Question
I have a deck of cards program that deals a hand evaluates the results. My problem is that since my card values are Strings i.e.
I have a deck of cards program that deals a hand evaluates the results. My problem is that since my card values are Strings i.e. (String[] faces = {"Ace", "Deuce", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King"};) I am struggling to sort them in order to determine if the hand has a straight.
This the code for the class that evaluates the hand. The two methods that are checking for a straight are sortByRank and isStraight.
---------------------------------------------------------------------------------------------------------------------------------------------
public class DeckOfCards {
// random number generator
private static final SecureRandom randomNumbers = new SecureRandom();
private static final int NUMBER_OF_CARDS = 52; // constant # of Cards
public static int flushn = 0;
public static int straightn=0;
//private Card[] hand;
private Card[] deck = new Card[NUMBER_OF_CARDS]; // Card references
private int currentCard = 0; // index of next Card to be dealt (0-51)
// constructor fills deck of Cards
public DeckOfCards() {
String[] faces = {"Ace", "Deuce", "Three", "Four", "Five", "Six",
"Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King"};
String[] suits = {"Hearts", "Diamonds", "Clubs", "Spades"};
// populate deck with Card objects
for (int count = 0; count < deck.length; count++) {
deck[count] =
new Card(faces[count % 13], suits[count / 13]);
}
} //end of DeckOfCards constructor
public String getCard(){
String cCard = ("");
return cCard;
}
// shuffle deck of Cards with one-pass algorithm
public void shuffle() {
// next call to method dealCard should start at deck[0] again
currentCard = 0;
// for each Card, pick another random Card (0-51) and swap them
for (int first = 0; first < deck.length; first++) {
// select a random number between 0 and 51
int second = randomNumbers.nextInt(NUMBER_OF_CARDS);
// swap current Card with randomly selected Card
Card temp = deck[first];
deck[first] = deck[second];
deck[second] = temp;
}
} //end of shuffle method
// deal one Card
public Card dealCard() {
// determine whether Cards remain to be dealt
if (currentCard < deck.length) {
return deck[currentCard++]; // return current Card in array
}
else {
return null; // return null to indicate that all Cards were dealt
}
}//end of dealCard method
public static void hasFlush(Card[] hand) {
boolean isFlush = true;
String suit = hand[0].getSuit();
for(int i = 1; i < hand.length; i++) {
if(!(hand[i].getSuit().equals(suit))) {
isFlush = false;
}
}
if(isFlush){
flushn = 5;
}
}//end of flush evaluation
public static void sortByRank( Card[] hand )
{
int i, j, min_j;
/* ---------------------------------------------------
The selection sort algorithm
--------------------------------------------------- */
for ( i = 0 ; i < hand.length ; i ++ )
{
/* ---------------------------------------------------
Find array element with min. value among
hand[i], hand[i+1], ..., hand[n-1]
--------------------------------------------------- */
min_j = i; // Assume elem i (hand[i]) is the minimum
for ( j = i+1 ; j < hand.length ; j++ )
{
if ( hand[j].getFace() < hand[min_j].getFace() )
{
min_j = j; // We found a smaller rank value, update min_j
}
}
/* ---------------------------------------------------
Swap a[i] and a[min_j]
--------------------------------------------------- */
Card help = hand[i];
hand[i] = hand[min_j];
hand[min_j] = help;
}
}
public static boolean isStraight( Card[] hand )
{
int i, testF=0;
String testface;
if ( hand.length != 5 )
return(false);
sortByRank(hand); // Sort the poker hand by the face of each card
/* ===========================
Check if hand has an Ace
=========================== */
if ( hand[4].getFace() == "Ace" )
{
/* =================================
Check straight using an Ace
================================= */
boolean a = hand[0].getFace() == "Deuce" && hand[1].getFace() == "Three" &&
hand[2].getFace() == "Four" && hand[3].getFace() == "Five" ;
boolean b = hand[0].getFace() == "Ten" && hand[1].getFace() == "Jack" &&
hand[2].getFace() == "Queen" && hand[3].getFace() == "King" ;
return ( a || b );
}
else
{
/* ===========================================
General case: check for increasing values
=========================================== */
testface = hand[testF].getFace() + 1;
for ( i = 1; i < 5; i++ )
{
if ( hand[i].getFace() != testface )
return(false); // Straight failed...
testF++; // Next card in hand
}
return(true); // Straight found !
}
}//end of straight evaluation
public static int Pair(){
int pair = 0, done = 0, handcount = 1;
int pairCheck=0;
while (done <1){
for(int i = 0; i < 4; i++){
String tempCard = DeckOfCardsTest.hand[i].getFace();
for(int j=handcount; j < DeckOfCardsTest.hand.length; j++){
if(tempCard.equals(DeckOfCardsTest.hand[j].getFace()))
pair++;
}
handcount++;
}
pairCheck = pair;
done++;
}
return pairCheck;
}//endof Pair method
public static String Results(){
String results=("High Card");
int checkHand = 0;
if (Pair()>0 ){
switch (Pair()){
case 1:
results = ("Pair");
break;
case 2:
results = ("Two Pair!");
break;
case 3:
results = ("Three of a kind!");
break;
case 4:
results = ("Full House!");
break;
case 6:
results = ("FOUR of a kind!");
break;
default:
results = ("Somthing went terribly wrong, sorry");
break;
}
}//end of pairing results
if (flushn > 0){
results = ("Flush!");
}
if (straightn > 0){
results = ("Straight!");
}
return results;
}
}//end of Class
-------------------------------------------------------------------------------------
public class Card { private final String face; // face of card ("Ace", "Deuce", ...) private final String suit; // suit of card ("Hearts", "Diamonds", ...)
// two-argument constructor initializes card's face and suit public Card(String cardFace, String cardSuit) { this.face = cardFace; // initialize face of card this.suit = cardSuit; // initialize suit of card } //end of Card inilization
// return String representation of Card public String toString() { return face + " of " + suit; }// endof toString method public String getFace() { return face; }//end of getFace method public String getSuit() { return suit; }//end of getSuit method }//end of Class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
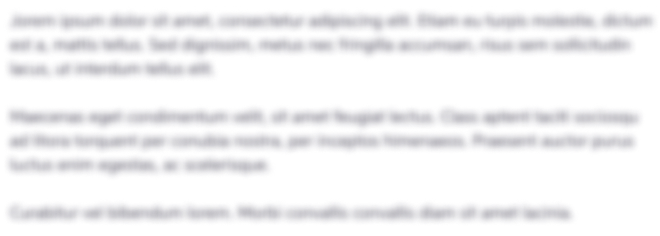
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started