Question
I have been trying to get this code to run in C++. I believe I am close to getting it to do what it needs
I have been trying to get this code to run in C++. I believe I am close to getting it to do what it needs to do. I need to finish making two of the programs in specific, the concatEncoded function and the prepmessage function. I have attempted both but cannot seem to figure it out. Please help! Thank you!
message.txt file says "I had the craziest dream last night"
encoded.txt file says "ITRERLNTHDHCAISDEMATIHAEZTASG"
/*
An Encoder module, consisting of files encoder.h and encoder.cpp,
containing code dealing specifically with encoding a message.
An Encoder module, consisting of files encoder.h and encoder.cpp,
containing code dealing specifically with encoding a message.
There are some functions that are not specifically related to either of these modules, but that contain code needed by them.
You should apportion these functions to the modules in a way consistent with the goals of high cohesion and low coupling.
First step will be to change input from std::cin to taking input from a file called message.txt or
encoded.txt
The given code has two Global Variables. Your finished code should have no Global Variables.
Input
Input to the program is taken from the standard input (cin).
You will need to change this to make your program read a single line of input from the text file message.txt or encoded.txt as appropriate.
Example files are included. The encoded message in encoded.txt was encoded with a key of 3.
You should also test your decoder on messages encoded with other keys.
Output
The program is set to print the result to screen. In addition to printing to the screen, your program should also
print to a file result.txt.
Part A:
Create a project containing the file railFence.cpp.
Implement the empty functions marked with ///Fill This Function comments, remove Global Variables, and ensure correct input and output.
The program should compile and have expected Input and Output as outlined above.
You will not need to split the functions into Modules for this portion.
Part B:
Create a project containing the files railFence.cpp, decoder.cpp, decoder.h, encoder.cpp, and encoder.h.
Split the functions from Part A into the appropriate modules as outlined in the general instructions.
The program should compile and have the same input and output as Part A.
*/
#include
#include
#include "encoder.h"
#include "decoder.h"
#include
std::string msg;
int key;
///Determines the number of letters on each rail, given a key and an encoded message, and separates
///the encoded message into an array of size key, representing the rails. The length of the cycle
///must be determined, along with the size of the remainder representing an unfinished cycle.
void splitEncoded( std::string strarray[]){
int cycle= (2 * key)-2;
int div=cycle;
int len=msg.length();
int remainder=0;
int pos=0;
for(int i=0; i
int counter=((len)/div);
if(cycle!=0 && remainder!=0){
counter= 2*counter;
if((len%div)>=(div-(i-1))){
counter+=2;
}
else if((len%div)>i){
counter++;
}
}
else if(((len)%div)>i){
counter++;
}
strarray[i]=msg.substr(pos,counter);
pos+=counter;
cycle=cycle-2;
remainder+=2;
}
}
///Takes the filled array representing the rails and cycles down and up to
///to retrieve the chars in the correct order, building the decoded
/// message.
std::string concatDecoded( std::string strarray[], int len){
std::string decodedMsg;
int counter=0;
while(counter
for(int i=0; i
if(counter==len){
return decodedMsg;
}
decodedMsg+=strarray[i][0];
if(strarray[i].length()>1)
strarray[i]=strarray[i].substr(1);
else
strarray[i]="";
++counter;
}
for(int i=key-2; i>0; i--){
if(counter==len){
return decodedMsg;
}
decodedMsg+=strarray[i][0];
if(strarray[i].length()>1)
strarray[i]=strarray[i].substr(1);
else
strarray[i]="";
++counter;
}
}
return decodedMsg;
}
///returns a char converted to Uppercase
char toUpperCase (char c)
///FILL THIS FUNCTION: converts char c to UPPER CASE and returns UPPER CASE.
{
if (c == 'a'){
c = 'A';
}
else if(c == 'b'){
c = 'B';
}
else if(c == 'c'){
c = 'C';
}
else if(c == 'd'){
c = 'D';
}
else if(c == 'e'){
c = 'E';
}
else if(c == 'f'){
c = 'F';
}
else if(c == 'g'){
c = 'G';
}
else if(c == 'h'){
c = 'H';
}
else if(c == 'i'){
c = 'I';
}
else if(c == 'j'){
c = 'J';
}
else if(c == 'k'){
c = 'K';
}
else if(c == 'l'){
c = 'L';
}
else if(c == 'm'){
c = 'M';
}
else if(c == 'n'){
c = 'N';
}
else if(c == 'o'){
c = 'O';
}
else if(c == 'p'){
c = 'P';
}
else if(c == 'q'){
c = 'Q';
}
else if(c == 'r'){
c = 'R';
}
else if(c == 's'){
c = 'S';
}
else if(c == 't'){
c = 'T';
}
else if(c == 'u'){
c = 'U';
}
else if(c == 'v'){
c = 'V';
}
else if(c == 'w'){
c = 'W';
}
else if(c == 'x'){
c = 'X';
}
else if(c == 'y'){
c = 'Y';
}
else if(c == 'z'){
c = 'Z';
}
return c;
};
///Return true if char is alpha numeric
bool isAlphanumeric (char c)
{
///FILL THIS FUNCTION: evaluates char c, returns true if c is Alphanumeric (0-9, A-Z, a-z)
if(c== 'A' || c== 'a' || c== 'E' || c== 'i' ||c== 'M' || c== 'm' ||c== 'Q' || c== 'q' ||c== 'U' || c== 'u' ||c== 'Y' || c== 'y' ||
c== 'B' || c== 'b' ||c== 'F' || c== 'j' ||c== 'N' || c== 'n' ||c== 'R' || c== 'r' ||c== 'V' || c== 'v' ||c== 'Z' || c== 'z' ||c== '2' || c== '5' ||c== '8' ||
c== 'C' || c== 'c' ||c== 'G' || c== 'k' ||c== 'O' || c== 'o' ||c== 'S' || c== 's' ||c== 'W' || c== 'w' ||c== 'A' || c== '0' ||c== '3' || c== '6' ||c== '9' ||
c== 'D' || c== 'd' ||c== 'H' || c== 'l' ||c== 'P' || c== 'p' ||c== 'T' || c== 't' ||c== 'X' || c== 'x' ||c== 'A' || c== '1' ||c== '4' || c== '7'){
return true;
}
else{
return false;
}
return true;///this is just a place holder for your return statement.
