Question
I got GUI, animal, reptile and mammal here, and i do not know if i need to write ArrayList by myself. Can you help me
I got GUI, animal, reptile and mammal here, and i do not know if i need to write ArrayList by myself. Can you help me to finish this lab? thanks
GUI:
package gui;
import java.awt.*; import java.awt.event.*; import javax.swing.*;
public class AnimalSortFrameStart extends JFrame implements ActionListener {
/** * GUI component for getting the name */ private GetInputPanel namePanel = null; /** * GUI component for getting the weight */ private GetInputPanel weightPanel = null; /** * GUI component for getting the age */ private GetComboPanel agePanel = null; /** * GUI component for getting the length */ private GetComboPanel lengthPanel = null; /** * GUI component for getting the color */ private GetInputPanel colorPanel = null;
/** * Button for adding a reptile to the collection */ private final JButton addReptileButton = new JButton("Add Reptile"); /** * Button for adding a mammal to the collection */ private final JButton addMammalButton = new JButton("Add Mammal"); /** * Button for displaying animals in the collection */ private final JButton displayAnimalsButton = new JButton("Display Animals"); /** * Button for sorting animals in the collection by name */ private final JButton sortAgeButton = new JButton("Sort Animals by Age"); /** * Button for sorting animals in the collection by kind */ private final JButton sortKindButton = new JButton("Sort Animals by kind"); /** * A for displaying data */ private final JTextArea verifyArea = new JTextArea(25, 45);
/** * Creates a new instance of AddAnimalFrame which is contains panels and other * GUI components */ public AnimalSortFrameStart() { super("Add and Sort Animals by name and by kind"); createGUI(); }
/** * A method to create GUI components */ private void createGUI() { Container c = this.getContentPane(); c.setLayout(new BorderLayout(5, 5));
JPanel inputPanel = new JPanel(); //contains GUI to input animals info inputPanel.setLayout(new GridLayout(5, 1)); namePanel = new GetInputPanel(20, " Animal's Name: "); inputPanel.add(namePanel); weightPanel = new GetInputPanel(6, " Animal's Weight (lb): "); inputPanel.add(weightPanel); agePanel = new GetComboPanel(" Animal's Age (years):", 125); inputPanel.add(agePanel); lengthPanel = new GetComboPanel("Reptile's Length (cm)", 1000); inputPanel.add(lengthPanel); colorPanel = new GetInputPanel(20, "Mammal's skin/fur color"); inputPanel.add(colorPanel);
JPanel buttonPanel = new JPanel(); //contains buttons buttonPanel.setLayout(new GridLayout(1, 5, 5, 5)); addReptileButton.setToolTipText("Press to add Reptile"); addMammalButton.setToolTipText("Press to add Mammal"); buttonPanel.add(addReptileButton); buttonPanel.add(addMammalButton);
buttonPanel.add(displayAnimalsButton); buttonPanel.add(sortAgeButton); buttonPanel.add(sortKindButton);
c.add(inputPanel, BorderLayout.NORTH); c.add(buttonPanel, BorderLayout.CENTER); JScrollPane scrollPane = new JScrollPane(verifyArea); c.add(scrollPane, BorderLayout.SOUTH);
addReptileButton.addActionListener(this); addMammalButton.addActionListener(this); displayAnimalsButton.addActionListener(this);
sortAgeButton.addActionListener(this); sortKindButton.addActionListener(this); setVisible(true); }
/** * main() instantiates an object * * @param argv */ static public void main(String[] argv) { AnimalSortFrameStart animalFrame = new AnimalSortFrameStart(); animalFrame.setSize(800, 650); animalFrame.setDefaultCloseOperation(EXIT_ON_CLOSE); }
/** * Responds to the "Display" and "Add" buttons * * @param ev The button press event */ public void actionPerformed(ActionEvent ev) { Object object = ev.getSource(); if (object == addReptileButton) {
} else if (object == addMammalButton) {
} else if (object == sortKindButton) {
} else if (object == sortAgeButton) {
} else {
} }
/** * A panel prompting for String input. It contains a label and a text field. */ class GetInputPanel extends JPanel {
private JTextField inputField; //used for the user input
/** * Constructor sets up a label and the text field * * @param size the size of the input text field * @param prompt the message specifying expected input */ public GetInputPanel(int size, String prompt) { inputField = new JTextField(size); JLabel label = new JLabel(prompt); add(label); add(inputField); }
/** * Gets the text from the text field * * @return Returns the text from the text field */ public String getText() { return inputField.getText(); }
/** * Converts the text field value into a number and displays an error message * when inputed data contains non digit characters * * @return the integer represented by the user input */ public double getValue() { double value = 0.0; try { value = Double.parseDouble(inputField.getText()); } catch (NumberFormatException ex) { JOptionPane.showMessageDialog(null, "Invalid characters in number", "Input Error", JOptionPane.ERROR_MESSAGE); } return value; } }
