Question
I could use some help with the following assignment. Thank you! Here are the starting project files: https://www.filehosting.org/file/details/782775/CustomerManager.zip The following is the code from the
I could use some help with the following assignment. Thank you!
Here are the starting project files: https://www.filehosting.org/file/details/782775/CustomerManager.zip
The following is the code from the given files.
Console.java
import java.util.Scanner;
public class Console {
private static Scanner sc = new Scanner(System.in);
public static String getLine(String prompt) {
System.out.print(prompt);
String s = sc.nextLine(); // read the whole line
return s;}
public static String getString(String prompt) {
System.out.print(prompt);
String s = sc.next(); // read the first string on the line
sc.nextLine(); // discard the rest of the line
return s;}
public static int getInt(String prompt) {
int i = 0; boolean isValid = false;
while (!isValid) {
System.out.print(prompt);
if (sc.hasNextInt()) {
i = sc.nextInt(); isValid = true;
} else {
System.out.println("Error! Invalid integer value. Try again.");}
sc.nextLine(); // discard any other data entered on the line}
return i;}
public static int getInt(String prompt, int min, int max) {
int i = 0; boolean isValid = false;
while (!isValid) {
i = getInt(prompt);
if (i
System.out.println("Error! Number must be greater than " + min + ".");
else if (i >= max)
System.out.println("Error! Number must be less than " + max + ".");
else
isValid = true;}
return i;}
public static double getDouble(String prompt) {
double d = 0; boolean isValid = false;
while (!isValid) {
System.out.print(prompt);
if (sc.hasNextDouble()) { d = sc.nextDouble(); isValid = true;
} else { System.out.println("Error! Invalid decimal value. Try again."); }
sc.nextLine(); // discard any other data entered on the line } return d;}
public static double getDouble(String prompt, double min, double max) {
double d = 0; boolean isValid = false;
while (!isValid) { d = getDouble(prompt);
if (d
System.out.println("Error! Number must be greater than " + min);
else if (d >= max)
System.out.println("Error! Number must be less than " + max);
else
isValid = true; }
return d; }}
Customer.java
public class Customer {
private String firstName; private String lastName; private String email;
public Customer() { this("", "", ""); }
public Customer(String firstName, String lastName, String email) { this.firstName = firstName; this.lastName = lastName; this.email = email; }
public void setFirstName(String firstName) { this.firstName = firstName; }
public String getFirstName() { return firstName; }
public void setLastName(String lastName) { this.lastName = lastName; }
public String getLastName() { return lastName; }
public void setEmail(String email) { this.email = email; }
public String getEmail() { return email; }
public String getName() { return firstName + " " + lastName; }}
CustomerManagerApp.java
import java.util.List;
public class CustomerManagerApp {
private static DAO customerDAO = null; // declare class variables
public static void main(String[] args) {
System.out.println("Welcome to the Customer Manager "); // display a welcome message
customerDAO = new CustomerTextFile(); // set the class variables
displayMenu(); // display the command menu
String action = ""; // perform 1 or more actions
while (!action.equalsIgnoreCase("exit")) {
action = Console.getString("Enter a command: "); // get the input from the user
System.out.println();
if (action.equalsIgnoreCase("list")) { displayAllCustomers();
} else if (action.equalsIgnoreCase("add")) { addCustomer();
} else if (action.equalsIgnoreCase("del") || action.equalsIgnoreCase("delete")) { deleteCustomer();
} else if (action.equalsIgnoreCase("help") || action.equalsIgnoreCase("menu")) { displayMenu();
} else if (action.equalsIgnoreCase("exit")) { System.out.println("Bye. ");
} else { System.out.println("Error! Not a valid command. ");}}}
public static void displayMenu() {
System.out.println("COMMAND MENU"); System.out.println("list - List all customers"); System.out.println("add - Add a customer"); System.out.println("del - Delete a customer"); System.out.println("help - Show this menu"); System.out.println("exit - Exit this application ");}
public static void displayAllCustomers() {
System.out.println("CUSTOMER LIST"); List customers = customerDAO.getAll(); Customer c;
StringBuilder sb = new StringBuilder();
for (int i = 0; i
c = customers.get(i); sb.append(StringUtils.padWithSpaces(c.getName(), 27));
sb.append(c.getEmail()); sb.append(" "); } System.out.println(sb.toString()); }
public static void addCustomer() {
String firstName = Console.getLine("Enter first name: "); String lastName = Console.getString("Enter last name: ");
String email = Console.getString("Enter customer email: "); Customer = new Customer();
customer.setFirstName(firstName); customer.setLastName(lastName); customer.setEmail(email); customerDAO.add(customer); System.out.println(); System.out.println(firstName + " " + lastName + " has been added. "); }
public static void deleteCustomer() {
String email = Console.getString("Enter email to delete: "); Customer c = customerDAO.get(email); System.out.println();
if (c != null) {customerDAO.delete(c); System.out.println(c.getName()+ " has been deleted. ");
} else {System.out.println("No customer matches that email. ");}}}
DAO.java
import java.util.List;
public interface DAO { T get(String code); List getAll(); boolean add(T t); boolean update(T t); boolean delete(T t); }
StringUtils.java
public class StringUtils {
