Question
i have 2 version of a maze i want to know how the second version is implementeded # this is version 1 class Maze: def
i have 2 version of a maze i want to know how the second version is implementeded
# this is version 1
class Maze:
def __init__(self,filename):
file = open(filename, "r")
lines = file.readlines()
dimensions = json.loads(lines[0])
themaze = json.loads(lines[1])
file.close()
self.num_rows = dimensions['maxRow']
self.num_cols = dimensions['maxCol']
self.num_cells = self.num_rows * self.num_cols
self.cells = [False for i in range(self.num_cells)]
self.walls = [[False for j in range(self.num_cells)] for i in range(self.num_cells)]
for the_cells in themaze["walls"]:
self.walls[the_cells[0]][the_cells[1]] = True
self.walls[the_cells[1]][the_cells[0]] = True
# returns total number of rows in the maze
def get_num_rows(self):
return self.num_rows
# returns total number of columns in the maze
def get_num_cols(self):
return self.num_cols
# returns the cell_number given row and col
def get_cell(self, row, col):
return row * self.num_cols + col
# returns the row given cell_number
def get_row(self, cell_number):
return cell_number//self.num_cols
# returns the col given cell_number
def get_col(self, cell_number):
return cell_number % self.num_cols
# returns the cell_number of the cell to the left of the cell_number
# if there is no cell on the left or there is a wall between current cell
# and left cell, function returns -1
def get_left(self, cell_number):
col = self.get_col(cell_number)
row = self.get_row(cell_number)
if col == 0:
return -1
else:
left_cell = self.get_cell(row,col-1)
if not self.walls[left_cell][cell_number]:
return left_cell
return -1
# returns the cell_number of the cell to the right of the cell_number
# if there is no cell on the right or there is a wall between current cell
# and right cell, function returns -1
def get_right(self, cell_number):
col = self.get_col(cell_number)
row = self.get_row(cell_number)
if col == self.num_cols-1:
return -1
else:
right_cell = self.get_cell(row,col+1)
if not self.walls[right_cell][cell_number]:
return right_cell
return -1
# returns the cell_number of the cell to the up of the cell_number
# if there is no cell on the up or there is a wall between current cell
# and up cell, function returns -1
def get_up(self, cell_number):
col = self.get_col(cell_number)
row = self.get_row(cell_number)
if row == 0:
return -1
else:
up_cell = self.get_cell(row-1,col)
if not self.walls[up_cell][cell_number]:
return up_cell
return -1
# returns the cell_number of the cell to the down of the cell_number
# if there is no cell on the down or there is a wall between current cell
# and down cell, function returns -1
def get_down(self, cell_number):
col = self.get_col(cell_number)
row = self.get_row(cell_number)
if row == self.num_rows-1:
return -1
else:
down_cell = self.get_cell(row+1,col)
if not self.walls[down_cell][cell_number]:
return down_cell
return -1
# marks the cell with cell_number, this will allow you to leave a mark on
# the cells that are part of your path (or being considered to be on your path)
def mark_cell(self, cell_number):
self.cells[cell_number]=True
# ummarks the cell
def unmark_cell(self, cell_number):
self.cells[cell_number]=False
# returns true of cell_number is marked, false otherwise
def get_is_marked(self,cell_number):
return self.cells[cell_number]
def print_pathfile(filename, result, row, col):
the_file = open(filename,"w")
the_file.write(f'{{"rows": {row}, "cols": {col},')
the_file.write(f' "pathLength": {len(result)},')
the_file.write(f'"path":{result}')
the_file.write("}")
the_file.close()
# i want an explanation how this second version is implemented
"""
import from Maze.py
"""
def find_path(the_maze, from_cell, to_cell):
"""
Define the function to take in the maze and the starting and ending cells.
Define the inner function for DFS traversal.
"""
def lf_search(cell):
"""
If the current cell is the target cell, return the cell as the path.
"""
if cell == to_cell:
return [cell]
# Mark the current cell as visited.
the_maze.mark_cell(cell)
# Iterate through all possible moves from the current cell (up, down, left, and right).
for next_cell in [the_maze.get_up(cell), the_maze.get_down(cell), the_maze.get_left(cell),
the_maze.get_right(cell)]:
# If the cell is valid and hasn't been visited before, continue DFS on that cell.
if next_cell != -1 and not the_maze.get_is_marked(next_cell):
# Recursive call to the dfs function.
p_found = lf_search(next_cell)
# If a path is found, prepend the current cell to the path and return.
if p_found:
return [cell] + p_found
# If no path is found from the current cell, return None.
return None
# Start LFS from the start cell.
path_found = lf_search(from_cell)
# Return the found path or an empty list if no path is found.
return path_found if path_found else []
Step by Step Solution
There are 3 Steps involved in it
Step: 1
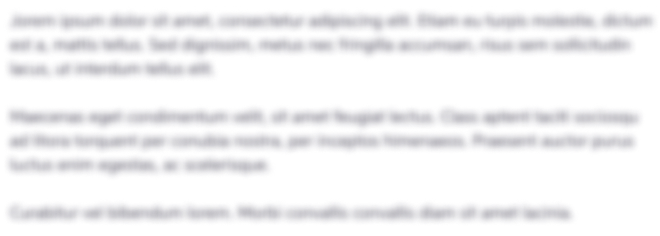
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started