Question
I cannot get this thing to work. Below is what i have so far. I have tried different ways to get my output,by following the
|
This application will declare an enumerated type DessertType, which will be used in a switch statement to determine which action needs to be taken.
Depending on the DessertType, the program should prompt for specific details in order to calculate the price for each item. CANDY Prompt for Name, Number of Pounds, Price per Pound
COOKIE Prompt for Name, Number of Cookies, Price per Dozen
ICE CREAM Prompt for Name, Number of Scoops, Price per Scoop
SUNDAE Prompt for Name, Number of Scoops, Price per Scoop, Number of Toppings and the Price per Topping
The application should be called DessertShop, which will prompt the user for items to purchase. This application will prompt the user for the name of the product. If no name is entered, the application will calculate the total cost of items entered.
The application will define four arrays for each DessertItem, which can store up to 10 items each.
Each derived class will contain a static variable which will store the total DessertItem cost and total DessertItem tax (if applicable) for that specific DessertType. The CANDY and COOKIE dessert types are taxable at 7%. The ICE CREAM and SUNDAY dessert types are non-taxable.
The DessertItem superclass will also contain a total cost and total tax static variable that will be shared between all items that were derived from that superclass.
Upon successfully entering an item, the total cost and total tax of that DessertItem will be updated, along with the total cost and total tax for all items. The application should check an item to determine if that item has already been entered, except for Sundaes. If the item has already been entered, the existing information should be updated to include the new items.
Upon entering all items, the application should print out a summary of the DessertItems, along with the count, the cost and tax. (See Sample Output) |
Sample Output |
CANDY [2] Cost: $4.52 Tax: $0.32 COOKIES [24] Cost: $12.96 Tax: $0.91 ICE CREAM [1] Cost: $3.99 SUNDAE [4] Cost: $8.87 ---- -------- ----- Subtotals [31] $30.34 $1.23
======== Total $31.57
After the total has been displayed, the application will prompt the user to see if they would like to save the receipt to a file. If yes, the application should prompt the user for the file name they would like to write the receipt to. The application should then open a text file and output the receipt information to the output file. |
import java.util.Scanner; public class DessertShopProject {
enum DessertType {
CANDY, COOKIES, ICECREAM, SUNDAE }; public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
String nameOfTheProduct;
//FOUR ARRAY(S) FOR EACH 'DESSERT_ITEM'....Store 10 items Candy candyArray[] = new Candy[10]; Cookie cookieArray[] = new Cookie[10]; IceCream iceCreamArray[] = new IceCream[10]; Sundae sundaeArray[] = new Sundae[10];
do { System.out.println("CANDY, COOKIES, ICE CREAM, SUNDAE");
System.out.println("Now, please enter the name of the desired product"); nameOfTheProduct = keyboard.nextLine(); DessertType dessertType = DessertType.valueOf(nameOfTheProduct.toUpperCase());
System.out.println("Please, enter the number items you wish to purchase"); int numberOfItems = keyboard.nextInt(); switch (dessertType) { case CANDY: System.out.println("please, enter a name"); String candyName = keyboard.nextLine(); System.out.println("Please, enter number of lbs"); double numberLbs = keyboard.nextDouble(); System.out.println("Please, enter price per pound"); double pricePerPound = keyboard.nextDouble(); for (int i = 0; i < candyArray.length; i++) { candyArray[i] = new Candy(candyName, numberLbs, pricePerPound); } break; case COOKIES: System.out.println("please, enter a name"); String cookieName = keyboard.nextLine(); System.out.println("Please, enter