Question
I am writing minesweeper game but can't make it work for chapter 8 ex 15 of my C++ book on visual studio code . Can
I am writing minesweeper game but can't make it work for chapter 8 ex 15 of my C++ book on visual studio code . Can Someone Help me with that?
#include
#include
#include
using namespace std; // This line can be removed and replaced with individual "std::"s for every cout, cin, and endl
// however for the purpose of this program it didn't really seem necesarry for me to do this here.
const int DIMX = 6, DIMY = 6;
const int MINE = -1, MINES = 6;
const char COVERED = 'X', UNCOVERED = ' ', FLAG = 'F';
int highscore = 999999; // Highscore = How fast the game was completed.
int state[DIMX][DIMY];
char display[DIMX][DIMY];
string name = "Anonymous";
string highscore_name = "Anonymous";
int start_s = 0;
void init(); // Initialize game states
int countSurroundingMines(int x, int y); // Helper function used by init
void reveal(int x, int y);
void player(); // Handles player input
void placeFlag();
void uncover();
void print(); // Displays the minefield
bool isWin();
bool isLose();
bool playNewGame();
int main()
{
cout << "Welcome to MineSweeper! ";
init();
bool playing = true;
while (playing)
{
print();
player();
if (isWin())
{
cout << " Congratulations!! " << name << " you Win!" << endl;
int stop_s = clock();
double time = (stop_s - start_s) / double(CLOCKS_PER_SEC); // Seconds
if (time < highscore)
{
cout << "You set a new score with the time: " << time << "!" << endl;
time = highscore;
name = highscore_name;
}
else
{
cout << "Your time was: " << time << " seconds! ";
cout << "Unfortunately that is longer than " << highscore_name << "'s score highscore of: " << highscore << endl;
}
playing = false;
}
else if (isLose())
{
cout << " I'm sorry, " << name << " you have hit a mine and lost." << endl;
int stop_s = clock();
double time = (stop_s - start_s) / double(CLOCKS_PER_SEC); // Seconds
cout << "Your time was: " << time << " seconds! ";
playing = false;
}
if (!playing)
{
playing = playNewGame();
if (playing)
{init();}
}
}
cout << "Exiting Minesweeper!" << endl;
return 0;
}
void init()
{
cout << " New Game! " << endl;
cout << "Please enter your name: ";
cin >> name;
start_s = clock();
// Set display to "uncovered"
for (int y = 0; y < DIMY; y++)
{
for (int x = 0; x < DIMX; x++)
{display[x][y] = COVERED;}
}
// Initialize mines
srand(time(0));
for (int i = 0; i < MINES; i++)
{
bool placed = false;
while (!placed)
{
int x = rand() % DIMX;
int y = rand() % DIMY;
if (state[x][y] != MINE) // Check if mine is not set
{
placed = true;
state[x][y] = MINE;
}
}
}
// Place the hint numbers
for (int y = 0; y < DIMY; y++)
{
for (int x = 0; x < DIMX; x++)
{state[x][y] = countSurroundingMines(x, y);}
}
}
bool playNewGame()
{
bool selected = false;
while (!selected)
{
char choice;
cout << "Play another game? Y or N" << endl;
cin >> choice;
if (choice == 'N' || choice == 'n') {
return false;
selected = true;
} else if (choice == 'Y' || choice == 'y') {
return true;
selected = true;
} else {
cout << "Error: Invalid choice selection. Please try again." << endl; playNewGame();
}
}
}
void reveal(int x, int y)
{
if (x >= 0 && x < DIMX && y >= 0 && y < DIMY) // Checks that x & y are valid
{
if (display[x][y] == UNCOVERED)
{return;}
display[x][y] = UNCOVERED;
if (state[x][y] == MINE || state[x][y] != 0)
{return;}
reveal(x - 1, y - 1);
reveal(x, y - 1);
reveal(x + 1, y - 1);
reveal(x - 1, y);
reveal(x + 1, y);
reveal(x - 1, y + 1);
