Question
I could use some help with the branches on my assembly language program. Objective of program: > Read the number of t-shirts the customer wishes
I could use some help with the branches on my assembly language program.
Objective of program:
> Read the number of t-shirts the customer wishes to buy. >Asks the customer if they have a coupon. >If number is less than 0, an "invalid entry" display is issued, then restarts. >If the quantity purchased is 1-49, each shirt costs $7. >If the quantity purchased is 50-99, each shirt costs $6. >If the quantity purchased 100+, each shirt costs $5.
Problem: My program reads "invalid entry" regardless of input.
###################################################################################### # Data Section # ######################################################################################
.data count1: .asciiz " How many T-shirts would you like to order? " coupon2: .asciiz " Do you have a discount coupon? Enter 1 for yes. (any other integer will indicate no discount) " charge3: .asciiz " Your total payment is: " invalid5: .asciiz " Invalid entry; Restarting program. "
price1: .word 7 price2:.word 6 price3:.word 5
###################################################################################### # Executed Program # ######################################################################################
.text .globl main
main:
##################################################################################### # Prompt user # #####################################################################################
la $a0, count1 # "How many T-shirts would you like to order?" li $v0, 4 syscall
li $v0, 5 syscall move $s3, $v0
la $a0, coupon2 # "Do you have a discount coupon? Enter 1 for yes." li $v0, 4 syscall
li $v0, 5 syscall move $s2, $v0
##################################################################################### # Load quantities into registers # #####################################################################################
lw $t3, price1 # Loads price1 of "$7" into register $s7. lw $t3, price2 # Loads price2 of "$6" into register $s7. lw $t4, price3 # Loads price3 of "$5" into register $s7.
addi $t2, $zero, 0 # Loads quantity1 of "0" into register $t2. addi $s0, $zero, 1 # Loads quantity1 of "1" into register $s0. addi $s1, $zero, 49 # Loads quantity1 of "49" into register $s1. addi $s5, $zero, 50 # Loads quantity1 of "50" into register $s5. addi $s6, $zero, 99 # Loads quantity1 of "99" into register $s6. addi $s7, $zero, 100 # Loads quantity1 of "100" into register $s7.
##################################################################################### # Use branch to jump to proper labels # #####################################################################################
ble $s3, $t2, INVALID # if input is less than or equal to 0, go to "INVALID". bge $s3, $s6, GREATERTHAN10 # if input is greater than or equal to 1, go to "LESSTHAN49".
INVALID: la $a0, invalid5 # "Invalid entry; Restarting program." li $v0, 4 syscall j main
GREATERTHAN10: ble $s3, $s1, LESSTHAN49 # if input is less than or equal to 49, go to "LESSTHAN49". bgt $s3, $s1, GREATERTHAN49 # if input is greater than or equal to 49, go to "GREATERTHAN49".
LESSTHAN49: mult $s3, $t3 # $t3 = multiplication of number of shirts by $7. mfhi $s3 # loads the upper 32 bits from the product register mflo $s2 # loads the lower 32 bits from the product register beq $s2, $s0, DISCOUNT # if customer indicates a coupon, jump to "COUPON". beq $s2, $s0, PRICE # if customer indicates no coupon, jump to "PRICE".
GREATERTHAN49: ble $s3, $s1, LESSTHAN99 # if input is less than or equal to 99, go to "LESSTHAN49". bgt $s3, $s1, GREATERTHAN99 # if input is greater than or equal to 99, go to "GREATERTHAN49".
LESSTHAN99: mult $s3, $t3 # $t3 = multiplication of number of shirts by $6. mfhi $s3 # loads the upper 32 bits from the product register mflo $s2 # loads the lower 32 bits from the product register beq $s2, $s0, DISCOUNT # if customer indicates a coupon, jump to "COUPON". beq $s2, $s0, PRICE # if customer indicates no coupon, jump to "PRICE".
GREATERTHAN99: mult $s3, $t4 # $t4 = multiplication of number of shirts by $5. mfhi $s3 # loads the upper 32 bits from the product register mflo $s2 # loads the lower 32 bits from the product register beq $s2, $s0, DISCOUNT # if customer indicates a coupon, jump to "COUPON". beq $s2, $s0, PRICE # if customer indicates no coupon, jump to "PRICE".
##################################################################################### # Reads back total payment # ####################################################################################
DISCOUNT: sub $s3, $s3, $t4 #subtracts $5 from total price. j PRICE
PRICE: la $a0, charge3 # Selects "charge3" defined at ".data"; "Your total payment is: " li $v0, 4 # System Call 4 - print string syscall # Command
li $v0, 5 # System Call 5 - read integer syscall # Command move $s3, $v0 # Moves user input integer into $s3 beq $s0, $s2, DISCOUNT
j exitprogram
Step by Step Solution
There are 3 Steps involved in it
Step: 1
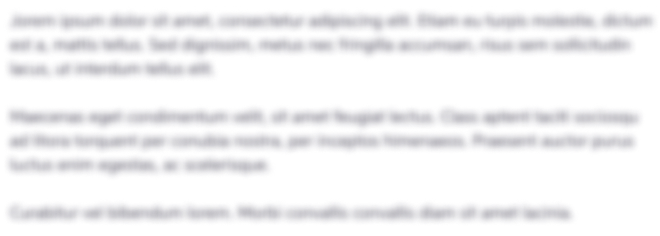
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started