Question
I had to implement this Priority queue interface with an ordered array. for example say I have an array of 5 4 3 3 3
I had to implement this Priority queue interface with an ordered array. for example say I have an array of 5 4 3 3 3 2 1 the 1 in index 6 has a higher priority than 5 at index zero. I am stuck with the delete method. The code on my delete method is an idea I have but it is probably not right. To delete the object getting passed in first I use binary search to locate it because it is a requirement but after that I am not to sure what to do. Say I want to delete all the 3's from the above array I use binary search to see if my array contains a 3 and then I need to delete all of them so that means my currSize (items in array) become less. Here are the instructions for the delete method:
// Deletes all instances of the parameter obj from the PQ if found, and
// returns true. Returns false if no match to the parameter obj is found.
public boolean delete(E obj);
public interface PriorityQueue
public static final int DEFAULT_MAX_CAPACITY = 1000;
// Inserts a new object into the priority queue. Returns true if
// the insertion is successful. If the PQ is full, the insertion
// is aborted, and the method returns false.
public boolean insert(E object);
// Removes the object of highest priority that has been in the
// PQ the longest, and returns it. Returns null if the PQ is empty.
public E remove();
// Deletes all instances of the parameter obj from the PQ if found, and
// returns true. Returns false if no match to the parameter obj is found.
public boolean delete(E obj);
// Returns the object of highest priority that has been in the
// PQ the longest, but does NOT remove it.
// Returns null if the PQ is empty.
public E peek();
// Returns true if the priority queue contains the specified element
// false otherwise.
public boolean contains(E obj);
// Returns the number of objects currently in the PQ.
public int size();
// Returns the PQ to an empty state.
public void clear();
// Returns true if the PQ is empty, otherwise false
public boolean isEmpty();
// Returns true if the PQ is full, otherwise false. List based
// implementations should always return false.
public boolean isFull();
// Returns an iterator of the objects in the PQ, in no particular
// order.
public Iterator
}
package data_structures; import java.util.Iterator; import java.util.NoSuchElementException;
public class OrderedArrayPriorityQueue
//Here's where I am stuck public boolean delete(E obj) { E temp; int pos = findInsertionPoint(obj, 0, currSize-1); for(int i = 0; i < currSize; i++) { if(obj.compareTo(queue[pos]) == 0) temp = queue[--pos]; return true; } return false; } public E peek() { if(isEmpty()) return null; return queue[currSize-1]; } public boolean contains(E obj) { int match = findInsertionPoint(obj, 0, currSize-1); if(obj.compareTo(queue[match]) == 0) return true; return false; } public int size() { return currSize; } public void clear() { currSize = 0; } public boolean isEmpty() { return currSize == 0; } public boolean isFull() { return currSize == capacity; } public Iterator
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
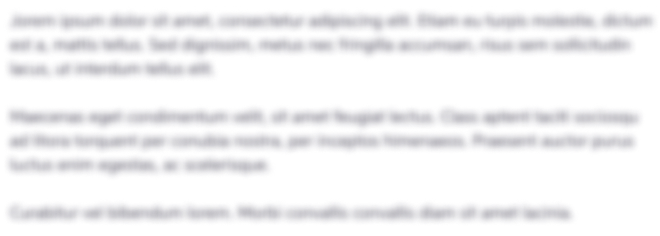
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started