Question
I have already done my program for the .h and .cpp files. I just need help in adding the operators and showing the revised files.
I have already done my program for the .h and .cpp files. I just need help in adding the operators and showing the revised files. Can you show me how it's done without using the friend function? Explain the process if you can.
You are asked to do the following:
- In complexno.h, add overloading unary operator - for the negation, overloading binary operators +, -, *, and << for the methodes add( const Complexno & ),sub(const Complexno&), mult(Complexno&)); and void shownum(); respectively.
- Add overloaded operator / for the division operator, so that it can do division on two complex numbers
- Modify your implementation file complexno.cpp to implement the Complexno class and the necessary overloaded operators.
- Change your revised file names to complexnum.h and complexnum.cpp.
- Copy the driver to test your codes.
- Show your revised files.
.h File:
#ifndef complexno_h #define complexno_h #include
class Complexno { public : Complexno(): Complexno(0, 0){} // Default constructor Complexno(double r): Complexno(r, 0){} // Second constructor - creates a complex number of equal value to a real. Complexno(double r, double c): real(r), complex(c){} // (1) Standard constructor - sets both of the real and complex
// components based on parameters. Complexno add(const Complexno& num2); // Adds two complex numbers and returns the answer. Complexno sub(const Complexno& num2); // Subtracts two complex numbers and returns the answer. Complexno mult(const Complexno& num2);// Multiplies two complex numbers and returns this answer. double magnitude(); //Computes and returns the magnitude of a complex number. void shownum(); //Prints out a complex number in a readable format.
Complexno negate(); // Negates a complex number. void enternum(); // Reads in a complex number from the user.
private : double real; // Stores real component of complex number double complex; // Stores complex component of complex number };
// Displays the answer to a complex number operation. void display(Complexno, Complexno, Complexno, char); #endif
.cpp file:
#include "complexno.h"
// Adds two complex numbers and returns the answer. Complexno Complexno::add(const Complexno& num2) { Complexno answer; answer.real = real + num2.real; answer.complex = complex + num2.complex; return answer; } // (2) --------------------------------- subtract------------------ // Define sub to subtracts two complex numbers and returns the answer. Complexno Complexno::sub(const Complexno& num2) { Complexno answer; answer.real = real - num2.real; answer.complex = complex - num2.complex; return answer; }
// (3) --------------------------------- Multiply------------------ // Multiplies two complex numbers and returns this answer. Complexno Complexno::mult(const Complexno& num2) { Complexno answer; answer.real = real * num2.real - complex * num2.complex; answer.complex = real * num2.complex + complex * num2.real; return answer; }
// Negates a complex number. Complexno Complexno::negate() { Complexno answer; answer.real = -real; answer.complex = -complex; return answer; } // (4) --------------------------------- Magnitude ------------------ // Computes and returns the magnitude of a complex number. double Complexno::magnitude() { double answer; answer = sqrt(real * real + complex * complex); return answer; } // (5) --------------------------------- Print ------------------ // Prints out a complex number in a readable format. void Complexno::shownum() { cout << real; if (complex > 0) { cout << "+"; } if (complex != 0) { cout << complex << "i"; } }
// Reads in a complex number from the user. void Complexno::enternum() { cout<<"Enter real component: "; cin >> real; cout<<"Enter complex component: "; cin >> complex; }
// Displays the answer to a complex number operation. void display(Complexno n1, Complexno n2, Complexno n3, char op) { n1.shownum(); cout << " " << op << " "; n2.shownum(); cout << " = "; n3.shownum(); cout << endl; }
driver(main function). Be sure to use it exactly as it is provided.
#include "complexnum.h"
int main() { cout.setf(ios::fixed); cout.setf(ios::showpoint); cout.precision(1); Complexno n1, n2, n3; cout << "Welcome to the complex number calculator. "; // Display menu options and read in the user's choice. int choice; while(true){ cout << " Would you like to "; cout << "1. Add 2 complex numbers. "; cout << "2. Subtract 2 complex numbers. "; cout << "3. Multiply 2 complex numbers. "; cout << "4. Divide 2 complex numbers. "; cout << "5. Find the magnitude of a complex number. "; cout << "6. Negate a complex number "; cout << "7. Quit "; cin >> choice; if (choice==7) { cout << "You quit the calculator. "; return (0); } // Read in the necessary complex numbers from the user. cout << "Enter the first complex number : "; n1.enternum(); if (choice<5) { cout << "Enter the second complex number : "; n2.enternum(); } // Execute the appropriate choice. if (choice == 1) { n3 = n1 + n2; cout << n1 << " + " << n2 << " = " << n3 << endl; } else if (choice == 2) { n3 = n1 - n2; cout << n1 << " - " << n2 << " = " << n3 << endl; }
else if (choice == 3) { n3 = n1 * n2; cout << n1 << " * " << n2 << " = " << n3 << endl; } else if (choice == 4) { n3 = n1 / n2; cout << n1 << " / " << n2 << " = " << n3 << endl; } else if (choice == 5) { cout << "|" << n1 << "| = " << n1.magnitude() << endl; } else if (choice==6) { n3 = -n1; cout << "-" << n1 << " = " << n3 << endl; } else cout << "Sorry, that was not a valid choice. Please try it again: "; } return 0;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
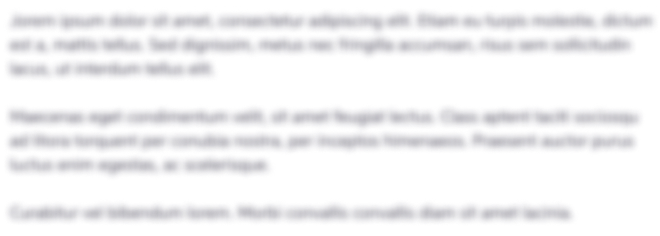
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started