Question
I am having trouble with the following problems in this Java code: 1. I am unable to get menu item #3 to display the objects
I am having trouble with the following problems in this Java code:
1. I am unable to get menu item #3 to display the objects in the ArrayList ListOfRecipes
2. I am unable to elicit user input on the chosen recipe because it always defaults to the first recipe instead of the one created by menu item #1 (create new recipe)
3. For menu item #4, adjust recipe servings, I need to get the ingredients and the ingredient amount to adjust the ingredient amount per serving but am unable to get that to display to calculate the new servings, ingredient, adjusted ingredient amount.
Here is the code:
package recipebox;
import java.util.Scanner; import java.util.ArrayList; import java.util.Iterator;
/** * * @author davidamonahan */ public class Ingredient { private double servings; private String ingredientName; private double ingredientAmount; private double ingredientCalories; private double totalRecipeCalories = (ingredientAmount * ingredientCalories);
public Ingredient(String ingredientName, double ingredientAmount, double ingredientCalories) { this.ingredientName = ingredientName; this.ingredientAmount = ingredientAmount; this.ingredientCalories = ingredientCalories; }
public String getIngredientName() { return ingredientName; }
public void setIngredientName(String ingredientName) { this.ingredientName = ingredientName; }
public double getIngredientAmount() { return ingredientAmount; }
public void setIngredientAmount(double ingredientAmount) { this.ingredientAmount = ingredientAmount; }
public double getIngredientCalories() { return ingredientCalories; }
public void setIngredientCalories(double ingredientCalories) { this.ingredientCalories = ingredientCalories; }
public double getTotalRecipeCalories() { return totalRecipeCalories; }
public void setTotalRecipeCalories(double totalRecipeCalories) { this.totalRecipeCalories = totalRecipeCalories; } }
public class RecipeBox {
private static String recipeName;
private double totalRecipeCalories;
private ArrayList recipeIngredients;
private static ArrayList listOfRecipes;
private double servings;
private double ingredientAmount;
private double ingredientAmountPerServing;
private String unitMeasurement;
/** * * @param recipeName * @param totalRecipeCalories * @param recipeIngredients * @param listOfRecipes * @param servings * @param ingredientAmount * @param ingredientAmountPerServing * @param unitMeasurement */ public RecipeBox(String recipeName, double totalRecipeCalories, ArrayList recipeIngredients, ArrayList listOfRecipes, double servings, double ingredientAmount, double ingredientAmountPerServing, String unitMeasurement) { this.recipeName = recipeName; this.totalRecipeCalories = totalRecipeCalories; this.recipeIngredients = recipeIngredients; this.listOfRecipes = listOfRecipes; this.servings = servings; this.ingredientAmount = ingredientAmount; this.ingredientAmountPerServing = ingredientAmountPerServing; this.unitMeasurement = unitMeasurement; }
/** * */ public RecipeBox() { this.listOfRecipes = new ArrayList<>(); }
/** * * @param index * @param element */ /** * * @param index * @return */ public ArrayList getListOfRecipes() { return listOfRecipes; }
/** * * @param listOfRecipes */ public void setListOfRecipes(ArrayList listOfRecipes) {
this.listOfRecipes = listOfRecipes; }
/** * * * @param listOfRecipes */ public RecipeBox(ArrayList listOfRecipes) { this.listOfRecipes = listOfRecipes; }
/** * */ public void printAllRecipeDetails() {
getListOfRecipes().stream().forEach((_recipeName) -> { printRecipe(); }); }
/** * * @return recipe name, ingredients, servings, total calories, per serving */ public double getIngredientAmount() { return ingredientAmount; }
/** * @return the ingredientAmountPerServing */ public double getIngredientAmountPerServing() { return ingredientAmountPerServing; }
/** * @param ingredientAmountPerServing the ingredientAmountPerServing to set */ public void setIngredientAmountPerServing(double ingredientAmountPerServing) { this.ingredientAmountPerServing = ingredientAmountPerServing; }
/** * @return the unitMeasurement */ public String getUnitMeasurement() { return unitMeasurement; }
/** * @param unitMeasurement the unitMeasurement to set */ public void setUnitMeasurement(String unitMeasurement) { this.unitMeasurement = unitMeasurement; }
/** * * @param recipeName * @param servings * @param recipeIngredients * @param totalRecipeCalories */ public RecipeBox(String recipeName, double servings, ArrayList recipeIngredients, double ingredientAmount, double totalRecipeCalories) { // for final project add ingredient amount per serving this.recipeName = recipeName;
this.servings = servings;
this.recipeIngredients = recipeIngredients;
this.totalRecipeCalories = totalRecipeCalories;
this.ingredientAmount = ingredientAmount;
this.ingredientAmountPerServing = ingredientAmountPerServing; }
