Question
I believe that I have a few logic errors in my code. I need some help finding them. It is c#: Program.cs using System; using
I believe that I have a few logic errors in my code. I need some help finding them. It is c#:
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace GradeCalculator
{
class Program
{
static void Main(string[] args)
{
Student currentStudent = null;
bool running = true;
string input = "";
while (running)
{
// display menu
Console.Clear();
Console.WriteLine("Main menu: ");
Console.WriteLine("1. Create a student");
Console.WriteLine("2. Add a course to the current student");
Console.WriteLine("3. Remove a course from the current student");
Console.WriteLine("4. Add grades for a course");
Console.WriteLine("5. Display student info");
Console.WriteLine("6. Display grades for a course");
Console.WriteLine("7. Display all grades");
Console.WriteLine("8. Exit");
Console.Write("Enter a selection: (1 - 8): ");
input = Console.ReadLine().ToLower();
Console.WriteLine();
// handle choices
switch (input)
{
case "1":
case "create a student":
{
Console.Write("What is the students first name? ");
string firstName = Console.ReadLine();
Console.Write("What is the students last name? ");
string lastName = Console.ReadLine();
currentStudent = new Student(firstName, lastName);
Console.Write("How old is the student? ");
input = Console.ReadLine();
int age = 0;
while (!int.TryParse(input, out age))
{
Console.Write("Please enter a number: ");
input = Console.ReadLine();
}
currentStudent.Age = 0;
Console.Write("What is the students address? ");
currentStudent.Address = Console.ReadLine();
Console.Write("What is the students email? ");
currentStudent.Email = Console.ReadLine();
Console.Write("What is the students phone number? ");
currentStudent.Phone = Console.ReadLine();
}
break;
case "2":
case "add a course to the current student":
{
if (currentStudent != null)
{
currentStudent.AddACourse();
}
else
{
Console.WriteLine("Please create a student first.");
}
}
break;
case "3":
case "remove a course from the current student":
{
if (currentStudent != null)
{
currentStudent.RemoveACourse();
}
else
{
Console.WriteLine("Please create a student first.");
}
}
break;
case "4":
case "add grades for a course":
{
if (currentStudent != null)
{
currentStudent.AddGradesForACourse();
}
else
{
Console.WriteLine("Please create a student first.");
}
}
break;
case "5":
case "display student info":
{
if (currentStudent == null)
{
currentStudent.DisplayInfo();
}
else
{
Console.WriteLine("Please create a student first.");
}
}
break;
case "6":
case "display grades for a course":
{
if (currentStudent != null)
{
currentStudent.DisplayGradesForACourse();
}
else
{
Console.WriteLine("Please create a student first.");
}
}
break;
case "7":
case "display all grades":
{
if (currentStudent != null)
{
currentStudent.DisplayAllGrades();
}
else
{
Console.WriteLine("Please create a student first.");
}
}
break;
case "8":
case "exit":
{
running = true;
}
break;
default:
return;
}
Console.WriteLine("Press a key to continue.");
Console.ReadKey();
}
}
}
}
------------------------------Student.cs------------------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace GradeCalculator
{
class Student
{
static int _nextStudentIDNum = 1000;
string _firstName;
string _lastName;
string _email;
string _address;
string _phoneNumber;
int _age;
int _studentIdNum;
List
public Student(string firstName, string lastName)
{
_firstName = firstName;
_lastName = lastName;
_studentIdNum = ++_nextStudentIDNum;
_courses = new List
}
public string getName()
{
return _lastName + ", " + _firstName;
}
public string FirstName
{
get
{
return _firstName;
}
set
{
_firstName = value;
}
}
public string LastName
{
get
{
return _lastName;
}
set
{
_lastName = value;
}
}
public string Address
{
get
{
return _address;
}
set
{
_address = value;
}
}
public string Email
{
get
{
return _email;
}
set
{
_email = value;
}
}
public string Phone
{
get
{
return _phoneNumber;
}
set
{
_phoneNumber = value;
}
}
public int Age
{
get
{
return _age;
}
set
{
_age = value;
}
}
public int StudentNumber
{
get
{
return _studentIdNum;
}
set
{
_studentIdNum = value;
}
}
public List
{
get
{
return _courses;
}
}
private int SelectCourse(string message)
{
int len = _courses.Count;
int index = -1;
if (len > 0)
{
for (index = 0; index < len; index++)
{
Console.WriteLine("Select {0} for Course \"{1}\"", index, _courses.ElementAt(index).Title);
}
Console.WriteLine("--------------------------------------------------");
Console.Write(message);
string selection = Console.ReadLine();
int.TryParse(selection, out index);
while (!int.TryParse(selection, out index) || (index < 0 || index > len - 1))
{
Console.Write("Please make a valid selection: ");
selection = Console.ReadLine();
}
}
return index;
}
public void AddACourse()
{
string input;
Console.Write("How many assignments are in the course? ");
input = Console.ReadLine();
int numAssignments = 0;
int.TryParse(input, out numAssignments);
while (!int.TryParse(input, out numAssignments))
{
Console.Write("Please enter a number: ");
