Question
I am reposting my previous question and clarifying it more. I have a python 3 lab with 6 steps, I will provide pictures of the
I am reposting my previous question and clarifying it more. I have a python 3 lab with 6 steps, I will provide pictures of the 6 different steps. The main task of this lab is to make a grocery list editor with undo stack. There are 7 .py files of code. Some of these .pys needed to be edited in order to complete the 6 steps to make this editor with undo stack.
Here is 2 images of the lab steps below.
Here is the full code from the main.py:
import sys from GroceryList import GroceryList
def main(): grocery_list = GroceryList()
quit = False while not quit: command = input()
# Process user input if command == "print": grocery_list.print_list(sys.stdout) elif 0 == command.find("add "): grocery_list.add_with_undo(command[4:]) elif 0 == command.find("removeat "): grocery_list.remove_at_with_undo(int(command[9:])) elif 0 == command.find("swap "): index1, index2 = parse_indices(command[5:]) grocery_list.swap_with_undo(index1, index2) if index2
def parse_indices(str): indices = [int(n) for n in str.split()] if len(indices) != 2: return -1, -1 else: return indices
if __name__ == '__main__': main()
Here is the code from the UndoCommand.py:
from abc import ABC, abstractmethod class UndoCommand(ABC): @abstractmethod def execute(self): pass
Here is the code from the RemoveLastCommand.py:
from UndoCommand import UndoCommand
class InsertAtCommand(UndoCommand): def __init__(self, source, index, new_item): super().__init__() self.source_list = source self.index = index self.new_item = new_item
def execute(self): self.source_list.insert(self.index, self.new_item)
def undo(self): self.source_list.pop(self.index)
Here is the code from the SwapCommand.py:
from UndoCommand import UndoCommand
class SwapCommand(UndoCommand): def __init__(self, source, index1, index2): # Type your code here. pass
def execute(self): # Type your code here. pass
Here is the code form the InsertAtCommand.py:
from Stack import Stack from UndoCommand import UndoCommand
class InsertAtCommand(UndoCommand): def __init__(self, source, index, new_item): # Type your code here. pass
def execute(self): # Type your code here. pass
Here is the code from the GroceryList.py:
from Stack import Stack from InsertAtCommand import InsertAtCommand from RemoveLastCommand import RemoveLastCommand from SwapCommand import SwapCommand
class GroceryList: def __init__(self): self.list_items = [] self.undo_stack = Stack()
def add_with_undo(self, new_item_name): # Add the list item self.list_items.append(new_item_name)
# Make an undo command that removes the last item and pushes it onto the undo stack self.undo_stack.push(RemoveLastCommand(self.list_items))
def remove_at_with_undo(self, removal_index): # Type your code here. pass
def swap_with_undo(self, index1, index2): # Type your code here. pass
def execute_undo(self): # Type your code here. pass
def get_list_size(self): return len(self.list_items)
def get_undo_stack_size(self): return self.undo_stack.size()
def get_list_copy(self): return self.list_items[:]
def print_list(self, outfil): for n, item in enumerate(self.list_items): print(f"""{n}. {item}""", file=outfil)
Here is the code from the Stack.py:
# Simple list implementation of Stack
class Stack: def __init__(self): self.stack_list = []
def size(self): return len(self.stack_list)
def pop(self): return self.stack_list.pop()
def push(self, item): self.stack_list.append(item)
def top(self): return self.stack_list[-1]
def items(self): return self.stack_list
Which .py code(s) need to be edited in order to complete steps 1-6? Thank you for the assistance.
4.26 LAB: Grocery list editor with undo stack In this lab a grocery list editor with undo functionality is implemented. Step 1: Inspect the UndoCommand abstract base class The read-only UndoCommand.py file has a declaration for the UndoCommand abstract base class. Access UndoCommand.py by clicking on the orange arrow next to main.py at the top of the coding window. The UndoCommand class represents a command object: an object that stores all needed information to execute an action at a later point in time. For this lab, a command object stores information to undo a grocery list change made by the user. Step 2: Inspect the incomplete GroceryList class The GroceryList class is declared in GroceryList.py. Two attributes are declared: - A list of strings for list items - A stack of UndoCommand references for undo commands A simple list implementation of stack ADT is provided in Stack.py. Note that the add_with_undo 0 method is already implemented. The method adds a new item to the list and pushes a new RemoveLastCommand object onto the undo stack. Step 3: Implement RemoveLastCommand's execute() method The RemoveLastCommand class inherits from UndoCommand and is declared in RemoveLastCommand.py. When a RemoveLastCommand object is executed, the string list's last element is removed. So when the user appends a new item to the grocery list, a RemoveLastCommand is pushed onto the stack of undo commands. Popping and executing the RemoveLastCommand then removes the item most recently added by the user. RemoveLastCommand's source_list attribute and constructor are already declared: - source_list is a reference to a GroceryList object's list of strings. - The constructor takes a reference to a list of strings as a parameter, and assigns source_list with the reference. Implement RemoveLastCommand's execute( ) method to remove source_list's last element. Step 4: Implement GroceryList's execute_undo() method Implement GroceryList's execute_undo() method to do the following: - Pop an UndoCommand off the undo stack - Execute the popped undo command File main.py has code that reads in a list of commands, one per line, that allow for basic testing of basic operations. So after implementing execute_undo(), run your program with the following input: add bananas add grapes add strawberries print undo print undo print quit Verify that the corresponding output is: Verify that the corresponding output is: 0 . bananas 1. grapes 2. strawberries 0. bananas 1. grapes 0. bananas The program's output does not affect grading, so additional test cases can be added, if desired. Submitting code written so far to obtain partial credit is recommended before proceeding to the next step. Step 5: Implement the SwapCommand class and GroceryList's swap_with_undo() method Implement the SwapCommand class in SwapCommand.py. The class itself is declared, but no attributes yet exist. Add necessary attributes and methods so that the command can undo swapping two items in the grocery list. Implement GroceryList's swap_with_undo() method. The method swaps list items at the specified indices, then pushes a SwapCommand, to undo that swap, onto the undo stack. Step 6: Implement the InsertAtCommand class and GroceryList's remove_at_with_undo() method Implement the InsertAtCommand class in InsertAtCommand.py. Add necessary fields and methods so that the command can undo removing a grocery list item at an arbitrary index. Implement GroceryList's remove_at_with_undon method. The method removes the list item at the specified index, then pushes an InsertAtCommand, to undo that removal, onto the undo stack. \begin{tabular}{l|l} LAB & 4.26.1: LAB: Grocery list editor with undo stack \end{tabular} 0/10 Downloadable files , and File is marked as read only Current file: main.pyStep by Step Solution
There are 3 Steps involved in it
Step: 1
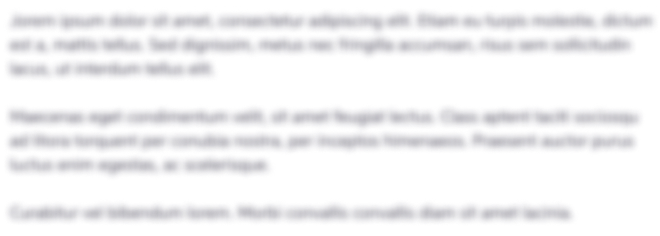
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started