Question
I have an assignment for my computer science class to write a program that would make a game similar to 2048. The methods that need
I have an assignment for my computer science class to write a program that would make a game similar to 2048.
The methods that need to be created are copyRowOrColumn(int rowOrColumn, Direction dir), getCell(int row, int col), setCell(int row, int col, int value), shiftGrid(Direction dir), undo, updateRowOrColumn(int[] arr, int rowOrColumn,Direction dir), and on the GameUtil program it's CalculateTotalScore(int[][] grid), copyGrid(int[][] grid), generateRandomTilePosition(int[][] grid, java.util.Random rand, Direction lastMove), generateRandomTileValue(java.util.Random rand), getScoreForValue(int value), initializeNewGrid(int size, java.util.random), mergeValues(int a, int b), shiftArray(int[] arr)
The constructors are Game(int givenSize, GameUtil givenConfig) and Game(int givenSize, GameUtil givenConfig, java.util.Random givenRandom).
Here is the sample code.
package hw3; import java.util.ArrayList; import java.util.Random;
import api.Direction; import api.Move; import api.TilePosition;
/** * The Game class contains the state and logic for an implementation of a * video game such as "Threes". The basic underlying state is an n by n * grid of tiles, represented by integer values. A zero in a cell is considered * to be * "empty". To play the game, a client calls the method shiftGrid()
, * selecting one of the four directions (LEFT, RIGHT, UP, DOWN). Each row or * column is then shifted according to the rules encapsulated in the * associated GameUtil
object. The move is completed by * calling newTile
, which makes a new tile appear in the grid * in preparation for the next move. *
* In between the call to shiftGrid()
and the call to * newTile
, the client may also call undo()
, which * reverts the grid to its state before the shift. *
* The game uses an instance of java.util.Random to generate new tile values * and to select the location for a new tile to appear. The new values * are generated by the associated GameUtil
's * generateRandomTileValue
method, and the new positions are * generated by the GameUtil
's * generateRandomTilePosition
method. The values are always * generated one move ahead and stored, in order to support the ability * for a UI to provide a preview of the next tile value. *
* The score is the sum over all cells of the individual scores returned by * the GameUtil
's getScoreForValue()
method. */ public class Game { /** * Constructs a game with a grid of the given size, using a default * random number generator. The initial grid is produced by the * initializeNewGrid
method of the given * GameUtil
object. * @param givenSize * size of the grid for this game * @param givenConfig * given instance of GameUtil */ public Game(int givenSize, GameUtil givenConfig) { // just call the other constructor this(givenSize, givenConfig, new Random()); } /** * Constructs a game with a grid of the given size, using the given * instance of Random
for the random number generator. * The initial grid is produced by the initializeNewGrid
* method of the given GameUtil
object. * @param givenSize * size of the grid for this game * @param givenConfig * given instance of GameUtil * @param givenRandom * given instance of Random */ public Game(int givenSize, GameUtil givenConfig, Random givenRandom) { // TODO } /** * Returns the value in the cell at the given row and column. * @param row * given row * @param col * given column * @return * value in the cell at the given row and column */ public int getCell(int row, int col) { // TODO return 0; } /** * Sets the value of the cell at the given row and column. * NOTE: This method should not be used by clients outside * of a testing environment. * @param row * given row * @param col * given col * @param value * value to be set */ public void setCell(int row, int col, int value) { // TODO } /** * Returns the size of this game's grid. * @return * size of the grid */ public int getSize() { // TODO return 0; } /** * Returns the current score. * @return * score for this game */ public int getScore() { // TODO return 0; } /** * Copy a row or column from the grid into a new one-dimensional array. * There are four possible actions depending on the given direction: *
- *
- LEFT - the row indicated by the index
rowOrColumn
is * copied into the new array from left to right * - RIGHT - the row indicated by the index
rowOrColumn
is * copied into the new array in reverse (from right to left) * - UP - the column indicated by the index
rowOrColumn
is * copied into the new array from top to bottom * - DOWN - the row indicated by the index
rowOrColumn
is * copied into the new array in reverse (from bottom to top) *
- *
- LEFT - the given array is copied into the the row indicated by the * index
rowOrColumn
from left to right * - RIGHT - the given array is copied into the the row indicated by the * index
rowOrColumn
in reverse (from right to left) * - UP - the given array is copied into the column indicated by the * index
rowOrColumn
from top to bottom * - DOWN - the given array is copied into the column indicated by the * index
rowOrColumn
in reverse (from bottom to top) *
/** * Plays one step of the game by shifting the grid in the given direction. * Returns a list of Move objects describing all moves performed. All Move * objects must include a valid value for getRowOrColumn()
and * getDirection()
. If no cells are moved, the method returns * an empty list. *
* The shift of an individual row or column is performed by the * method shiftArray
of GameUtil
. *
* The score is not updated. * * @param dir * direction in which to shift the grid * @return * list of moved or merged tiles */ public ArrayList
/** * Reverts the shift performed in a previous call to shiftGrid()
, * provided that neither newTile()
nor undo()
* has been called. If there was no previous call to shiftGrid()
* without a newTile()
or undo()
, * this method does nothing and returns false; otherwise returns true. * @return * true if the previous shift was undone, false otherwise */ public boolean undo() { // TODO return false; } /** * Generates a new tile and places its value in the grid, provided that * there was a previous call to shiftGrid
without a * corresponding call to undo
or newTile
. * The tile's position is determined according * to the generateRandomTilePosition
of this game's * associated GameUtil
object. If there was no previous call to * shiftGrid
without an undo
or newTile
, * this method does nothing and returns null; otherwise returns a * TilePosition
object with the new tiles's position and value. * Note that the returned tile's value should match the current * value returned by getNextTileValue
, and if this method returns * a non-null value the upcoming tile value should be updated according * to generateRandomTileValue()
. This method should * update the total score and the score should include the newly generated tile. * @return * TilePosition containing the new tile's position and value, or null * if no new tile is created */ public TilePosition newTile() { // TODO return null; } /** * Returns the value that will appear on the next tile generated in a call to * newTile
. This is an accessor method that does not modify * the game state. * @return * value to appear on the next generated tile */ public int getNextTileValue() { // TODO return 0; }
}
Here is the GameUtil which will also need to be updated to actually play the game.