/** * This panel represents a panel with a label and a combo box. */ class GetComboPanel extends JPanel {
JLabel label; //explains the purpose of the combo box JComboBox ageCombo; //used for the user input
/** * Constructor sets up a panel with a label and a combo box. * * @param message the text indicating the purpose of the combo box * @param numChoices the range of choices displayed in the combo box */ public GetComboPanel(String message, int numChoices) { label = new JLabel(message); String[] age = new String[numChoices];
for (int i = 0; i
add(label); add(ageCombo); }
/** * Gets the value from the combo box * * @return value selected from the combo box */ public int getValue() { int a; a = Integer.parseInt((String) ageCombo.getSelectedItem()); return a; } } }
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Animals:
package animal; import javax.swing.*; import exceptions.*; public class Animal implements Comparable{
/** * give an initial name */ protected String name = null; /** * give an initial value of Weight */ protected double weight = 0.0; /** * give an initial value of age */ protected int age = 0;
/** * @param name Name of the animal * @param age age of animal * @param weight Weight of animal * @throws InvalidNameException * @throws InvalidWeightException */ public Animal(String name, int age,double weight)throws InvalidWeightException , InvalidNameException { if (name.length() 0){ return 1; } return 0; } }
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Mammal:
package animal;
/** * */ import exceptions.InvalidNameException; import exceptions.InvalidWeightException; import javax.swing.JTextArea; public class Mammal extends Animal {
private String hairColor= null;
/** * * @param name * @param weight * @param age * @param hairColor * @throws InvalidWeightException * @throws InvalidNameException */ public Mammal(String name, int age,double weight, String hairColor) throws InvalidWeightException, InvalidNameException { super(name, age, weight); this.hairColor = hairColor; }
/** * @param hairColor */ public void setColor(String hairColor) { this.hairColor = hairColor; }
/** * gets the color of the mammal * * @return the color of the mammal */ public String getColor() { return hairColor; }
/** * Displays a Mammal in the textArea * * @param output a text area to display an information about the animal */ @Override public void display(JTextArea output) { super.display(output); output.append("\t hair/fur color: " + hairColor); }
/** * Returns a string representation of the Mammal object * * @return a string containing contents of the object's fields */ @Override public String toString() { return (super.toString() + "\t hair/fur color: " + hairColor); } }
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Reptile:
package animal;
/** * */ import exceptions.InvalidNameException; import exceptions.InvalidWeightException; import javax.swing.JTextArea; public class reptile extends Animal {
private int length;
/** * * @param name * @param weight * @param age * @param length * @throws InvalidWeightException * @throws InvalidNameException */ public reptile(String name, int age,Double weight, int length) throws InvalidWeightException, InvalidNameException { super(name, age, weight); this.length = length; } /** * * @param length */ public void setLength(int length) { this.length = length; } /** * * @return the length of reptile */ public int getLength() { return this.length; } /** * * @param output a text area to display an information about the animal */ @Override public void display(JTextArea output) { super.display(output); output.append("length" + length); } /** * * @return a string representation of the reptile object */ @Override public String toString() { return (super.toString() + "length" + this.length); } }
Objectives: Using ArrayList class from java.util package Using sort method from java Collections class Implementing Comparable interface Writing a comparator Using an iterator to iterate over the collection Lab Assignment: 1. In this lab, you are going to store animals in the ArrayList collection from java.util package Use method add from ArrayList class to add animals to the list and use method sort from the Collections class to sort animals by the age in ascending order and by kind in ascending order (mammals first then reptiles) 2. Modify method compareTo in class Animal so it compares animals by age in ascending order 3. Use method add from the ArrayList class to add the animals to the list 4. To display animals you must use an iterator from the ArrayList class instead of method toString from the ArrayList. Use the iterator irn AnimalGUI to step through the collection 5. To sort animals by age use method sort(ListStep by Step Solution
There are 3 Steps involved in it
Step: 1
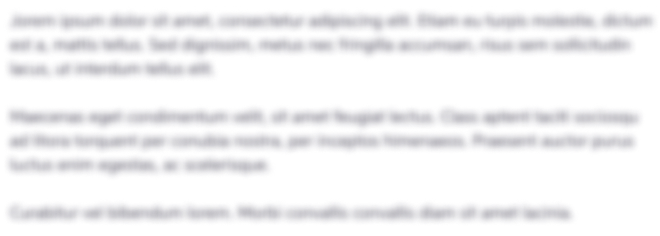
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started