Question
I am sort of stuck on this drawing program! Ive tried my best to create my handlers but I cant figure out how to implement
I am sort of stuck on this drawing program! Ive tried my best to create my handlers but I cant figure out how to implement MouseHandler ColorHandler. or Any help is greatly appreciated thank you!
The GUI should two Combo Boxes, one to select a color, and one to select a thickness of lines. It should also have two buttons, one is to undo (erase) the last drawn line, and one is to erase all lines.
A user can choose a color using a combo box. Until the color is changed, any line will be drawn with that color. The default color is black.
A user can choose a width of line strokes using a combo box. Until the width is changed, any line will be drawn with that width. The default color is 1.
A user can move a mouse into the drawing area and press the mouse button, and this point will be the starting point of a line. A user can drag a mouse, and one should see a line from the pressed point to the point where a user is dragging. Once a user releases the mouse button. that line between the pressed point and released point should be drawn on the drawing pane permanently. A user can again presses, drags and releases the mouse to draw another line with a choice of color and size at that time.
If a user presses the Undo button, then the last drawn line should disappear.
If a user pressed the Erase button, all drawn lines should disappear.
The DrawingPane class organizes all components in the GUI. It should contain a Pane (drawing area) at the bottom, and another pane containing two combo boxes, one for colors, one for stroke width, and undo button and erase button. Your pane needs to have the same appearance as the shown picture.
This is where all components are arranged. Add as many instance variables as you like to this class, and initialize them in this constructor. Then they need to be arranged so that the GUI will have the required appearance in this constructor. Objects of the Handlers are also instantiated here and are used to set for their corresponding component(s).public DrawingPane()
MouseHandler class (defined as a nested class of DrawingPane class)
This method should work with the canvas pane. If the mouse button is pressed, and is dragged, then it should show a temporary line using the pressed point and the dragging point (thus while the mouse is dragged, this line will keep moving since the dragged point is moving.) When the mouse button is released, a permanent line using the pressed point and the released point will be drawn. If a user pressed and released the mouse multiple times, then multiple lines will be drawn. Each line should be drawn using the selected color and the selected stroke with at that time.
ButtonHandler class (defined as a nested class of DrawingPane class
This method should work with the undoButton and eraseButton. If the undoButton is pushed, then it should delete the last drawn line, and if the eraseButton is pushed, then it should erase all drawn lines.
ColorHandler class (defined as a nested class of DrawingPane class)
This method is executed in case the color combo box is used to select a color. It should record a choice of the color so that lines can be drawn using it.
WidthHandler class (defined as a nested class of DrawingPane class)
This method is executed in case the width combo box is used to select a width of line strokes. It should record a choice of the width so that lines can be drawn using it.
How to get started:
Download the following files and use them as a base of your program: Assignment7.java DrawingPane.java
Assignment7.java:
import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.layout.StackPane;
public class Assignment7 extends Application { public void start(Stage primaryStage) { //create a DrawPane object. See DrawPane.java for details. DrawingPane gui = new DrawingPane(); //put gui on top of the rootPane StackPane rootPane = new StackPane(); rootPane.getChildren().add(gui); // Create a scene and place rootPane in the stage Scene scene = new Scene(rootPane, 600, 400); primaryStage.setTitle("Line Drawing"); primaryStage.setScene(scene); // Place the scene in the stage primaryStage.show(); // Display the stage } public static void main(String[] args) { Application.launch(args); } }
DrawingPane.Java:
//import any classes necessary here //---- import javafx.scene.control.Button; import javafx.scene.control.ComboBox; import javafx.scene.layout.Pane; import javafx.scene.layout.BorderPane; import javafx.scene.layout.HBox; import javafx.scene.shape.Line; import javafx.scene.paint.Color; import javafx.geometry.Insets; import javafx.geometry.Pos; import javafx.geometry.Orientation; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.input.MouseEvent;
import java.util.ArrayList; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.scene.control.Label;
public class DrawingPane extends BorderPane { private Button undoButton, eraseButton; private ComboBox
public DrawingPane() { //Step #1: initialize instance variable and set up layout undoButton = new Button("Undo"); eraseButton = new Button("Erase"); undoButton.setMinWidth(80.0); eraseButton.setMinWidth(80.0);
//Create the color comboBox and width comboBox, ObservableList
//Step #3: Register the source nodes with its handler objects canvas.setOnMousePressed(new MouseHandler()); undoButton.setOnAction(new ButtonHandler()); // registering undo button with ButtonHandler eraseButton.setOnAction(new ButtonHandler()); // registering erase button with ButtonHandler }
//Step #2(A) - MouseHandler private class MouseHandler implements EventHandler
public void handle(MouseEvent event) { //handle MouseEvent here //Note: you can use if(event.getEventType()== MouseEvent.MOUSE_PRESSED) //to check whether the mouse key is pressed, dragged or released //write your own codes here //----
if (event.getEventType() == MouseEvent.MOUSE_PRESSED){ firstX = event.getX(); firstY = event.getY();
line.setStartX(firstX); line.setStartY(firstY);
} if (event.getEventType() == MouseEvent.MOUSE_DRAGGED) { double deltaX = event.getX(); double deltaY = event.getY();
line.setEndX(deltaX); line.setEndY(deltaY); } if (event.getEventType() == MouseEvent.MOUSE_RELEASED) { endX = event.getX(); endY = event.getY();
line.setEndX(endX); line.setEndY(endY); } lineList.add(line); canvas.getChildren().add(line); }//end handle() }//end MouseHandler
//Step #2(C)- A handler class used to handle colors private class ColorHandler implements EventHandler
Step by Step Solution
There are 3 Steps involved in it
Step: 1
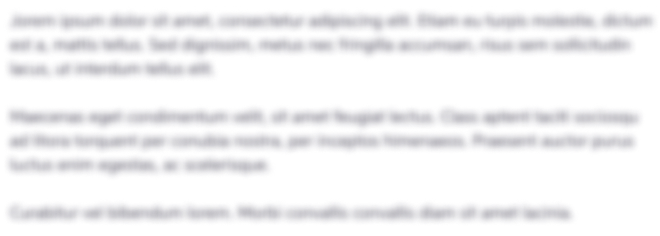
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started