number of cookies"); int numberCookies = keyboard.nextInt(); System.out.println("Please, enter price per dozen"); double pricePerDozen = keyboard.nextDouble(); for (int i = 0; i < cookieArray.length; i++) { cookieArray[i] = new Cookie(cookieName, numberCookies, pricePerDozen); } break; case ICECREAM: System.out.println("please, enter a name"); String iceCreamName = keyboard.nextLine(); System.out.println("Please, enter number of scoops"); int numberOfScoops = keyboard.nextInt(); System.out.println("Please, enter price per scoop"); double pricePerScoop = keyboard.nextDouble(); for (int i = 0; i < iceCreamArray.length; i++) { iceCreamArray[i] = new IceCream(iceCreamName, numberOfScoops, pricePerScoop); } break; case SUNDAE: System.out.println("please, enter a name"); String sundaeName = keyboard.nextLine(); System.out.println("Please, enter number of scoops"); int numberOfSundaeScoops = keyboard.nextInt(); System.out.println("Please, enter price per scoop"); double pricePerSundaeScoop = keyboard.nextDouble(); System.out.println("please, enter number of toppings"); int numberOfToppings = keyboard.nextInt(); System.out.println("Please, enter price per topping"); double pricePerTopping = keyboard.nextDouble(); for (int i = 0; i < sundaeArray.length; i++) { sundaeArray[i] = new Sundae(sundaeName, numberOfSundaeScoops, pricePerSundaeScoop, numberOfToppings, pricePerTopping); } break;
default: System.out.println("Sorry, you entered something incorrectly"); break;
}//END SWITCH_STATEMENT
} while (false); }//End Main() }//End Desert Shop Class
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
package dessertshopproject; public abstract class DessertItem {
protected String name; static double dessertItemCost; static double totalDessertItemTax;
public DessertItem() { }
public DessertItem(String name) { this.name = name; }
//GETTERS--just the name--down the road public String getName() { return name; }
public abstract double respectiveDessertTotalCost();
}//END DESSERT_ITEM CLASS
///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
package dessertshopproject;
import java.text.NumberFormat;
public class Candy extends DessertItem {
private String candyName; private double candyNumberOfLbs; private double candyPricePerLbs; //Each Derived Class will contain a static variable!! Cost/Tax static double totalCandyCost; static double totalCandyTax;
public Candy(String name, double candyNumberOfLbs, double candyPricePerLbs) { super(name); this.candyNumberOfLbs = candyNumberOfLbs; this.candyPricePerLbs = candyPricePerLbs; }
public String getCandyName() { return candyName; }
public double getCandyNumberOfLbs() { return candyNumberOfLbs; }
public double getCandyPricePerLbs() { return candyPricePerLbs; }
@Override public double respectiveDessertTotalCost() { double result; totalCandyCost = (candyNumberOfLbs * candyPricePerLbs); totalCandyTax = (totalCandyCost * 0.07); /////////////////////////////////////// result = totalCandyCost + totalCandyTax; return result; } //End Respective_Dessert_Total_Cost
@Override public String toString() { NumberFormat formatter = NumberFormat.getCurrencyInstance(); return (super.getName() + "\t\tCost: " + formatter.format(this.respectiveDessertTotalCost()) + "\t\tTax: " + formatter.format(totalCandyTax)); }
}// END CLASS CANDY
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
package dessertshopproject;
import static dessertshopproject.DessertItem.dessertItemCost; import java.text.NumberFormat; import java.util.Scanner;
public class Cookie extends DessertItem {
private String cookieName; private int numberOfCookies; private double cookiePricePerDozen; //Each Derived Class will contain a static variable!! Cost/Tax private static double totalCookieCost; private static double totalCookieTax;
