Question
I am working on a Java project building a Car class and accompanying constructors and methods... However, I've been instructed to add more components and
I am working on a Java project building a Car class and accompanying constructors and methods... However, I've been instructed to add more components and need help with where and how to add them to the existing code...
This is the existing code:
____________________________________________________________________________________________________________
import java.text.DecimalFormat;
public class Car
{ public void main (String [] args){} private String make; private String model; private int y; private double mpg; private double mileageNextOilChange; private double odometer; private double gasGauge; private static final double TANK_CAPACITY = 12.5; private static final int MILES_BETWEEN_OIL_CHANGE = 5000; private static DecimalFormat df = new DecimalFormat("###,##0.00");
public Car() { make = "Ford"; model = "Explorer"; y = 2020; mpg = 29.0; gasGauge = TANK_CAPACITY; odometer = 0.0; mileageNextOilChange = MILES_BETWEEN_OIL_CHANGE; }
public Car (String make, String model, int y, double mpg) { this.make = make; this.model = model; this.y = y; this.mpg = mpg; gasGauge = TANK_CAPACITY; odometer = 0.0; mileageNextOilChange = MILES_BETWEEN_OIL_CHANGE; }
public String getMake() { return make; }
public String getModel() { return model; }
public int getYear() { return y; }
public double getMpg() { return mpg; }
public double getMileageNextOilChange() { return mileageNextOilChange; }
public double checkOdometer() { return odometer; }
public double checkGasGauge() { return gasGauge; }
public void setMake(String make) { this.make = make; }
public void setModel(String model) { this.model = model; }
public void setYear(int y) { this.y = y; }
public void setMpg(double value) { this.mpg = value; }
public void honkHorn() { System.out.println("Beep Beep!"); }
public void addGas (double gallons) { if(gallons < 0) { System.out.println(make + " " + model + " " + y + " gallons cannot be a negative number - Gas in Tank after the fill up: " + df.format(gasGauge)); } else if((gasGauge + gallons) > TANK_CAPACITY) { gasGauge = TANK_CAPACITY; System.out.println(make + " " + model + " " + y + " tank overflowed - Gas in Tank after the fill up: " + df.format(gasGauge)); } else { gasGauge += gallons; System.out.println(make + " " + model + " " + y + " added gas: " + gallons + " - Gas in Tank after the fill up: " + df.format(gasGauge)); } }
public void drive (double miles) { if(miles < 0) { System.out.println(make + " " + model + " " + y + " cannot drive negative miles."); } else { double gasRequired = miles / mpg; if(gasRequired > gasGauge) { double distance = gasGauge * mpg; gasGauge = 0.0; odometer += distance;
System.out.println(make + " " + model + " " + y + " ran out of gas after driving " + df.format(distance) + " miles."); } else { gasGauge -= gasRequired; odometer += miles; System.out.println(make + " " + model + " " + y + " drove " + df.format(miles) + " miles."); } } }
public void changeOil ( ) { mileageNextOilChange = odometer + MILES_BETWEEN_OIL_CHANGE; System.out.println(make + " " + model + " " + y + " oil changed, next mileage to change oil is: " + df.format(mileageNextOilChange)); }
public void checkOil ( ) { if(odometer >= mileageNextOilChange) { System.out.println(make + " " + model + " " + y + " - It is time to change the oil."); } else { System.out.println(make + " " + model + " " + y + " - Oil is OK, no need to change."); } }
public String toString ( ) { return (make + " " + model + " " + y + " Odometer: " + df.format(odometer) + " Gas in Tank: " + df.format(gasGauge)); }
public boolean equals (Car other) { return this.make == other.getMake() && this.model == other.getModel() && this.y == other.getYear(); } }
________________________________________________________________________________________________________________________
This is the additional methods I have been asked to add. My issue is that I don't know where to add them into the current coding or how to test them to be sure they work.
Challenge Requirements
- Add a boolean instance variable that monitors if the engine is on or off.
- Create new methods:
- public void startEngine( ) set the boolean variable to the appropriate value and print a message
Example:
Nissan Pathfinder 2015 engine started.
- public void stopEngine( ) set the boolean variable to the appropriate value and print a message
Example:
Nissan Pathfinder 2015 engine stopped.
-
- public boolean isEngineOn( ) return true if the engine is on, returns false otherwise.
- Modify existing methods with a warning message if the car is, or is not, running and do not perform the requested action when appropriate. For example, the car must be running to drive. The car must be turned off to add gas, check oil, or change the oil.
Examples:
- Ford Explorer 2020 must be ON to drive.
- Ford Explorer 2020 must be OFF to add gas.
More Methods to add
- private double calcRange() - calculate the total range (maximum miles to drive) based on the gas in the tank and the mpg of the car.
- private double calcGasNeededToFillTank() - calculate the gas needed to fill the tank.
- public void simulateMultiCityTrip (int numberCities ) Use a loop to simulate a person doing a trip to visit a number of cities, entered as input parameter. You must invoke the drive and addGas methods.
Inside of the loop you need to do the following:
- invoke the calcRange()method to figure out the maximum miles you can drive with the gas that you have in the tank
- generate an integer random number to simulate the number of miles needed to drive to get to the next city. This random number should be an integer number between 1 and the maximum mileage (inclusive). Make sure to cast the maximum range to an int before using it as parameter
- invoke the method to start the engine
- invoke the drive method and use as the input parameter the random number generated on the previous step
- invoke the method to stop the engine
- invoke the addGas method. Invoke the calcGasNeededToFillTank()method to figure out the gas needed to fill the tank and pass this value as input parameter to the addGas method.I
I also need to provide code to test and prove my methods work.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
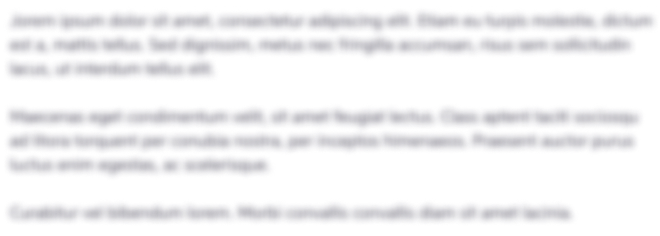
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started