/** * * @return */ public String getRecipeName() {
return recipeName;
}
/** * * @param recipeName */ public void setRecipeName(String recipeName) {
this.recipeName = recipeName;
}
/** * * @return */ public double getTotalRecipeCalories() {
return totalRecipeCalories;
}
/** * * @param totalRecipeCalories */ public void setTotalRecipeCalories(double totalRecipeCalories) {
this.totalRecipeCalories = totalRecipeCalories;
}
/** * * @return */ public ArrayList getRecipeIngredients() {
return recipeIngredients;
}
/** * * @param recipeIngredients */ public void setRecipeIngredients(ArrayList recipeIngredients) {
this.recipeIngredients = recipeIngredients;
}
/** * * @return */ public double getServings() {
return servings;
}
/** * * @param servings */ public void setServings(double servings) {
this.servings = servings;
}
/** * * @return */ public double getingredientAmount() {
return getingredientAmount(); }
/** * * @param ingredientAmount */ public void setIngredientAmount(double ingredientAmount) {
this.ingredientAmount = ingredientAmount; }
/** * */ public void printRecipeName() {
//To change body of generated methods, choose Tools | Templates. }
/** * */ public void printAllRecipeNames() {
for (UltimateRecipeBuilder currentRecipe : listOfRecipes) { System.out.println(currentRecipe.getRecipeName());
} }
/** * */ public void printRecipe() { // print method to display recipe
double singleServingCalories = (double) (getTotalRecipeCalories() / getServings());
String unitMeasurement = "";
System.out.println("Recipe: " + getRecipeName());
System.out.println("Serves: " + getServings());
getRecipeIngredients().stream().forEach((ingredient) -> { System.out.println(ingredient); //+ " Use " + getIngredientAmountPerServing() this part is under //+ getUnitMeasurement() + " per serving"); }); //FIX ME- need to attach ingredient amount to each ingredient in array!
System.out.println("Each serving has " + singleServingCalories + " Calories.");
} public void addNewRecipe() { listOfRecipes.add(new UltimateRecipeBuilder().createNewRecipe()); }
/** * * @param args * @return */ @SuppressWarnings("unchecked") public static void main(String[] args) { RecipeBox recipe = createNewRecipe();
recipe.printRecipe();
RecipeBox myRecipeBox = new RecipeBox(); ArrayList listOfRecipes = new ArrayList();
Scanner menuScnr = new Scanner(System.in);
System.out.println("Please select a menu item: " + "1. Add Recipe " + "2. Print Recipe " + "3. Print Recipe Names " + "4. Adjust Recipe Servings " + "5. Delete Recipe "); while (menuScnr.hasNextInt() || menuScnr.hasNextLine()) {
System.out.println("Please select a menu item: " + "1. Add Recipe " + "2. Print Recipe " + "3. Print Recipe Names " + "4. Adjust Recipe Servings " + "5. Delete Recipe "); int input = menuScnr.nextInt();
if (input == 1) {
myRecipeBox.createNewRecipe(); listOfRecipes.add(recipeName); recipe.printRecipe(); // FIX ME! the only thing this changes is recipeName, the ingredients from the // first recipe are still there! System.out.println("Please select a menu item: " + "1. Add Recipe " + "2. Print Recipe " + "3. Print Recipe Names " + "4. Adjust Recipe Servings " + "5. Delete Recipe ");
} else if (input == 2) {
System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
recipe.printRecipe(); System.out.println("Please select a menu item: " + "1. Add Recipe " + "2. Print Recipe " + "3. Print Recipe Names " + "4. Adjust Recipe Servings " + "5. Delete Recipe ");
} else if (input == 3) { for (int j = 0; j < myRecipeBox.listOfRecipes.size(); j++) { System.out.println((j + 1) + ": " + myRecipeBox.listOfRecipes.get(j).getRecipeName()); // this will NOT give me any recipes/recipeNames! System.out.println("Please select a menu item: " + "1. Add Recipe " + "2. Print Recipe " + "3. Print Recipe Names " + "4. Adjust Recipe Servings " + "5. Delete Recipe "); }
} else if (input == 4){ System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
listOfRecipes.stream().forEach((UltimateRecipeBuilder_recipeName) -> { }); recipe.printRecipe(); System.out.println ("How many servings would you like?"); while (menuScnr.hasNextInt() || menuScnr.hasNextLine()) { input = menuScnr.nextInt(); //FIX ME, do not know how to get this because unable to attach ingredient amount //to ingredient }
} else if (input == 5){ System.out.println("Which recipe? "); String selectedRecipeName = menuScnr.next();
listOfRecipes.stream().forEach((UltimateRecipeBuilder_recipeName) -> { }); // FIX ME need to display new list of recipes or confirm that it was removed but // unable because CAN"T display original list! listOfRecipes.remove(recipeName); System.out.println("Please select a menu item: " + "1. Add Recipe " + "2. Print Recipe " + "3. Print Recipe Names " + "4. Adjust Recipe Servings " + "5. Delete Recipe "); } } }
/** * * @return */ public static RecipeBox createNewRecipe() {
Scanner scnr = new Scanner(System.in);