public static String padWithSpaces(String s, int length) {
if (s.length()
while(sb.length()
return sb.toString(); } else { return s.substring(0, length); }}}
CustomerTextFile.java
import java.util.*; import java.io.*; import java.nio.file.*;
public final class CustomerTextFile implements DAO
private List
public CustomerTextFile() { customersPath = Paths.get("customers.txt"); customersFile = customersPath.toFile(); customers = this.getAll(); }
@Override public List
if (customers != null) { // if the customers file has already been read, don't read it again
return customers;}
if(true) { try { throw new IOException("Test"); } catch (IOException e) { e.printStackTrace(); }}
customers = new ArrayList();
if (Files.exists(customersPath)) { // prevent the FileNotFoundException
try (BufferedReader in = new BufferedReader(new FileReader(customersFile))) {
String line = in.readLine(); // read all customers stored in the file into the array list
while (line != null) { String[] columns = line.split(FIELD_SEP); String firstName = columns[0]; String lastName = columns[1]; String email = columns[2]; Customer c = new Customer(firstName, lastName, email); customers.add(c); line = in.readLine(); }
} catch (IOException e) { System.out.println(e); return null; }} return customers; }
@Override public Customer get(String email) { for (Customer c : customers) { if (c.getEmail().equals(email)) { return c; }} return null; }
@Override public boolean add(Customer c) { customers.add(c); return this.saveAll(); }
@Override public boolean delete(Customer c) { customers.remove(c); return this.saveAll(); }
@Override public boolean update(Customer newCustomer) {
Customer oldCustomer = this.get(newCustomer.getEmail()); // get the old customer and remove it
int i = customers.indexOf(oldCustomer); customers.remove(i);
customers.add(i, newCustomer); // add the updated customer
return this.saveAll();}
private boolean saveAll() {
try (PrintWriter out = new PrintWriter(new BufferedWriter(new FileWriter(customersFile)))) {
for (Customer c : customers) { // write all customers in the array list to the file
out.print(c.getFirstName() + FIELD_SEP); out.print(c.getLastName() + FIELD_SEP); out.println(c.getEmail());}
} catch (IOException e) { System.out.println(e); return false; } return true; }}
Chapter 16 Project: Customer Manager Improve the exception handling for an application that manages customer data. Console for a FileNotFoundException Welcome to the Customer Manager Error reading data file! Exiting application. java.io.FileNotFoundException: customers.txt (The system cannot find the file specified) Console for an IOException Welcome to the Customer Manager COMMAND MENU list add del help exit - List all customers -Add a customer - Delete a customer -Show this menu - Exit this application Enter a command: add Enter first name: John Enter last name: Doe Enter customer email: johndoe@x.com Error adding customer. Try again. Enter a command: Specifications . Your instructor should provide you with a starting project for a Customer Manager application that allows you to add and delete customer records. This source code should be the same as the solution for exercise 15-1 in the book Modify the CustomerTextFile class so it throws all exceptions to the calling class. To get this to work, you'll need to modify the DAO interface so each of its methods throws the IOException. .Modify the CustomerManager App class so it handles all exceptions appropriately . If the application can't find the file that stores the data, display an error message and exit the application. To cause a FileNotFoundException, you can move or rename the data file If the application can't add or delete a record, display an error message and allow the user to try again. To simulate an IOException, you can add a statement to the CustomerTextFile class that throws an IOException. . Chapter 16 Project: Customer Manager Improve the exception handling for an application that manages customer data. Console for a FileNotFoundException Welcome to the Customer Manager Error reading data file! Exiting application. java.io.FileNotFoundException: customers.txt (The system cannot find the file specified) Console for an IOException Welcome to the Customer Manager COMMAND MENU list add del help exit - List all customers -Add a customer - Delete a customer -Show this menu - Exit this application Enter a command: add Enter first name: John Enter last name: Doe Enter customer email: johndoe@x.com Error adding customer. Try again. Enter a command: Specifications . Your instructor should provide you with a starting project for a Customer Manager application that allows you to add and delete customer records. This source code should be the same as the solution for exercise 15-1 in the book Modify the CustomerTextFile class so it throws all exceptions to the calling class. To get this to work, you'll need to modify the DAO interface so each of its methods throws the IOException. .Modify the CustomerManager App class so it handles all exceptions appropriately . If the application can't find the file that stores the data, display an error message and exit the application. To cause a FileNotFoundException, you can move or rename the data file If the application can't add or delete a record, display an error message and allow the user to try again. To simulate an IOException, you can add a statement to the CustomerTextFile class that throws an IOExceptionStep by Step Solution
There are 3 Steps involved in it
Step: 1
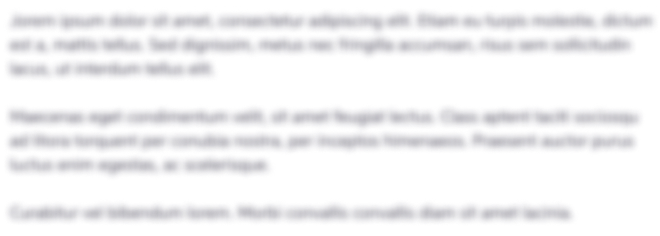
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started