package hw3;
import java.util.ArrayList; import java.util.Random; import api.Direction; import api.Move; import api.TilePosition;
/** * Utility class containing some elements of the basic logic for * performing moves in a game of "Threes". */ public class GameUtil {
/** * Constructor does nothing, since this object is stateless. */ public GameUtil() { // do nothing } /** * Returns the result of merging the two given tile values, or zero * if they can't be merged. The rules are: a 1 can be merged with a 2, * and two values greater than 2 can be merged if they match. The * result of a merge is always the sum of the tile values. * @param a * given tile value * @param b * given tile value * @return * result of merging the two values, or zero if no merge is possible */ public int mergeValues(int a, int b) { if (a > 0 && b > 0 && (a + b == 3) || (a >= 3 && b == a)) { return a + b; } else { return 0; } }
/** * Returns the score for a single tile value. Tiles with value less * than 3 have score zero. All other values result from starting with * value 3 and doubling N times, for some N; the score is 3 to the power N + 1. * For example: the value 48 is obtained from 3 by doubling N = 4 * times (48 / 3 is 16, which is 2 to the 4th), so the score is 3 * to the power 5, or 243. * @param value * tile value for which to compute the score * @return * score for the given gile value */ public int getScoreForValue(int value) { // TODO return 0; } /** * Returns a new size x size array with two nonzero cells. * The nonzero cells consist of a 1 and a 2, placed randomly * in the grid using the given Random instance. * @param size * width and height of the new array * @param rand * random number generator to use for positioning the nonzero cells * @return * new size x size array */ public int[][] initializeNewGrid(int size, Random rand) { int[][] grid = new int[size][size]; int numCells = size * size; // To select two distinct cells, think of the numCells cells as ordered // left to right within rows, with the rows ordered top to bottom. // First select two distinct integers between 0 and numCells int first = rand.nextInt(numCells); int second = rand.nextInt(numCells - 1); if (second >= first) { second += 1; } // Then, divide by size to get the row, mod by size to get column, // put a 1 in the first and a 2 in the other grid[first / size][first % size] = 1; grid[second / size][second % size] = 2; return grid; }
/** * Returns the total score for the given grid. The grid * is not modified. * @param grid * given grid * @return * sum of scores for the values in the grid */ public int calculateTotalScore(int[][] grid) { int total = 0; for (int row = 0; row < grid.length; ++row) { for (int col = 0; col < grid[0].length; ++col) { total += getScoreForValue(grid[row][col]); } } return total; } /** * Makes a copy of the given 2D array. The array * must be nonempty and rectangular. * @param grid * given 2D array to copy * @return * copy of the given array */ public int[][] copyGrid(int[][] grid) { int[][] ret = new int[grid.length][grid[0].length]; for (int row = 0; row < grid.length; ++row) { for (int col = 0; col < grid[0].length; ++col) { ret[row][col] = grid[row][col];
Step by Step Solution
There are 3 Steps involved in it
Step: 1
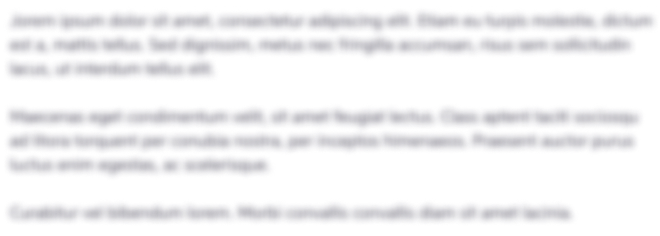
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started