};
///Given an uncoded message and an array representing the rails
///cycles down and up the rails, filling the array until message ends
void splitMessage( std::string strarray[]){
int counter=0;
while(counter
for(int i=0; i
strarray[i]+= msg[counter];
++counter;
if(counter==msg.length())
return;
}
for(int i=key-2; i>0; i--){
strarray[i]+= msg[counter];
++counter;
if(counter==msg.length())
return;
}
}
};
///given a filled array representing the rails, concatenates the
///strings on the rails and returns a coded message
std::string concatEncoded(std::string strarray[], int szearray)
// if (key == 3){
// for(){
// cout << strarray[0] << "..." << strarray[]
///FILL THIS FUNCTION: given an array of strings representing the rails,
///and the size of the array,
///concatenate the strings into a single string representing the encoded message and
///return it.
return "this is an encoded message";///this is just a place holder for your return statement
}
///given a message, formats the message for encoding or decoding
void prepmessage(std::string strarray[], std::string& simplifiedMessage){
int arraySize = sizeof(strarray);
char c;
char c1;
for(int i=0; i
{
c1 = static_cast(strarray[i]);
cerr << strarray[i];
c1 = static_cast(strarray[i]);
c1 = char(strarray[i]);
c = touppercase(c1);
// c = toUpperCase(strarray[i]);
if(isAlphanumeric(strarray[i]))
sinplifiedMessage += strarray[i];
}
/*
for(int i =0; i
isAlphanumeric(strarray[i]);
}
for(int i =0; i
toUpperCase(strarray[i]);
}
for(int i=0; i)
*/
///FILL THIS FUNCTION: given a message, converts message to all
///uppercase and removes non-alphanumeric characters including spaces
///and punctuation. Notice this is a VOID function!!!
}
///makes functions calls for encoding a message
void encodeMessage(){
prepmessage();
std::string encoded[key];//an array representing the rails
splitMessage(encoded);
msg=concatEncoded(encoded);
};
///makes function calls to decode a coded message
void decodeMessage(){
std::string decoded[key];//an array representing the rails
splitEncoded(decoded);
msg=concatDecoded( decoded, msg.length());
}
///**********************************************
///EVERYTHING BELOW THIS LINE REMAINS IN RAILFENCE.CPP
///**********************************************
///reads message from file stream in
std::string readMessage(std::istream& in)
{
std::string inmsg;
std::string line;
getline (in, line);
while (in)
{
inmsg = inmsg + line + " ";
getline (in, line);
}
std::cout << inmsg << std::endl;
return inmsg;
}
///given an out stream, prints the message
void printMessage(std::ostream& out){
out<
out<
}
///promps the user for a KEY 2-5
int getKey(){
int newkey;
while(true){
std::cout<<"Select a Key (2-5): ";
if(std::cin>>newkey){
if(newkey>1 && newkey<=5)
break;
}
std::cout<<" Please enter a valid key!! ";
std::cin.ignore();
}
std::cin.ignore();
return key;
};
///prints a menu and asks the user for a selection
char getselection(){
std::string selection="0";
std::cin.clear();
while(selection[0]<'1' || selection[0]>'3'){
std::cout << " *****Welcome to Rail Fence Coder***** Menu: "
<< "\t1 - encrypt a file "
<< "\t2 - decrypt a file "
<< "\t3 - quit "
<<"Selection: ";
getline(std::cin, selection);
if(selection[0]<'1' || selection[0]>'3'){
std::cout<<" Please enter a valid selection!!"<
}
}
return selection[0];
}
int main()
{
bool quit=false;
std::ifstream inmessage("message.txt");
std::ifstream incoded("encoded.txt");
while(!quit){
char select=getselection();
switch(select){
case '1':
key = getKey();
msg=readMessage(inmessage);
printMessage(std::cout);
encodeMessage();
printMessage(std::cout);
break;
case '2':
key = getKey();
msg=readMessage(incoded);
printMessage(std::cout);
prepmessage();
decodeMessage();
printMessage(std::cout);
break;
case '3':
std::cout<<" Exiting Rail Fence Coder "<
quit=true;
}
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
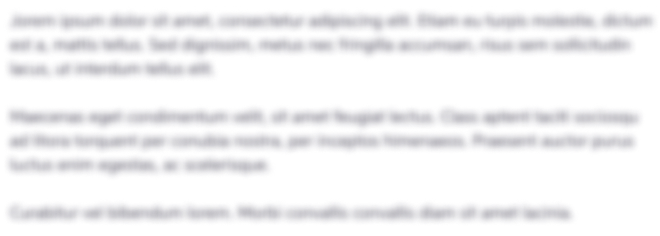
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started