name() elif choice == "2": print("Our tax calculator is unable to help you out.") exit() print("Do you have (1)Kids in daycare or (2)No Kids in daycare? ") choice = input() if choice == "1": print("You have kids in daycare") self.daycare() elif choice == "2": print("You don't have kids in daycare") print("Do you want to (1)have health care deducted before taxes or (2) not have health care deducted? ") choice = input() if choice == "1": print("You pay for healthcare") self.healthcare() elif choice == "2": print("You don't pay for healthcare") print("Do you work (1)Monthly or (2)Hourly? ") choice = input() if choice == "1": print("You work monthly") self.monthly() elif choice == "2": print("You work hourly") self.hourly() def name(self): self.employename = input("What is your name?") def daycare(self): dchild = float(input("How many kids do you have in daycare? ")) dmoney = float(input("How much do you pay per child per month? ")) dtime = float(input("How many months did your kid or kids go to daycare? ")) dtotal = dchild * dmoney * dtime self.kids = dtotal round(self.kids,2) def healthcare(self): healthnumber = float(input("How many people do you insure? ")) healthmoney = float(input("How much do you pay per person per month? ")) healthtotal = healthnumber * healthmoney * 12 self.health = healthtotal round(self.health,2) def hourly(self): hmoney = float(input("How much do you make an hour? ")) hhours = float(input("How many hours did you work in a month? ")) hmonths = float(input("How many months did you work this year? ")) self.htotal = hhours * hmoney * hmonths self.money = self.htotal - self.kids - self.health self.sstax = float(input("How much is your state tax percentage? ")) tsstax = self.sstax/100 self.sftax = float(input("How much is your federal tax percentage? ")) tsftax = self.sftax/100 ttax = self.sstax + self.sftax + self.medicare + self.social self.money4 = self.money * (1 - ttax/100) print(" ") print(" ") print(" ") print("The paycheck rundown of", self.employename, " is:") print(" ") print(" Your gross pay for the year was ", round(self.htotal,2), ".") print(" ") print(" Day care is a pre-tax expense that deducted $", self.kids,"of your gross pay.") print(" ") print(" Health care is a pre-tax expense that deducted $", self.health,"of your gross pay.") print(" ") print(" Your taxable income for the year was $", round(self.money,2), ".") print(" ") print(" Federal tax was", self.sftax,"percent of your taxable income or $", round(self.money * tsftax,2),".") print(" ") print(" State tax was", self.sstax,"percent of your taxable income or $" ,round(self.money * tsstax,2),".") print(" ") print(" Fica was", self.social ,"percent of your taxable income which is equal to $",round(self.money * .063,2), ".") print(" ") print(" Medicare was", self.medicare ,"percent of your taxable income which is equal to $", round(self.money * .0145,2), ".") print(" ") print(" You earned $" ,round(self.money4,2), "after taxes and other benefit costs")#roudn this number output_file = open("output.txt", "w") output_file.write(" ") output_file.write(" ") output_file.write(" ") input_text = "The paycheck rundown of " + str(self.employename) + " is:" output_file.write(input_text) output_file.write(" ") input_text = " Your gross pay for the year was " + str(round(self.htotal,2)) + "." output_file.write(input_text) output_file.write(" ") input_text = " Day care is a pre-tax expense that deducted $" + str(self.kids) + " of your gross pay." output_file.write(input_text) output_file.write(" ") input_text = " Health care is a pre-tax expense that deducted $" + str(self.health) +" of your gross pay." output_file.write(input_text) output_file.write(" ") input_text = " Your taxable income for the year was $" + str(round(self.money,2)) + "." output_file.write(input_text) output_file.write(" ") input_text = " Federal tax was " + str(self.sftax) + " percent of your taxable income or $" + str(round(self.money * tsftax,2)) + "." output_file.write(input_text) output_file.write(" ") input_text = " State tax was " + str(self.sstax) + " percent of your taxable income or $" + str(round(self.money * tsstax,2)) +"." output_file.write(input_text) output_file.write(" ") input_text = " Fica was " + str(self.social) + " percent of your taxable income which is equal to $" + str(round(self.money * .063,2)) + "." output_file.write(input_text) output_file.write(" ") input_text = " Medicare was " + str(self.medicare) + " percent of your taxable income which is equal to $" + str(round(self.money * .0145,2)) + "." output_file.write(input_text) output_file.write(" ") input_text =" You earned $" + str(round(self.money4,2)) + " after taxes and other benefit costs." output_file.write(input_text) output_file.close() self.pieplot2() def monthly(self): mmoney = float(input("How much money do you make a