Question
I can useget_size(), is_empty() and delete_minimum(). I am to define a Python function namedget_kth_smallest_list(priority_queue, k)which takes a priority queue and an integer as parameters andreturnsk
I can useget_size(), is_empty() and delete_minimum().
I am to define a Python function namedget_kth_smallest_list(priority_queue, k)which takes a priority queue and an integer as parameters andreturnsk smallest elements in the parameter priority queue.
Note: You need to ensure that you do not attempt to delete items if the priority queue is empty. Some examples of the function being used are shown below.
Test | Result |
print(get_kth_smallest_list(priority_queue1, 3)) | [5, 6, 7] |
priority_queue = PriorityQueue() print(get_kth_smallest_list(priority_queue, 2)) | [] |
priority_queue = PriorityQueue() num_list = [12, 26, 7, 95] for num in num_list: priority_queue.insert(num) print(get_kth_smallest_list(priority_queue, 6)) | [7, 12, 26, 95] |
class PriorityQueue: def __init__(self): self.binary_heap = [0] self.size = 0 def insert(self, value): self.binary_heap.append(value) self.size += 1 self.percolate_up(self.size) def delete_minimum(self): if not self.is_empty(): heap_root_index = 1 minimum_value = self.binary_heap[heap_root_index] self.binary_heap[heap_root_index] = self.binary_heap[self.size] self.binary_heap.pop() self.size -= 1 self.percolate_down(heap_root_index) return minimum_value def peek(self): if not self.is_empty(): heap_root_index = 1 minimum_value = self.binary_heap[heap_root_index] return minimum_value def is_empty(self): return self.size == 0 def percolate_up(self, index): while index // 2 > 0: if self.binary_heap[index] tmp = self.binary_heap[index // 2] self.binary_heap[index // 2] = self.binary_heap[index] self.binary_heap[index] = tmp index = index // 2 def percolate_down(self, index): while (index * 2) => smallest_child = self.get_smaller_child_index(index) if self.binary_heap[index] > self.binary_heap[smallest_child]: tmp = self.binary_heap[index] self.binary_heap[index] = self.binary_heap[smallest_child] self.binary_heap[smallest_child] = tmp index = smallest_child def get_smaller_child_index(self, index): if index * 2 + 1 > self.size: return index * 2 else: if self.binary_heap[index*2] return index * 2 else: return index * 2 + 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
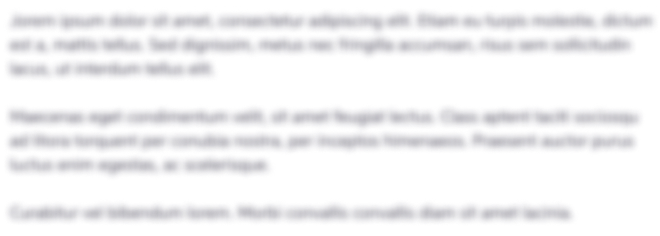
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started