ArrayList recipeIngredients = new ArrayList(); // lists recipe ingredients ArrayList listOfRecipes = new ArrayList(); // lists recipes double totalRecipeCalories = 0; // total of calories from all ingredients double ingredientCalories = 0; // # calories per each double ingredientAmount = 0; // amount of ingredient defined by unit of measure (cup, tsp, etc.) double servings = 0; // # servings per recipe boolean addMoreIngredients = true;
System.out.println("Please enter the recipe name: "); String recipeName = scnr.nextLine(); // gets name of recipe
while (!recipeName.matches("[a-zA-Z_]+")) { System.out.println("Invalid name, use letters only");
recipeName = scnr.nextLine(); // validates user input is letters, repeats statement until letters entered }
listOfRecipes.add(recipeName);
System.out.println("Please enter the number of servings: "); // gets # servings
while (!scnr.hasNextDouble()) { // verifies input is pos # System.out.println("Please enter the number of servings" + ". Use numbers only "); // validates user inputs numbers for servings, repeats until numbers entered scnr.nextLine();
} servings = scnr.nextDouble();
scnr.nextLine();
do{ System.out.println("Please enter an ingredient name using letters only. Enter 'e' if you are finished"); // gets first ingredient String ingredientName = scnr.next(); if (ingredientName.toLowerCase().equals("e")) { // breaks loop of adding more ingredients, goes to print recipe addMoreIngredients = false; // allow to add more ingredients } else{ while (!ingredientName.matches(".*[a-z].*")) { System.out.println("Please enter an ingredient name. Use letters only"); ingredientName = scnr.next(); // validates user input is letters, repeats until letters entered } recipeIngredients.add(ingredientName); // adds ingredient to array list System.out.println("Please enter the unit of measure for the" + " ingredient (ex.- cup, oz, tsp, tbsp, pt). Use only" + " letters, no numbers: "); // gets unit of measurement for ingredient (cup, oz, tsp. etc.) String unitMeasurement = scnr.nextLine(); while (!unitMeasurement.matches( ".*[a-z].*")) { //validates input is letters System.out.println("Please enter the unit of measure for the" + " ingredient (ex.- cup, oz, tsp, tbsp, pt). Use only" + " letters, no numbers: "); //validates user input is letters, repeats until letters entered unitMeasurement = scnr.nextLine(); } System.out.println("How many " + unitMeasurement + "s of " + ingredientName + "?. Enter numbers only!"); // gets ingredient amount while (!scnr.hasNextDouble()) { System.out.println("How many " + unitMeasurement + "s of " + ingredientName + "?. Enter numbers only!"); // validates user input is numbers for amount, repeats until numbers entered scnr.nextLine(); } ingredientAmount = scnr.nextDouble(); System.out.println("Please enter the number of calories per " + unitMeasurement + ". Use numbers only!"); // gets # calories per amount of ingredient while (!scnr.hasNextDouble()) { System.out.println("Please enter the number of calories per " + unitMeasurement + ". Use numbers only!"); // validates user input # calories is numbers, repeats until number entered scnr.nextLine(); } ingredientCalories = scnr.nextDouble(); scnr.nextLine(); totalRecipeCalories += (ingredientCalories * ingredientAmount); }
}while(addMoreIngredients);
listOfRecipes.add(recipeName);
RecipeBox recipe = new RecipeBox(recipeName, servings, recipeIngredients, ingredientAmount, totalRecipeCalories); // creates recipe- name, # servings, ingredients, total calories, calories per serving
return recipe; // prints recipe } }
Here are two additional classes
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package recipebox;
import java.util.ArrayList;
/** * * @author davidamonahan */ class UltimateRecipeBuilder { static Object listOfRecipes; static double servings; static ArrayList
String getRecipeName() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
void printRecipe() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
UltimateRecipeBuilder createNewRecipe() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } }
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package recipebox;
/** * * @author davidamonahan */ class UltimateRecipeBuilder {
boolean getRecipeName() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
boolean getListOfRecipes() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
UltimateRecipeBuilder createNewRecipe() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } }
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package recipebox;
/** * * @author davidamonahan */ class UltimateRecipeBuilder {
boolean getRecipeName() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
boolean getListOfRecipes() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
UltimateRecipeBuilder createNewRecipe() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
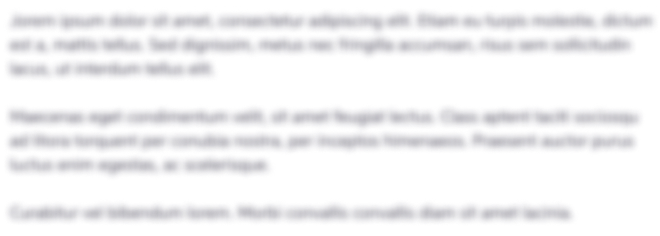
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started