Question
I am doing a project requiring an MSP430G2553. I can not get the P2.0, P2.1, and P2.2 pins to read their inputs. ANY HELP IS
I am doing a project requiring an MSP430G2553. I can not get the P2.0, P2.1, and P2.2 pins to read their inputs. ANY HELP IS APPRECIATED. THANK YOU
#include
#define CLOCK BIT6
#define OUTPUTMASK (BIT6 + BIT0)
#define INPUTMASK (BIT1 + BIT2 + BIT3)
#define INPUTMASKK (BIT0 + BIT1 + BIT2)
// Global variable used to hold the current state
//char mask;
// Global variable used to hold the number of pushes
/*
* Initialization function runs once before the state machine while(1) loop.
*/
void init(void)
{
WDTCTL = WDTPW + WDTHOLD; // Stop watchdog timer
P1DIR |= OUTPUTMASK; // Set P1.0 and P1.6 to output direction
P2DIR |= INPUTMASKK;
// P1.3 is set to input by default in the P1DIR reg
P1DIR &= ~(BIT3); // So clearing it here is redundant
// Setting the internal resistor to be a pullup
// may be redundant if P1.3 has an external pullup
P1REN |= INPUTMASK; // P1.3 enable internal resistor
P1OUT |= INPUTMASK; // P1.3 make internal resistor pullup
P2OUT |= INPUTMASKK;
P2IN |= (BIT0 + BIT1 + BIT2);
P1OUT &= ~(OUTPUTMASK); // Set display initially to zero
// Using a mask so that only the desired bits are affect
P2IN |= ~(BIT0 + BIT1 + BIT2);
}
/*
* readInput - This function reads the status of the enable button
* and returns its value
* (S2 on the Launchpad connected to Port 1 Bit 3)
*
* returns 0 if the button is not pressed
* returns 1 if the button is pressed
*
* It also waits for the button to be released so that only one
* button push is registered
*/
long int readInput(void)
{
char series = 0;
// The P1IN registers holds the logical values of the pins that are
// configured as inputs
if (!(P1IN & BIT1)) { // Check for S2 input
// pushes = pushes + 1; // Code that executes when S2 button is pushed
series = 1;
__delay_cycles(10000); // Wait for debounce to end (bad code)
while(!(P1IN & BIT1)) {
// Wait for Up button to return to "normal"
// Must re-read the input port
// Not good code because loop could run forever
__delay_cycles(10000); // Again wait for debounce to end (again bad code)
}}
else if(!(P1IN & BIT2)) { // Check for S2 input
// pushes = pushes + 1; // Code that executes when S2 button is pushed
series = 2;
__delay_cycles(10000); // Wait for debounce to end (bad code)
while(!(P1IN & BIT2)) {
// Wait for Up button to return to "normal"
// Must re-read the input port
// Not good code because loop could run forever
__delay_cycles(10000); // Again wait for debounce to end (again bad code)
}}
else if(!(P1IN & BIT3)) { // Check for S2 input
// pushes = pushes + 1; // Code that executes when S2 button is pushed
series = 7;
__delay_cycles(10000); // Wait for debounce to end (bad code)
while(!(P1IN & BIT3)) {
// Wait for Up button to return to "normal"
// Must re-read the input port
// Not good code because loop could run forever
__delay_cycles(10000); // Again wait for debounce to end (again bad code)
}}
else if(!(P2IN & BIT0)) { // Check for S2 input
// pushes = pushes + 1; // Code that executes when S2 button is pushed
series = 4;
__delay_cycles(10000); // Wait for debounce to end (bad code)
while(!(P2IN & BIT0)) {
// Wait for Up button to return to "normal"
// Must re-read the input port
// Not good code because loop could run forever
__delay_cycles(10000); // Again wait for debounce to end (again bad code)
}}
else if(!(P2IN & BIT1)) { // Check for S2 input
// pushes = pushes + 1; // Code that executes when S2 button is pushed
series = 5;
__delay_cycles(10000); // Wait for debounce to end (bad code)
while(!(P2IN & BIT1)) {
