Question
I am trying to use an if statement to display the ratings of provided movies with one star * to five stars *****. I have
I am trying to use an if statement to display the ratings of provided movies with one star * to five stars *****. I have the code running but I cannot figure out how to make it work with an if statement. Also needs to make the user try again if they do not enter strictly *, **, ***, ****, or ***** for the rating. No stars or anything more than 5 or the program will ask the same question, inform the user to only use 1-5 stars, and to try again without crashing.
This first picture is just the explanation.
This second picture is just more explanation.
I will attach a copy of my code below.
using System; using System.Collections.Generic;// for our movie lists using System.IO; // used for File.ReadAllText using System.Text.Json;// used for Json
namespace ConsoleApp4 { class Program {
static void Main(string[] args) { MultipleJson(); SingleJson(); }
static void MultipleJson() { // open a bunch of JSON files and save to a list
string jsonDirectory = "JSON-Movies"; // the JSON folder in the same folder as the EXE
string[] jsonFiles = Directory.GetFiles(jsonDirectory);
// initializing variables for the loop /// list of a bunch of "Movie" objects, to be pulled from the jsonFiles array List
// placeholder movie object Movie oneMovie = new Movie();
string jsonData = ""; // placeholder for the raw JSON data read from the file
// copying all the JSON data into the list of objects // one JSON file per element in the list foreach (var jsonFile in jsonFiles) { try { jsonData = File.ReadAllText(jsonFile); // extracts all the raw text from the JSON file (all of the lines) and saves it into a string
oneMovie = JsonSerializer.Deserialize
movieList.Add(oneMovie); // adding the oneCar object to the list of Movies } catch (Exception ex) { Console.WriteLine("Erron: invalid JSON file (" + jsonFile + ")"); }
} // EX: printing out all of the movies in the list Console.WriteLine("You have these movies:"); Console.WriteLine(); foreach (var aMovie in movieList) { Console.WriteLine(aMovie.title + " " + aMovie.year + " "); } Console.WriteLine("Please use '*'(s) to rate the movies one at a time: ");// These WriteLine's are being used to explain the directions to the user Console.WriteLine("Using one star rating (*), to five stars rating (*****). ");// Also explaining the rating system. Console.WriteLine("Start with your rating for The Wizard of Oz. ");
}
static void SingleJson() { string jsonDirectory = "JSON-Movies"; // the JSON folder in the same folder as the EXE
string[] jsonFiles = Directory.GetFiles(jsonDirectory);
// Reading (deserealizing) the 1st JSON file string jsonfiles = "tt0032138.json";// declares the name and location of the JSON file (same folder as the exe) string jsonmoviedata1 = File.ReadAllText(jsonfiles);// copies ALL of the text in the JSON file to a string.
Movie movie1 = JsonSerializer.Deserialize
//Write/Update (serializing) a JSON file movie1.id = "tt0032138"; //updating the property in memory
movie1.rating = Console.ReadLine(); Console.WriteLine("You rated The Wizard of Oz " + movie1.rating); Console.WriteLine();
//Parsing the movie1 object, converting all of the properties into a single raw JSON formatted string (serializing) jsonmoviedata1 = JsonSerializer.Serialize(movie1); //OPTIONAL: use pretty printing, or indented text, for the JSON output file var jsonOptions = new JsonSerializerOptions { WriteIndented = true }; jsonmoviedata1 = JsonSerializer.Serialize(movie1, jsonOptions);
StreamWriter movie1writer = new StreamWriter(jsonfiles);//creates a new streamwriter object to write to the jsonmovieFile1 file.
movie1writer.Write(jsonmoviedata1);//writes the valid JSON string saved in the jsonmoviedata1 variable to the jsonmovieFile1 file.
movie1writer.Close(); //closes the file to make Professor Grohs :)
// Reading (deserealizing) the 2nd JSON file string jsonmovieFile2 = "tt0034583.json";// declares the name and location of the JSON file (same folder as the exe) string jsonmoviedata2 = File.ReadAllText(jsonmovieFile2);// copies ALL of the text in the JSON file to a string.
