Question
I am trying to add these to my program. can you help with that. Thanks. 1) Store all entries in the phonebook into a location/file-name
I am trying to add these to my program. can you help with that. Thanks.
1) Store all entries in the phonebook into a location/file-name specified by the user. 2) Retrieve entries from the location/file-name specified by the user.
#include
#include
#include
#include
//Function prototypes
void Add();
void Delete();
void Display();
void Alphabetical();
void Number();
void Random();
void DeleteAll();
//Structure for Phonebook list
typedef struct PhoneBookList {
char *FirstName;
char *LastName;
char *PhoneNumber;
} list;
//Structure for delete
typedef struct DeleteEntry {
char *FirstName;
char *LastName;
} take;
//Pointers to structures
list *lt;
take *tk;
//Global variables
int count = 0;
int delCount = 0;
int main(void)
{
//Variable declarations
int iSelection;
do { //Print menu to screen, ask user for selection
printf(" \t\t\tPhone Book: ");
printf("\t1) Add contact ");
printf("\t2) Delete contact ");
printf("\t3) Display phone book ");
printf("\t4) Sort contact alphabetically by last name ");
printf("\t5) Find phone number for given name ");
printf("\t6) Randomly select contact ");
printf("\t7) Delete all contacts ");
printf("\t8) Exit ");
printf("\tWhat do you want to do? ");
scanf("%d", &iSelection);
switch (iSelection) {
case 1: //Add entry
Add();
break;
case 2: //Delete entry
Delete();
break;
case 3: //Display all entries
Display();
break;
case 4: //Sort entries alphabetically
Alphabetical();
break;
case 5: //Find phone number
Number();
break;
case 6: //Randomly select contact
Random();
break;
case 7: //Delete all contacts
DeleteAll();
break;
case 8: //Break loop and close program
break;
default: //User entered an invalid number
printf(" \t\tYou entered an invalid selection. Try again. ");
break;
} //End Switch
} while (iSelection != 8); //End Do While loop
//Return used memory
free(tk);
free(lt);
tk = NULL;
lt = NULL;
return 0;
} //End Main
//Add an entry
void Add()
{
char *leftName;
if (count == 0)
{
lt = (list *) malloc ((count*25) + 25);
}
else
{
lt = (list *) realloc (lt, (count*50) + 50);
}
if (lt == NULL)
{
printf("You cannot add more memory ");
}
else
{
lt[count].FirstName = (char *) malloc(sizeof(char)*15);
lt[count].LastName = (char *) malloc(sizeof(char)*15);
lt[count].PhoneNumber = (char *) malloc(sizeof(char)*15);
printf(" \tEnter their First Name: ");
scanf("%s", lt[count].FirstName);
printf(" \tEnter their Last Name: ");
scanf("%s", lt[count].LastName);
printf(" \tEnter their Phone Number: ");
scanf("%s", lt[count].PhoneNumber);
printf(" \t\t\tContact added! ");
}
count++;
}//End Function
//Delete an entry
void Delete()
{
int i;
int q = 0;
char *userName;
if (delCount == 0)
{
tk = (take *) malloc ((delCount*25) + 25);
}
else
{
tk = (take *) realloc (tk, (delCount*1) + 1);
}
if (tk == NULL)
{
printf("This cannot be deleted (out of memory) ");
}
else
{
tk[delCount].FirstName = (char *) malloc(sizeof(char)*15);
tk[delCount].LastName = (char *) malloc(sizeof(char)*15);
printf(" \tEnter their First Name: ");
scanf("%s", tk[delCount].FirstName);
printf(" \tEnter their Last Name: ");
scanf("%s", tk[delCount].LastName);
}
for (i = 0; i < count; i++)
{
if (lt[i].FirstName == NULL && lt[i].LastName == NULL) continue;
if (strcmp(lt[i].FirstName, tk[delCount].FirstName) == 0 && strcmp(lt[i].LastName, tk[delCount].LastName) == 0)
{
printf(" \t\t\t%s %s has been deleted! ", lt[i].FirstName, lt[i].LastName);
lt[i].FirstName = NULL;
lt[i].LastName = NULL;
lt[i].PhoneNumber = NULL;
q = 1;
break;
}
} //End for loop
if (q != 1)
{
printf(" \tThis person is not in the Phonebook ");
}
delCount++;
count--;
}//End function
//Display all phonebook entries
void Display()
{
int i;
printf(" \tYour contacts: ");
for (i = 0; i < count; i++)
{
if (lt[i].FirstName != NULL && lt[i].LastName != NULL)
{
printf(" \t\t%s %s: %s ", lt[i].FirstName, lt[i].LastName, lt[i].PhoneNumber);
}
}//End for loop
system("pause");
}//End function
//Sort entries alphabetically by last name.
void Alphabetical()
{
int i;
int j;
char temp[50][50];
printf(" \tYour contacts: ");
for (i = 0; i < count; i++)
{
for (j = i + 1; j < count; j++)
{
if (strcmp(lt[i].LastName, lt[j].LastName) > 0)
{
strcpy(temp[i], lt[i].LastName);
strcpy(lt[i].LastName, lt[j].LastName);
strcpy(lt[j].LastName, temp[i]);
}
}
}//End for loop
for (i = 0; i < count; i++)
printf(" \t\t%s %s: %s ", lt[i].FirstName, lt[i].LastName, lt[i].PhoneNumber);
system("pause");
}//End function
//Find phone number for given name
void Number()
{
int i;
int q = 0;
char fName[25];
char lName[25];
printf(" \tEnter their First Name: ");
scanf("%s", fName);
printf(" \tEnter their Last Name: ");
scanf("%s", lName);
for (i = 0; i < count; i++)
{
if (strcmp(lt[i].FirstName, fName) == 0 && strcmp(lt[i].LastName, lName) == 0)
{
printf(" \t\t%s %s's phone number is: %s ", lt[i].FirstName, lt[i].LastName, lt[i].PhoneNumber);
q = 1;
break;
}
}
if (q != 1)
{
printf(" This person is not in the Phonebook ");
}
system("pause");
}//End function
//Randomly select contact
void Random()
{
srand(time(NULL));
int iRandomNum;
iRandomNum = (rand() % count) + 1;
printf("\t\t%s %s: %s ", lt[iRandomNum].FirstName, lt[iRandomNum].LastName, lt[iRandomNum].PhoneNumber);
system("pause");
}//End function
//Delete everyone from phone book
void DeleteAll()
{
int i;
for (i = 0; i < count; i++)
{
do{
lt[i].FirstName = NULL;
lt[i].LastName = NULL;
lt[i].PhoneNumber = NULL;
break;
} while (i <= count);
}
printf(" \t\tAll contacts deleted ");
system("pause");
}//End function
Step by Step Solution
There are 3 Steps involved in it
Step: 1
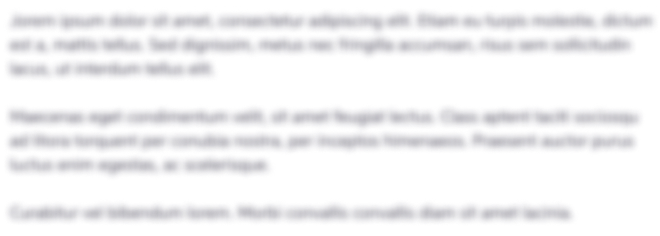
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started