public Cookie(String name, int numberOfCookies, double cookiePricePerDozen) { super(name); this.numberOfCookies = numberOfCookies; this.cookiePricePerDozen = cookiePricePerDozen; }
public int getNumberOfCookies() { return numberOfCookies; }
public double getCookiePricePerDozen() { return cookiePricePerDozen; }
@Override public double respectiveDessertTotalCost() { double result; totalCookieCost = (numberOfCookies * (cookiePricePerDozen / 12)); totalCookieTax = (totalCookieCost * 0.07);//------->Only CANDY/COOKIES /////////////////////////////////////// result = totalCookieCost + totalCookieTax; dessertItemCost += totalCookieCost; return result; }//End Respective_Dessert_Total_Cost
@Override public String toString() { NumberFormat formatter = NumberFormat.getCurrencyInstance(); return (super.getName() + "\t\tCost: " + formatter.format(this.respectiveDessertTotalCost()) + "\t\tTax: " + formatter.format(totalCookieTax)); }
}//End Class_Cookie
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
package dessertshopproject;
import java.text.NumberFormat;
public class IceCream extends DessertItem {
private String iceCreamName; private int numberOfScoops; private double iceCreamPricePerScoop; //Each Derived Class will contain a static variable!! Cost/Tax-- Not ICE_CREAM/SUNDAYS private static double totalIceCreamCost;
public IceCream(String name, int numberOfScoops, double iceCreamPricePerScoop) { super(name); this.numberOfScoops = numberOfScoops; this.iceCreamPricePerScoop = iceCreamPricePerScoop; }
public int getNumberOfScoops() { return numberOfScoops; }
public double getIceCreamPricePerScoop() { return iceCreamPricePerScoop; }
@Override public double respectiveDessertTotalCost() { double result; totalIceCreamCost = (numberOfScoops * iceCreamPricePerScoop); /////////////////////////////////////// result = totalIceCreamCost; return result; }//End Respective_Dessert_Total_Cost
@Override public String toString() {
NumberFormat formatter = NumberFormat.getCurrencyInstance();
return (super.getName() + "\t\t" + "Cost: " + formatter.format(this.respectiveDessertTotalCost()) + "\t"); }
}//END ICE_CREAM CLASS
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
package dessertshopproject;
import java.text.NumberFormat;
public class Sundae extends DessertItem {
private String sundaeName; private int sundaeNumberOfScoops; private double sundaePricePerScoop; private int sundaeNumberOfToppings; private double sundaePricePerTopping; //Each Derived Class will contain a static variable!! Cost/Tax-- Not ICE_CREAM/SUNDAYS private static double totalSundaeCost;
public Sundae(String name, int sundaeNumberOfScoops, double sundaePricePerScoop, int sundaeNumberOfToppings, double sundaePricePerTopping) { super(name); this.sundaeNumberOfScoops = sundaeNumberOfScoops; this.sundaePricePerScoop = sundaePricePerScoop; this.sundaeNumberOfToppings = sundaeNumberOfToppings; this.sundaePricePerTopping = sundaePricePerTopping; }
public int getSundaeNumberOfScoops() { return sundaeNumberOfScoops; }
public double getSundaePricePerScoop() { return sundaePricePerScoop; }
public int getSundaeNumberOfToppings() { return sundaeNumberOfToppings; }
public double getSundaePricePerTopping() { return sundaePricePerTopping; }
@Override public double respectiveDessertTotalCost() { double result;
totalSundaeCost = ((sundaeNumberOfScoops * sundaePricePerScoop) + (sundaeNumberOfToppings * sundaePricePerTopping)); /////////////////////////////////////// result = totalSundaeCost; return result; }//End Respective_Dessert_Total_Cost
@Override public String toString() {
NumberFormat formatter = NumberFormat.getCurrencyInstance();
return (super.getName() + "\t\t\t" + "Cost: " + formatter.format(this.respectiveDessertTotalCost()) + "\t"); }
}//END SUNDAE CLASS
/////////////////////// THANK YOU
Step by Step Solution
There are 3 Steps involved in it
Step: 1
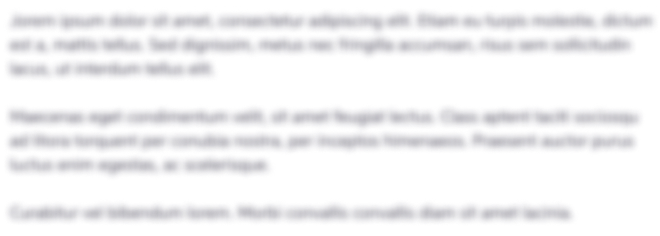
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started