input = Console.ReadLine();
}
Course course = new Course(numAssignments);
Console.Write("What is the courses title? ");
course.Title = Console.ReadLine();
Console.Write("What is the courses description? ");
string descrip = Console.ReadLine();
course.Description = descrip;
_courses.Add(course);
}
public void RemoveACourse()
{
int index = SelectCourse("Select a course to remove. (Enter the number): ");
if (index == 0)
{
Console.WriteLine("No courses to remove. Try adding one first.");
}
else
{
_courses.RemoveAt(index);
}
}
public void AddGradesForACourse()
{
int index = SelectCourse("Select a course to add grades for. (Enter the number): ");
if (index == -1)
{
Console.WriteLine("No course to add grades to. Try adding one first.");
}
else
{
_courses[index].AddGrades();
}
}
public void DisplayGradesForACourse()
{
int index = SelectCourse("Select a course to display grades for. (Enter the number): ");
if (index == -1)
{
Console.WriteLine("No course to display grades for. Try adding one first.");
}
else
{
_courses[index].DisplayGrades();
}
}
public void DisplayAllGrades()
{
foreach (Course c in _courses)
{
c.DisplayGrades();
}
}
public void DisplayInfo()
{
Console.WriteLine("Name: {0} Age: {1} Address: {2} Phone: {3} Email: {4}", getName(), Age, Address, Phone, Email);
}
}
}
------------------------------Course.cs------------------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace GradeCalculator
{
class Course
{
string _title;
string _description;
Grade[] _grades;
bool _graded;
public string Title
{
get
{
return _title;
}
set
{
_title = value;
}
}
public string Description
{
get
{
return _description;
}
set
{
_description = value;
}
}
public Grade[] Grades
{
get
{
return _grades;
}
set
{
_grades = value;
}
}
public Course(int numberOfAssignments)
{
_grades = new Grade[numberOfAssignments];
_graded = false;
}
public void AddGrades()
{
for (int i = 0; i < _grades.Length; i++)
{
_grades[i] = new Grade();
Console.Write("Enter a description for assignment {0}: ", (i + 1));
_grades[i].Description = Console.ReadLine();
string input = "";
float value;
Console.Write("Enter the grade earned for assignment {0} as a percentage (0 - 100): ", (i + 1));
input = Console.ReadLine();
float.TryParse(input, out value);
while (!float.TryParse(input, out value))
{
Console.WriteLine("Please enter a number: ");
input = Console.ReadLine();
}
_grades[i].PercentEarned = value;
Console.Write("Enter assignment {0}'s weight of total grade as a percentage (0 - 100): ", (i + 1));
input = Console.ReadLine();
float.TryParse(input, out value);
while (!float.TryParse(input, out value))
{
Console.WriteLine("Please enter a number: ");
input = Console.ReadLine();
}
_grades[i].Weight = value;
}
_graded = true;
}
private bool ValidateWeightTotal()
{
float precisionFactor = 0.001f;
float _totalWeight = 0;
bool result = false;
for (int i = 0; i < _grades.Length; ++i)
{
_totalWeight += _grades[i].Weight;
}
if (_totalWeight < 100 + precisionFactor && _totalWeight > 100 - precisionFactor)
{
result = true;
}
if (result == false)
{
Console.WriteLine("Weight total = {0} instead of 100. Please enter the values again. ", _totalWeight);
}
return result;
}
public float GetFinalGrade()
{
bool weightsAreValid = ValidateWeightTotal();
float finalGrade = 0;
if (weightsAreValid)
{
for (int i = 0; i < _grades.Length; ++i)
{
finalGrade += _grades[i].GetPercentOfFinalGrade();
}
}
return finalGrade;
}
public void DisplayGrades()
{
if (_graded)
{
float total = 0f;
Console.WriteLine("-------------------------------------");
Console.WriteLine("Title: {0}", Title);
for (int i = 0; i < _grades.Length; ++i)
{
Grade grade = _grades[i];
total += grade.GetPercentOfFinalGrade();
Console.WriteLine("Desc: {0} Earned: {1} Percent towards final grade: {2} ", grade.Description, grade.PercentEarned, grade.GetPercentOfFinalGrade());
}
Console.WriteLine("Grade for the course: {0}", total);
Console.WriteLine("-------------------------------------");
}
else
{
Console.WriteLine("Please add grades first.");
}
}
}
}
------------------------------Grade.cs------------------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace GradeCalculator
{
class Grade
{
string _description;
float _percentEarned;
float _weight;
public string Description
{
get
{
return _description;
}
set
{
_description = value;
}
}
public float PercentEarned
{
get
{
return _percentEarned;
}
set
{
if (value < 0.0f || value > 100.0f)
{
_percentEarned = 0;
Console.WriteLine("Percent earned was less than 0 or greater than 100 so value was set to 0.");
}
else
{
_percentEarned = value;
}
}
}
public float Weight
{
get
{
return _weight;
}
set
{
if (value < 0.0f || value > 100.0f)
{
_weight = 0;
Console.WriteLine("Weight was less than 0 or greater than 100 so value was set to 0.");
}
else
{
_weight = Weight;
}
}
}
public float GetPercentOfFinalGrade()
{
float result = (_percentEarned * _weight) / 100f;
return result;
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
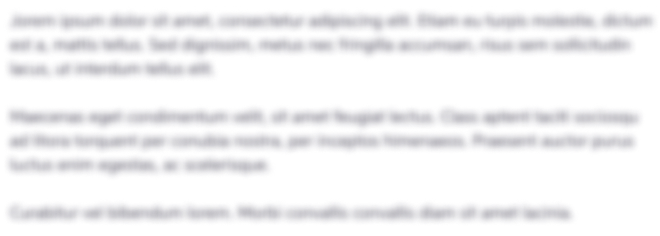
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started