reveal(x, y + 1);
reveal(x + 1, y + 1);
}
else { return; }
}
/*
[x-1, y-1] [x, y-1] [x+1, y-1]
[x-1, y ] [x, y ] [x+1, y ]
[x-1, y+1] [x, y+1] [x+1, y+1]
*/
int countSurroundingMines(int x, int y)
{
if (state[x][y] == MINE)
{
return MINE;
}
int num = 0;
if (x > 0 && y > 0) { if (state[x - 1][y - 1] == MINE) { num++; } } // Top Left
if (y > 0) { if (state[x][y - 1] == MINE) { num++; } } // Top
if (x < DIMX - 1 && y > 0) { if (state[x + 1][y - 1] == MINE) { num++; } } // Top Right
if (x > 0) { if (state[x - 1][y] == MINE) { num++; } } // Left
if (x < DIMX - 1) { if (state[x + 1][y] == MINE) { num++; } } // Right
if (y < DIMY - 1) { if (state[x][y + 1] == MINE) { num++; } } // Bottom
if (x > 0 && y < DIMY - 1) { if (state[x - 1][y + 1] == MINE) { num++; } } // Bottom Left
if (y < DIMY - 1) { if (state[x][y + 1] == MINE) { num++; } } // Bottom
if (x < DIMX - 1 && y < DIMY - 1) { if (state[x + 1][y + 1] == MINE) { num++; } } // Bottom Right
return num;
}
bool isWin()
{
bool win = true;
for (int y = 0; y < DIMY; y++)
{
for (int x = 0; x < DIMX; x++)
{
win &= ((display[x][y] == UNCOVERED && state[x][y] != MINE) ||
((display[x][y] == COVERED || display[x][y] == FLAG) && state[x][y] == MINE));
}
}
return win;
}
bool isLose()
{
for (int y = 0; y < DIMY; y++)
{
for (int x = 0; x < DIMX; x++)
{
if (display[x][y] == UNCOVERED && state[x][y] == MINE)
{return true;}
}
}
return false;
}
void player()
{
cout << " Command list: ";
cout << " Enter 'S' to Sweep a coordinate" << endl;
cout << " Enter 'F' to place a flag" << endl;
cout << " Enter 'N' to start a new game" << endl;
cout << " Enter 'Q' to quit program." << endl;
cout << endl;
bool selected = false;
while (!selected)
{
char choice;
cin >> choice;
if (choice == 'S' || choice == 's')
{
uncover();
selected = true;
} else if (choice == 'F' || choice == 'f') {
placeFlag();
selected = true;
} else if (choice == 'N' || choice == 'n') {
init();
selected = true;
} else if (choice == 'Q' || choice == 'q') {
exit(EXIT_SUCCESS);
} else {
cout << "Error: Choice not in command list, please try again." << endl;
player();
}
}
}
void uncover()
{
bool selected = false;
while (!selected)
{
int x;
int y;
cout << "Input X (1 - " << (DIMX) << ")" << endl;
cin >> x;
cout << "Input Y (1 - " << (DIMY) << ")" << endl;
cin >> y;
x--;
y--;
if (x >= 0 && x < DIMX && y >= 0 && y < DIMY)
{
reveal(x, y); // Call recursive reveal algorithm
selected = true;
}
else { cout << "(X,Y) Values out of range!" << endl; }
}
}
void placeFlag()
{
bool selected = false;
while (!selected)
{
int x;
int y;
cout << "Input X (1 - " << (DIMX) << ")" << endl;
cin >> x;
cout << "Input Y (1 - " << (DIMY) << ")" << endl;
cin >> y;
x--;
y--;
if (x >= 0 && x < DIMX && y >= 0 && y < DIMY)
{
display[x][y] = FLAG;
selected = true;
}
else
{cout << "(X,Y) Values out of range!" << endl;}
}
}
void print()
{
cout << " ";
for (int x = 0; x < DIMX; x++)
{cout << (x + 1) << " ";}
cout << endl << "";
for (int x = 0; x < DIMX; x++)
{cout << "";}
cout << endl;
for (int y = 0; y < DIMY; y++)
{
cout << (y + 1) << " ";
for (int x = 0; x < DIMX; x++)
{
if (display[x][y] == COVERED || display[x][y] == 'F')
{cout << display[x][y] << " ";}
else // Display the state
{
if (state[x][y] == MINE)
{cout << "@ ";}
else
{
if (state[x][y] == 0)
{cout << " ";}
else
{cout << state[x][y] << " ";}
}
}
}
cout << endl;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
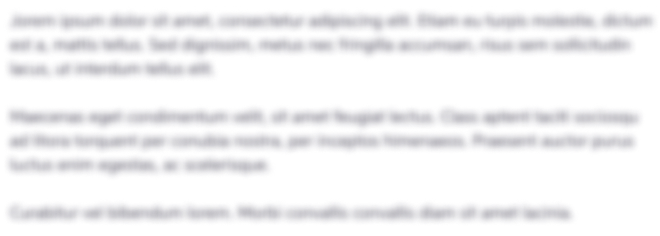
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started