Question
I have a wrong output. MINE is : P sa 8 P li 7 P he 9 P tu 5 F li 7 tu 5
I have a wrong output. MINE is :
P sa 8
P li 7
P he 9
P tu 5
F li 7 tu 5
F li 7 he 9
F tu 5 sa 8
L tu 5
li 7 sa 8
L sa 8
tu 5
U li 7 tu 5
L tu 5
sa 8
Q li 7 he 9
Yes
Q he 9 li 7
Yes
Q he 9 li 23
No
Q he 9 he 9
No
but here is the intended input and output:
INPUT OUTPUt
* p john 8
* p mike 7
* p sara 9
* p jane 5
* f mike 7 jane 5
* f mike 7 sara 9
* f jane 5 john 8
* l jane 5 mike 7 john 8
* l john 8 jane 5
* u mike 7 jane 5
* l jane 5 john 8
* q mike 7 sara 9 yes
* q sara 9 mike 7 yes
* q sara 9 mike 23 no
* q sara 9 sara 9 no
Heres my code:
public class MyFB {
public static boolean DEBUG = true;
public static Person makePerson (In in) {
try {
String name = in.readString ();
int age = in.readInt ();
return makePerson (name, age);
} catch (java.util.InputMismatchException e) {
StdOut.println ("Input format error");
return null;
}
}
Person k;
HashMap
public MyFB() {
map = new HashMap
}
public MyFB(HashMap
this.map = map;
}
public HashMap
return map;
}
public static Person makePerson (String name, int age) {
return new Person (name, age);
}
static class Person {
String name;
int age;
public Person (String name, int age) {
this.name = name;
this.age = age;
}
public String toString () {
return name + " " + age;
}
@Override
public boolean equals (Object obj) {
if(this== obj)
return true;
if ((obj == null) || (obj.getClass() !=
this.getClass()))
return false;
Person t = (Person) obj;
return age == t.age && (name == t.name || (name != null && name.equals(t.name)));
}
@Override
public int hashCode () {
int hash = 31;
hash = 31 * hash + ((Integer) age).hashCode();
hash = 31 * hash + (null == name ? 0 : name.hashCode());
return hash;
}
}
// a person "exists" after they are added using addPerson
// addPerson does nothing if p already exists
public void addPerson (Person p) {
if (DEBUG) {
StdOut.format("P %s ", p);
}
if (map.containsKey(p)) {
return;
}
map.put(p, new HashSet
}
// addFriendship does nothing if p1 and p2 are already friends or if one does not exist
public void addFriendship (Person p1, Person p2) {
if (DEBUG) {
StdOut.format("F %s %s ", p1, p2);
}
if (p1.equals(p2)) {
return;
}
if (!map.containsKey(p1) || !map.containsKey(p2)) {
return;
}
HashSet
HashSet
if(!s1.contains(p2))
{
s1.add(p2);
s2.add(p1);
}
}
// removeFriendship does nothing if p1 and p2 are not friends or if one does not exist
public void removeFriendship (Person p1, Person p2) {
if (DEBUG) {
StdOut.format("U %s %s ", p1, p2);
}
if (p1.equals(p2)) {
return;
}
if (!map.containsKey(p1) || !map.containsKey(p2)) {
return;
}
HashSet
HashSet
if(s1.contains(p2))
{
s1.remove(p2);
s2.remove(p1);
}
}
// queryFriendship returns false if p1 and p2 are not friends or if one does not exist
public boolean queryFriendship (Person p1, Person p2) {
if (DEBUG) {
StdOut.format("Q %s %s ", p1, p2);
}
if (p1 == p2) {
return false;
}
if (!map.containsKey(p1) || !map.containsKey(p2)) {
return false;
} else {
HashSet
return s1.contains(p2);
}
}
// lookupFriends returns null or empty iterable if p does not exists
public Iterable
if (DEBUG) {
StdOut.format("L %s ", p);