month? ")) mmonths = float(input("How many months did you work this year? ")) self.mmoney = mmonths * mmoney self.money2 = self.mmoney - self.kids - self.health self.mstax = float(input("How much is your state tax percentage? ")) tmstax = self.mstax/100 self.mftax = float(input("How much is your federal tax percentage? ")) tmftax = self.mftax/100 ttax2 = self.mstax + self.mftax + self.medicare + self.social self.money3 = self.money2 *(1 - ttax2/100) print(" ") print(" ") print(" ") print("The paycheck rundown of", self.employename, " is:") print(" ") print(" Your gross pay for the year was $", round(self.mmoney,2), ".") print(" ") print(" Day care is a pre-tax expense that deducted $", self.kids,"of your gross pay.") print(" ") print(" Health care is a pre-tax expense that deducted $", self.health,"of your gross pay.") print(" ") print(" Your taxable income for the year was $", self.money2, ".") print(" ") print(" Federal tax was", self.mftax,"percent of your taxable income or $",round(self.money2 * tmftax,2),".") print(" ") print(" State tax was", self.mstax,"percent of your taxable income or $" ,round(self.money2 * tmstax,2) ,".") print(" ") print(" Fica was", self.social ,"percent of your taxable income which is equal to $",round(self.money2 * .063,2),".") print(" ") print(" Medicare was", self.medicare ,"percent of your taxable income which is equal to $", round(self.money2 *.0145,2), ".") print(" ") print(" You earned $" ,round(self.money3,2), "after taxes and other benefit costs")#roudn this number output_file = open("output.txt", "w") output_file.write(" ") output_file.write(" ") output_file.write(" ") input_text = "The paycheck rundown of " + str(self.employename) + " is:" output_file.write(input_text) output_file.write(" ") input_text = " Your gross pay for the year was $" + str(round(self.mmoney,2)) + "." output_file.write(input_text) output_file.write(" ") input_text = " Day care is a pre-tax expense that deducted $" + str(self.kids) + " of your gross pay." output_file.write(input_text) output_file.write(" ") input_text = " Health care is a pre-tax expense that deducted $" + str(self.health) + " of your gross pay." output_file.write(input_text) output_file.write(" ") input_text = " Your taxable income for the year was $" + str(self.money2) + "." output_file.write(input_text) output_file.write(" ") input_text = " Federal tax was " + str(self.mftax) + " percent of your taxable income or $" + str(round(self.money2 * tmftax,2)) + "." output_file.write(input_text) output_file.write(" ") input_text = " State tax was " + str(self.mstax) + " percent of your taxable income or $" + str(round(self.money2 * tmstax,2)) + "." output_file.write(input_text) output_file.write(" ") input_text = " Fica was " + str(self.social) + " percent of your taxable income which is equal to $" + str(round(self.money2 * .063,2)) + "." output_file.write(input_text) output_file.write(" ") input_text = " Medicare was " + str(self.medicare) + " percent of your taxable income which is equal to $" + str(round(self.money2 *.0145,2)) + "." output_file.write(input_text) output_file.write(" ") input_text = " You earned $" + str(round(self.money3,2)) + " after taxes and other benefit costs." output_file.write(input_text) output_file.close() self.pieplot() def pieplot(self): pdaycare = (self.mmoney- self.kids) pdaycare1 = (self.mmoney - pdaycare) phealth = (self.mmoney - self.health) phealth1 = (self.mmoney - phealth) pfica = (self.money2 * .063) pmedicare = (self.money2 * .0145) pstate = (self.mstax* self.money2 /100) pfed = (self.mftax* self.money2 /100) labels = 'State Tax', 'Federal Tax', 'Fica', 'Health Insurance', 'Day Care', 'Medicare', 'Net Pay' sizes= [pstate, pfed, pfica, phealth1, pdaycare1, pmedicare, self.money3] colors = ['red', 'aqua', 'green', 'blue', 'purple', 'pink', 'gold'] patches, texts = plt.pie(sizes, colors=colors, shadow=True, startangle=90) plt.legend(patches, labels, loc="best") plt.axis('equal') plt.show() def pieplot2(self): pdaycare2 = (self.htotal- self.kids) pdaycare3 = (self.htotal-pdaycare2) phealth2 = (self.htotal - self.health) phealth3 = (self.htotal - phealth2) pfica2= (self.money * .063) pmedicare2 = (self.money * .0145) pstate2 = (self.sstax*self.money /100) pfed2 = (self.sftax*self.money /100) labels = 'State Tax', 'Federal Tax', 'Fica', 'Health Insurance', 'Day Care', 'Medicare', 'Net Pay' sizes= [pstate2, pfed2, pfica2, phealth3, pdaycare3,pmedicare2, self.money4] colors = ['red', 'aqua', 'green', 'blue', 'purple', 'pink', 'gold'] patches, texts = plt.pie(sizes, colors=colors, shadow=True, startangle=90) plt.legend(patches, labels, loc="best") plt.axis('equal') plt.show()
Bob = employe([])
Step by Step Solution
There are 3 Steps involved in it
Step: 1
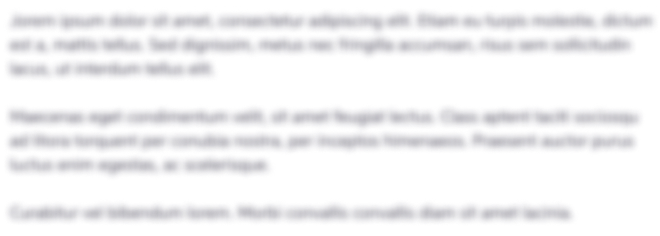
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started