Movie movie2 = JsonSerializer.Deserialize
//Write/Update (serializing) a JSON file movie2.id = "tt0034583"; //updating the property in memory Console.WriteLine("Now, your rating for Casablanca "); Console.WriteLine(); movie2.rating = Console.ReadLine(); Console.WriteLine("You rated Casablanca " + movie2.rating); Console.WriteLine();
//Parsing the movie1 object, converting all of the properties into a single raw JSON formatted string (serializing) jsonmoviedata2 = JsonSerializer.Serialize(movie2); //OPTIONAL: use pretty printing, or indented text, for the JSON output file var jsonOptions2 = new JsonSerializerOptions { WriteIndented = true }; jsonmoviedata2 = JsonSerializer.Serialize(movie2, jsonOptions2);
StreamWriter movie2writer = new StreamWriter(jsonmovieFile2);//creates a new streamwriter object to write to the jsonmovieFile1 file.
movie2writer.Write(jsonmoviedata2);//writes the valid JSON string saved in the jsonmoviedata1 variable to the jsonmovieFile1 file.
movie2writer.Close(); //closes the file to make Professor Grohs :)
// Reading (deserealizing) the 3rd JSON file string jsonmovieFile3 = "tt0059742.json";// declares the name and location of the JSON file (same folder as the exe) string jsonmoviedata3 = File.ReadAllText(jsonmovieFile3);// copies ALL of the text in the JSON file to a string.
Movie movie3 = JsonSerializer.Deserialize
//Write/Update (serializing) a JSON file movie3.id = "tt0059742"; //updating the property in memory Console.WriteLine("Now, your rating for The Sound of Music "); movie3.rating = Console.ReadLine(); Console.WriteLine("You rated The Sound of Music " + movie3.rating); Console.WriteLine();
//Parsing the movie3 object, converting all of the properties into a single raw JSON formatted string (serializing) jsonmoviedata3 = JsonSerializer.Serialize(movie3); //OPTIONAL: use pretty printing, or indented text, for the JSON output file var jsonOptions3 = new JsonSerializerOptions { WriteIndented = true }; jsonmoviedata3 = JsonSerializer.Serialize(movie3, jsonOptions3);
StreamWriter movie3writer = new StreamWriter(jsonmovieFile3);//creates a new streamwriter object to write to the jsonmovieFile1 file.
movie3writer.Write(jsonmoviedata3);//writes the valid JSON string saved in the jsonmoviedata1 variable to the jsonmovieFile1 file.
movie3writer.Close(); //closes the file to make Professor Grohs :)
// Reading (deserealizing) the 4th JSON file string jsonmovieFile4 = "tt0071853.json";// declares the name and location of the JSON file (same folder as the exe) string jsonmoviedata4 = File.ReadAllText(jsonmovieFile4);// copies ALL of the text in the JSON file to a string.
Movie movie4 = JsonSerializer.Deserialize
//Write/Update (serializing) a JSON file movie4.id = "tt0071853"; //updating the property in memory Console.WriteLine("Now, your rating for Monty Python and the Holy Grail "); movie4.rating = Console.ReadLine();
Console.WriteLine("You rated Monty Python and the Holy Grail " + movie4.rating); Console.WriteLine();
//Parsing the movie4 object, converting all of the properties into a single raw JSON formatted string (serializing) jsonmoviedata4 = JsonSerializer.Serialize(movie4); //OPTIONAL: use pretty printing, or indented text, for the JSON output file var jsonOptions4 = new JsonSerializerOptions { WriteIndented = true }; jsonmoviedata4 = JsonSerializer.Serialize(movie4, jsonOptions4);
StreamWriter movie4writer = new StreamWriter(jsonmovieFile4);//creates a new streamwriter object to write to the jsonmovieFile1 file.
movie4writer.Write(jsonmoviedata4);//writes the valid JSON string saved in the jsonmoviedata1 variable to the jsonmovieFile1 file.
