Question
I have a program (see code below) in which the user can add items, remove items, change the amount, display the current list, save and
I have a program (see code below) in which the user can add items, remove items, change the amount, display the current list, save and load --> a shoppinglist! Right now, the amount of items in the list are restricted to a maximum of 5. I need help with the following:
*Allow the user to enter how many items as they want, use dynamic memory to solve this problem. Start by removing the restriction of 5 items (which I have), next resize the dynamic array (list-->itemList) to fit another item (remember that the dynamic array start as empty, so this has to be done even when you add just one item).To resize the dynamic array, use realloc(). I have major problems in this function, it just keeps crashing when I try to add an item to the list, I don't know how to fix it!
*If the user adds the same item twice (or more), update the amount of the item instead of having the same item written twice in the list!
*To save and load the list, you can choose whether to use binary files or text files. For saveList(): When the user chooses to save a shopping list, the user should first select a file name. If you use binary files note that the shoppingList is a pointer! This means you can't save the whole shoppingList struct with one fwrite(), you have to save the length field separately first and then save the dynamic array of items with another fwrite(). For loadList(): The user should first be asked for a filename,if the file doesn't exist return a error message. If the user is currently working with a shoppinglist, that list should not be destroyed if the file could not open! When loading a list, it should overwrite the previous list the user was working with. New memory should be allocated for the loaded list. First, load the size of the list and then allocate memory for the list. You can do this in two ways, either by using free() on the old list and call malloc() with the appropiate new size , or you can use realloc().Make sure there is no memory leak! Note, it's important that you need to let the itemList member point to the new memory that is returned from malloc() or realloc() ,otherwise strange errors may occur.
main.c-file:
#define _CRT_SECURE_NO_WARNINGS #include
int main(void) { struct ShoppingList shoppingList; shoppingList.length = 0; // The shopping list is empty at the start shoppingList.itemList = 0;
int option;
do { printf(" Welcome to the shopping list manager! "); printf("===================================== ");
printf("1. Add an item "); printf("2. Display the shopping list "); printf("3. Remove an item "); printf("4. Change an item "); printf("5. Save list "); printf("6. Load list "); printf("7. Exit ");
printf("What do you want to do? "); scanf_s("%d", &option);
switch (option) { case 1: addItem(&shoppingList); break; case 2: printList(&shoppingList); break; case 3: removeItem(&shoppingList); break; case 4: editItem(&shoppingList); break; case 5: saveList(&shoppingList); break; case 6: loadList(&shoppingList); break; case 7: break; default: printf("Please enter a number between 1 and 7"); } } while (option != 7);
free(shoppingList.itemList); return 0; }
shoppingList.h-file:
#ifndef SHOPPING_LIST_H #define SHOPPING_LIST_H
// Struct definitions
struct GroceryItem { char productName[20]; float amount; char unit[10]; };
struct ShoppingList { int length; struct GroceryItem* itemList; };
// Function declarations
void addItem(struct ShoppingList *list); void printList(struct ShoppingList *list); void editItem(struct ShoppingList *list); void removeItem(struct ShoppingList *list); void saveList(struct ShoppingList *list); void loadList(struct ShoppingList* list);
#endif
shoppingList.c-file:
#define _CRT_SECURE_NO_WARNINGS #include"ShoppingList.h" #include
void addItem(struct ShoppingList* list) { printf("===================================== "); printf("Your list contains %d items ", list->length);
struct GroceryItem* itemList = (struct GroceryItem*)malloc(sizeof(struct GroceryItem) * (list->length));
while (getchar() != ' '); printf("Enter name: "); gets(list->itemList[list->length].productName);
printf("Enter amount: "); scanf_s("%f", &list->itemList[list->length].amount);
while (getchar() != ' '); printf("Enter unit: "); gets(list->itemList[list->length].unit); list->length++; free(itemList); itemList = NULL;
printf("The list now contains %d items ", list->length);
struct GroceryItem* itemList = (struct GroceryItem*)realloc(itemList, sizeof(struct GroceryItem) * (list->length + 1)); if (itemList != NULL) { itemList = itemList; } else { printf("NULL"); }
return 0; }
...
//code
...
void saveList(struct ShoppingList *list)//shopping_list.txt { FILE* file; //create a pointer to file,which is the variable that will handle the file. char filename; struct GroceryItem* itemList; int length; printf("Enter filename: "); gets(filename);
file = fopen(filename, "wb"); //We now open the file and "w" makes it possible to write to the file.ShoppingList?
if (file != NULL) { //Spara lngden p listan fwrite(length, sizeof(int), 1, file); //Spara sjlva listan fwrite(itemList, sizeof(struct GroceryItem), length, file); fclose(file); } else { printf("The file could not be opened!"); return -1; }
for (int i = 0; i < list->length; i++) { fprintf(file, "%s %.1f %s ", list->itemList[i].productName, list->itemList[i].amount, list->itemList[i].unit); } fclose(file); return 0; }
void loadList(struct ShoppingList* list) { FILE* file; char filename; int length; printf("Enter filename: "); gets(filename); file = fopen(filename, "rb"); //"r" makes it possible to read from the file
//Kod fr att checka om filen existerar?? if (filename == NULL) { printf("The file does not exist!"); } else { fread(list, sizeof(struct ShoppingList), length, file); fread(list, sizeof(struct GroceryItem), length, file); struct GroceryItem* itemList = (struct GroceryItem*)realloc(itemList,sizeof(struct GroceryItem) * length); //?? fclose(file); }
list->length = 0;
if (file == NULL) { printf("The file could not be opened!"); return -1; }
char line[100]; while (fgets(line, 100, file)) { struct GroceryItem item; fscanf_s(line, "%s %f %s", item.productName, &item.amount, item.unit); addItem(list, item.productName, item.amount, item.unit); }
fclose(file); return 0; }
To remove the restriction of 5 items, I have added the following in the main.c-file:
shoppingList.itemList=NULL;
After that, I've tried to modify the addItem() function to allow the user to enter how many items as possible. But the program keeps crashing when I try to add an item and I'm not sure how to fix it.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
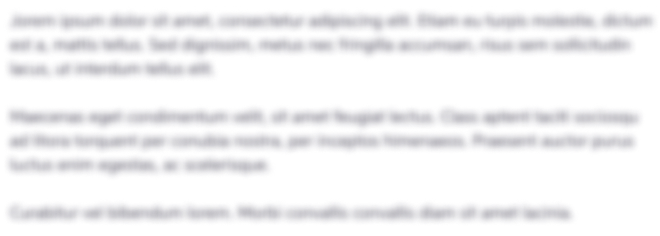
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started