Question
I get the white empty screen like in the first screenshot. But the program's menu should be like in the second screenshot. What's the problem?
I get the white empty screen like in the first screenshot. But the program's menu should be like in the second screenshot. What's the problem? Can you please fix it? The format HAS TO BE JavaFX.
The code that I am using is down below. IT SHOULD BE WRITTEN WITH JAVAFX.
package application;
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class PayableInterfaceTest extends Application{ public static void main(String[] args) { launch(args); }
@Override public void start(Stage primaryStage) throws Exception { Payable[] payableObjects = new Payable[6];
payableObjects[0] = new Invoice("01234", "seat", 2, 375.00); payableObjects[1] = new Invoice("56789", "tire", 4, 79.95); payableObjects[2] = new SalariedEmployee("John", "Smith", "111-11-1111", 800.00); payableObjects[3] = new HourlyEmployee("Bob", "Marley", "222-22-2222", 33.00, 40); payableObjects[4] = new CommissionEmployee("Jessica", "Johnson", "333-33-3333", 1350, 0.85); payableObjects[5] = new BasePlusCommissionEmployee("Jenny", "James", "666-66-6666", 1175, 0.95, 660.00);
StringBuilder invoicesAndEmployees = new StringBuilder("Invoices and Employees processed polymorphically: "); for(Payable currentPayable : payableObjects){ invoicesAndEmployees.append(currentPayable.toString()).append(" ") .append("Payment due").append(": $").append(String.format("%,.2f", currentPayable.getPaymentAmount())).append(" "); }
for (Payable currentPayable : payableObjects){ if(currentPayable instanceof BasePlusCommissionEmployee){ BasePlusCommissionEmployee employee = (BasePlusCommissionEmployee)currentPayable; double employeePay = employee.getBaseSalary(); double newSalary = employeePay + (employeePay * .10); employee.setBaseSalary(newSalary); invoicesAndEmployees.append("The base pay of ").append(employee.getFirstName()).append(" ").append(employee.getLastName()) .append(" with a 10% raise is ").append(String.format("%.2f", newSalary)).append(" "); } }
StackPane root = new StackPane(); Scene scene = new Scene(root, 600, 400); primaryStage.setTitle("Payable Interface Test"); primaryStage.setScene(scene); primaryStage.show(); } }
public class CommissionEmployee extends Employee { private double grossSales; private double commissionRate;
public CommissionEmployee(String first, String last, String ssn, double sales, double rate){ super(first, last, ssn); setGrossSales(sales); setCommissionRate(rate); }
public void setCommissionRate(double rate){ if(rate > 0.0 && rate 0.0 and
public double getCommissionRate(){ return commissionRate; }
public void setGrossSales(double sales){ if(sales >= 0.0){ grossSales = sales; }else{ throw new IllegalArgumentException("Gross sales must be >= 0.0"); } }
public double getGrossSales(){ return grossSales; }
@Override public double getPaymentAmount(){ return getCommissionRate() * getGrossSales(); }
@Override public String toString(){ return String.format("%s: %s %s: $%,.2f; %s: %.2f", "commission employee", super.toString(), "gross sales", getGrossSales(), "commission rate", getCommissionRate()); }
}
public class BasePlusCommissionEmployee extends CommissionEmployee { private double baseSalary; public BasePlusCommissionEmployee(String first, String last, String ssn, double sales, double rate, double salary){ super(first, last, ssn, sales, rate); setBaseSalary(salary); }
public void setBaseSalary(double salary){ if(salary >= 0.0){ baseSalary = salary; }else{ throw new IllegalArgumentException( "Base salary must be >= 0.0"); } }
public double getBaseSalary(){ return baseSalary; }
@Override public double getPaymentAmount(){ return getBaseSalary() + super.getPaymentAmount(); }
@Override public String toString(){ return String.format("%s %s; %s: $%,.2f", "Base-salaried", super.toString(), "Base salary", getBaseSalary()); } }
public abstract class Employee implements Payable { private final String firstName; private final String lastName; private final String socialSecurityNumber; public Employee(String first, String last, String ssn){ firstName = first; lastName = last; socialSecurityNumber = ssn; }
public String getFirstName(){ return firstName; }
public String getLastName(){ return lastName; }
public String getSocialSecurityNumber(){ return socialSecurityNumber; }
@Override public String toString(){ return String.format("%s %s %s: %s", getFirstName(), getLastName(), "social security number", getSocialSecurityNumber()); }
@Override public abstract double getPaymentAmount();
}
public class HourlyEmployee extends Employee { private double wage; private double hours; public HourlyEmployee(String first, String last, String ssn, double hourlyWage, double hoursWorked){ super(first, last, ssn); setWage(hourlyWage); setHours(hoursWorked); }
private void setHours(double hoursWorked) { // TODO Auto-generated method stub }
public void setWage(double hourlyWage){ if(hourlyWage >= 0.0){ wage = hourlyWage; }else{ throw new IllegalArgumentException( "Hourly wage must be >= 0.0"); } }
public double getWage(){ return wage; }
@Override public double getPaymentAmount() { // TODO Auto-generated method stub return 0; } }
public class Invoice implements Payable { private final String partNumber; private final String partDescription; private int quantity; private double pricePerItem;
public Invoice(String part, String description, int count, double price){ partNumber = part; partDescription = description; setQuantity(count); setPricePerItem(price); }
public void setQuantity(int count){ if(count >= 0){ quantity = count; }else{ throw new IllegalArgumentException("Quantity must be >= 0"); } }
public int getQuantity(){ return quantity; }
public void setPricePerItem(double price){ if(price >= 0.0){ pricePerItem = price; }else{ throw new IllegalArgumentException( "Price per item must be >= 0"); } }
public double getPricePerItem(){ return pricePerItem; }
@Override public String toString(){ return String.format("%s: %n%s: %s (%s) %n%s: %d %n%s: $%,.2f", "invoice", "part number", getPartNumber(), getPartDescription(), "quantity", getQuantity(), "price per item", getPricePerItem()); }
public String getPartNumber(){ return partNumber; }
public String getPartDescription(){ return partDescription; }
@Override public double getPaymentAmount(){ return getQuantity() * getPricePerItem(); }
}
public interface Payable { double getPaymentAmount(); }
public class SalariedEmployee extends Employee { private double weeklySalary; public SalariedEmployee(String first, String last, String ssn, double salary){ super(first, last, ssn); setWeeklySalary(salary); }
public void setWeeklySalary(double salary){ if(salary >= 0.0){ weeklySalary = salary; }else{ throw new IllegalArgumentException( "Weekly salary must be >= 0.0"); } }
public double getWeeklySalary(){ return weeklySalary; }
@Override public double getPaymentAmount(){ return getWeeklySalary(); }
@Override public String toString(){ return String.format("salaried employee: %s %s: $%,.2f", super.toString(), "weekly salary", getWeeklySalary()); }
}
FIRST SCREENSHOT
SECOND SCREENSHOT
Step by Step Solution
There are 3 Steps involved in it
Step: 1
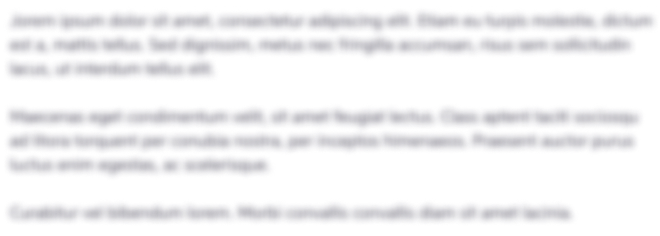
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started