movie4writer.Close(); //closes the file to make Professor Grohs :) }
}
class Movie { public string id { get; set; } public string title { get; set; } public string year { get; set; } public string runtime { get; set; } public string genre { get; set; } public string rating { get; set; } } }
Console assignment 4: No valid files found in the JSON folder An invalid file could not be deserialized into an object o output the name of the invalid file to the console skip that file and continue The user enters something other than *, **, *******, or for a rating o hint: use an if statement o repeat until the user types in a valid entry Attempted to write to a JSON file and failed o output the id of the object that failed to the console o skip that file and continue For each exception or condition, output a custom error message to the console. The standard catch (Exception ex) { } clause is allowed for all exceptions. Multiple or specialized catch clauses are not required. Create a C# Console .NET project using Visual Studio 2019 that opens a folder of movie data saved as JSON files and edits the data. You program will deserialize the JSON files as objects, save them into an array/list of objects, prompt the user to update a property, then serialize that updated data and save it back into the JSON files. Note: Be sure to select "Console App (.NET Core) [C#]" as your project template. "Console App (.NET Framework) [C#]" does not allow JSON file processing. Click here to download a list of JSON files with movie data. You are free to add additional JSON files to this list or take some away. Use a minimum of four files for testing. The JSON is formatted with the following fields (all strings): id: IMDb movie ID title: the movie's title year: the release year runtime: how long the movie is, in minutes genre: what kind of story it is rating: scale from * (one star) to ***** (five stars) how well you personally like the movie, blank for all movies When your program runs, automatically get the names of the JSON files in a folder, deserialize their contents into objects of a custom class, and save them into an array/list, as shown in Console Lecture 4-2 video. Next, display the title and year of each movie to the console, one at at time, and ask the user to enter their rating from * (one star) to ***** (five stars). Save that input into the rating property of the object. Repeat for each file in the array/list. Finally, save the updated movie data to the original JSON files (serialize). The JSON files should be updated with the correct data (don't mix them up). Use one custom class that corresponds 1:1 with the JSON file format. Console assignment 4: No valid files found in the JSON folder An invalid file could not be deserialized into an object o output the name of the invalid file to the console skip that file and continue The user enters something other than *, **, *******, or for a rating o hint: use an if statement o repeat until the user types in a valid entry Attempted to write to a JSON file and failed o output the id of the object that failed to the console o skip that file and continue For each exception or condition, output a custom error message to the console. The standard catch (Exception ex) { } clause is allowed for all exceptions. Multiple or specialized catch clauses are not required. Create a C# Console .NET project using Visual Studio 2019 that opens a folder of movie data saved as JSON files and edits the data. You program will deserialize the JSON files as objects, save them into an array/list of objects, prompt the user to update a property, then serialize that updated data and save it back into the JSON files. Note: Be sure to select "Console App (.NET Core) [C#]" as your project template. "Console App (.NET Framework) [C#]" does not allow JSON file processing. Click here to download a list of JSON files with movie data. You are free to add additional JSON files to this list or take some away. Use a minimum of four files for testing. The JSON is formatted with the following fields (all strings): id: IMDb movie ID title: the movie's title year: the release year runtime: how long the movie is, in minutes genre: what kind of story it is rating: scale from * (one star) to ***** (five stars) how well you personally like the movie, blank for all movies When your program runs, automatically get the names of the JSON files in a folder, deserialize their contents into objects of a custom class, and save them into an array/list, as shown in Console Lecture 4-2 video. Next, display the title and year of each movie to the console, one at at time, and ask the user to enter their rating from * (one star) to ***** (five stars). Save that input into the rating property of the object. Repeat for each file in the array/list. Finally, save the updated movie data to the original JSON files (serialize). The JSON files should be updated with the correct data (don't mix them up). Use one custom class that corresponds 1:1 with the JSON file format
Step by Step Solution
There are 3 Steps involved in it
Step: 1
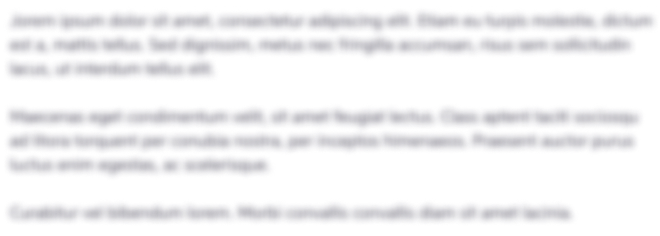
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started