}
if (!map.containsKey(p))
return null;
else {
return map.get(p);
}
}
public static void hashTest () {
Trace.showBuiltInObjects (true);
//Trace.run ();
Person x = makePerson ("bob", 27);
Person y = makePerson ("bob", 27);
Boolean eq = x.equals(y);
Boolean hash = x.hashCode() == y.hashCode();
StdOut.println(x.toString() + " ("+x.hashCode()+") == " + y.toString() + " ("+y.hashCode()+")");
StdOut.println( " Equal Test: " + (eq ? "equal" : "not equal") );
StdOut.println( " Hash Test: " + (hash ? "equal" : "not equal") );
x = makePerson ("mike", 27);
y = makePerson ("john", 29);
eq = x.equals(y);
hash = x.hashCode() == y.hashCode();
StdOut.println(x.toString() + " ("+x.hashCode()+") == " + y.toString() + " ("+y.hashCode()+")");
StdOut.println( " Equal Test: " + (eq ? "equal" : "not equal") );
StdOut.println( " Hash Test: " + (hash ? "equal" : "not equal") );
}
public static void main (String[] args) {
Trace.showBuiltInObjects (true);
//Trace.run ();
hashTest();
/*
* The first line below causes "in" to read from the console. You can
* change this to read from a file, by using something like the line
* below, where "input.txt" is a file you create in your eclipse
* workspace. The file should be in the same folder that contains "src"
* "bin" and "lib":
*/
//In[] inputFiles = new In[] { new In () }; // from console
In[] inputFiles = new In[] { new In ("input.txt") }; // from file
//In[] inputFiles = new In[] { new In ("input.txt"), new In () }; // from file, then console
MyFB fb = new MyFB ();
StdOut.println (" ");
StdOut.println ("Enter one of the following:");
StdOut.println (" P name age -- add person");
StdOut.println (" F name1 age1 name2 age2 -- add friendship");
StdOut.println (" U name1 age1 name2 age2 -- remove friendship");
StdOut.println (" Q name1 age1 name2 age2 -- query friendship");
StdOut.println (" L name age -- lookup friends");
StdOut.println (" R -- restart");
StdOut.println (" X -- exit ");
for (In in : inputFiles) {
while (in.hasNextLine ()) {
String action;
try {
action = in.readString ();
} catch (NoSuchElementException e) { continue; }
//StdOut.println (action); // while debugging, print debugging info here! You can print maps, sets, lists.
switch (action) {
case "P":
case "p": {
Person p = makePerson (in);
fb.addPerson (p);
break;
}
case "F":
case "f": {
Person p1 = makePerson (in);
Person p2 = makePerson (in);
fb.addFriendship (p1, p2);
break;
}
case "U":
case "u": {
Person p1 = makePerson (in);
Person p2 = makePerson (in);
fb.removeFriendship (p1, p2);
//Trace.draw ();
break;
}
case "Q":
case "q": {
Person p1 = makePerson (in);
Person p2 = makePerson (in);
boolean result = fb.queryFriendship (p1, p2);
StdOut.println (result ? "Yes" : "No");
break;
}
case "L":
case "l": {
Person p = makePerson (in);
Iterable
if (result != null) {
for (Person friend : result) {
StdOut.print (friend);
StdOut.print (" ");
}
}
StdOut.println ();
break;
}
case "R":
case "r":
fb = new MyFB ();
break;
case "X":
case "x":
System.exit (0);
}
}
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
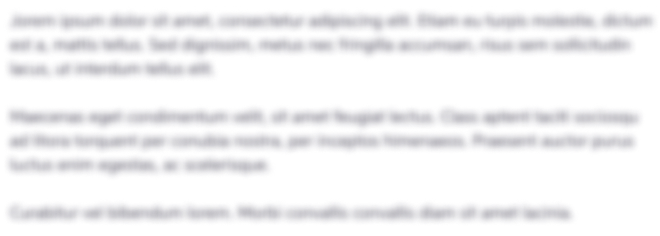
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started