Question
I am having a lot of difficulties designing a test for a singleton. I can't turn it into an array. Any recommendations for improvements to
I am having a lot of difficulties designing a test for a singleton. I can't turn it into an array. Any recommendations for improvements to the test design?
Class Being Tested
package medical.com.medicalApplication.services;
import java.util.ArrayList; import java.util.Collections; import java.util.List;
import medical.com.medicalApplication.model.MedicalRecord; import medical.com.medicalApplication.model.Patient; import medical.com.medicalApplication.model.PatientHistory; /** * * This class uses a singleton pattern to mock a service instead of using dependency injection * * In addition, it stores data in memory only using Lists * */ //FIXME: Spelling Error. MedicalRescordService -> MedicalRecordService public class MedicalRecordService { //FIXME: Spelling Error. MedicalRescordService -> MedicalRecordService private static MedicalRecordService reference = new MedicalRecordService(); private List
//FIXME: Spelling Error MedicalRescordService -> MedicalRecordService public static MedicalRecordService getReference() { return reference; }
//FIXME: Spelling Error MedicalRescordService -> MedicalRecordService MedicalRecordService() { this.patients = new ArrayList
public boolean addPatient(String name, String id) { boolean patientAdded = !patients.stream() .anyMatch(patient -> patient.getId().equals(id)); if (patientAdded) { Patient newPatient = new Patient(name, id); //FIXME: Add newHistory variable PatientHistory newHistory = new PatientHistory(); patients.add(newPatient); //FIXME: Add newHistory variable medicalRecords.add(new MedicalRecord(newPatient, newHistory)); } return patientAdded; } public MedicalRecord getMedicalRecord(String patientId) { return medicalRecords.stream() .filter(medicalRecord -> medicalRecord.getPatient().getId().equals(patientId)).findFirst().get(); }
public Patient getPatient(String patientId) { return patients.stream().filter(person -> person.getId().equals(patientId)) .findFirst().get(); }
public List
//1 of 2 branches missed if(getMedicalRecord(patient.getId()).getHistory().getAllergies().stream().filter(allergy -> allergy.getName().equals(allergyName)) != null){ return Collections.singletonList(patient); } } return Collections.emptyList(); //Code not covered by testing } }
Current Test Design
package medical.com.medicalApplication.services.tests;
import static org.junit.Assert.*;
import java.util.List;
import org.junit.After; import org.junit.Before; import org.junit.Test;
import medical.com.medicalApplication.services.MedicalRecordService; import medical.com.medicalApplication.model.Allergy; import medical.com.medicalApplication.model.MedicalRecord; import medical.com.medicalApplication.model.Patient;
public class TestMedicalRecordService {
private MedicalRecordService service; private List
record.getHistory().addAllergy(allergy); List
Step by Step Solution
There are 3 Steps involved in it
Step: 1
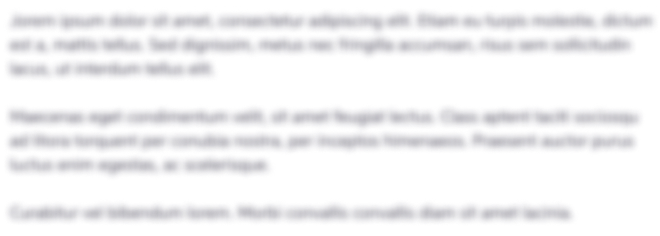
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started