// Wait for Up button to return to "normal"
// Must re-read the input port
// Not good code because loop could run forever
__delay_cycles(10000); // Again wait for debounce to end (again bad code)
}}
else if(!(P2IN & BIT2)) { // Check for S2 input
// pushes = pushes + 1; // Code that executes when S2 button is pushed
series = 3;
__delay_cycles(10000); // Wait for debounce to end (bad code)
while(!(P2IN & BIT2)) {
// Wait for Up button to return to "normal"
// Must re-read the input port
// Not good code because loop could run forever
__delay_cycles(10000); // Again wait for debounce to end (again bad code)
}}
return series;
}
void ClockData(void)
{
P1OUT |= CLOCK; // Assert P1.6 (rising clock edge)
__delay_cycles(1); // Delay
P1OUT &= ~CLOCK; // De-assert P1.6 (falling clock)
}
/*void blink(mask)
{
SerialDataOut(0);
}*/
// Shift out the value of DataOut to the connected shift register
void SerialDataOut(long int DataOut)
{
char i, lsb; // i = for loop index
// lsb = Value of the Least Significant Bit of DataOut
for(i=0; i<=23; i++)
{
lsb = DataOut & 0x01; // Set lsb to right most bit of DataOut
// Place the LSB of DataOut on P1.0 and therefore to the input of the shift register
if (lsb)
{
P1OUT |= 0x01; // Assert P1.0 if the LSB of DataOut is 1
}
else
{
P1OUT &= ~0x01; // De-assert P1.0 if the LSB of DataOut is 0
}
//__delay_cycles(100); // Delay
// Clock the Data to the Shift Register
ClockData();
// Shift data left and repeat the for loop
DataOut >>= 0x01;
}
}
/*
* main.c
*/
long int main(void)
{
char enable = 0; // Holds status of the S2 button
// char enableclock = 0;
init(); // One time initialization function
long int light = 1;
long int mask = 0;
/*
* The main while loop of the state machine will constantly scan the inputs
* and update the system based on those inputs
*/
while(1) {
// Clear Data
SerialDataOut(light^mask);
//1`SerialDataOut(mask);
__delay_cycles(80000);
SerialDataOut(mask);
__delay_cycles(80000);
enable = readInput(); // Check the S2 button
/*
* The decision making in the state machine can be made with
* a switch statement or nested if statements
* Both implementations are given below. Only one should be enabled at a time
* Simply make one and only one of the #if statements #if 1.
*/
/*#if 1
switch(enable) {
case 0 :
// Do nothing if the button is not pressed
break;
case 1 :
// If the button is pressed then allow the counter to increment once
countUp();
__delay_cycles(800000);
break;
}
#endif*/
//#if 0
switch(enable) {
case 0 :
// Do nothing if the button is not pressed
break;
case 1 :
if ((light==8)||(light==128)||(light==2048)||(light==32768)||(light==0x80000)||(light==0x800000)||(light==0x8000000)||(light==0x80000000)){
}
else {
light=light<<1;}
__delay_cycles(80000);
break;
case 2 :
if ((light==1)||(light==16)||(light==256)||(light==4096)||(light==0x10000)||(light==0x100000)||(light==0x1000000)||(light==0x10000000)){
}
else {
light=light>>1;}
__delay_cycles(80000);
break;
case 3 : if ((light==0x100000)||(light==0x200000)||(light==0x400000)||(light==0x800000)) {
light=light>>20;
}
else {
light=light<<4;
}
__delay_cycles(80000);
break;
case 4 : if ((light==1)||(light==2)||(light==4)||(light==8)) {
light=light<<20;
}
else {
light=light>>4;}
__delay_cycles(80000);
break;
case 5 :
mask=light^mask;
__delay_cycles(80000);
break;
case 7 :
if(light=light){
light=0;
}else{
light=1;
}
__delay_cycles(80000);
break;
}
} // end while loop
} // end main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
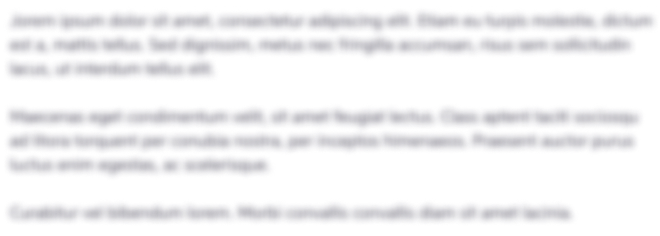
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started