Question
I have code called HashTable.py and need to write a program to read in data from the 6degrees.csv file and store it to allow fast
I have code called HashTable.py and need to write a program to read in data from the 6degrees.csv file and store it to allow fast retrieval of movies, actors and movie roles. Your user interface should ask for the type of entry to retrieve and the string to search for. Along with the query result, it should also give a query time measurement.
HashTable code is below:
import numpy as np import math
class Table(): # Inner class Entry class Entry(): #0 = never used/free, 1 = used / not free
def __init__(self, inKey="", value=None): self.key = inKey self.value = value if self.key == "": self.state = 0 else: self.state = 1 # End inner class def __init__(self, tableSize):
self.actualSize = self.nextPrime(tableSize - 1); self.hashArray = np.zeros(self.actualSize, dtype=object)
for i in range(0, self.actualSize): self.hashArray[i] = self.Q4HashEntry() self.hashCount = 0 def put(self, inKey, inValue): hashIdx = self.hash(inKey) initIdx = hashIdx i = 1
while (self.hashArray[hashIdx] != None and not self.hashArray[hashIdx].key == inKey): if ( not self.hashArray[hashIdx].key == inKey): if (self.hashArray[hashIdx].state == 1): hashIdx = (initIdx + i) % len(self.hashArray) if (self.hashArray[hashIdx].state < 1): self.hashArray[hashIdx] = self.Q4HashEntry(inKey, inValue) self.hashCount = self.hashCount + 1 i += 1
def getLoadFactor(self): loadFactor = self.hashCount / len(self.hashArray)
return loadFactor
def display(self): for i in range(0, len(self.hashArray)): if (self.hashArray[i].value != None): print("\t\t" + str(i) + "\t" + str(self.hashArray[i].key)) def hash(self, inKey): hashIdx = 0 for i in range(0, len(inKey)): hashIdx = hashIdx + ord(inKey[i]) retVal = hashIdx % len(self.hashArray) return retVal
def nextPrime(self, inNum):
isPrime = False
if (inNum % 2 == 0): prime = inNum - 1 else: prime = inNum
while (not isPrime): prime = prime + 2 i = 3 isPrime = True rootVal = math.sqrt(prime) while ((i <= rootVal) and (isPrime)): if ((prime % i) == 0): isPrime = False else: i = i + 2 return prime
def getArrayLength(self): return len(self.hashArray)
6degrees CSV:
Movie | Year | Actor | Role |
---|---|---|---|
Cool Surfings | 1997 | John Smith | James |
Cool Surfings | 1997 | Emily Willis | Kate |
Cool Surfings | 1997 | Will Moore | Will |
Cool Surfings | 1997 | Jaylin Green | Chef |
Cool Surfings | 1997 | Lara Moore | Cleaner |
Browning | 2002 | Owen Young | Hotel Guest |
Browning | 2002 | Zac Jill | Serena |
Browning | 2002 | Paul Harry | Katie |
PokuPoke | 2010 | John Cole | Grandma |
PokuPoke | 2010 | Joe Cole | Grandad |
PokuPoke | 2010 | Aaron Ivey | Clown |
PokuPoke | 2010 | Kris Jook | Skater |
PokuPoke | 2010 | Jalen Sha | Christina |
Rest of This | 2015 | Kayla Brat | Natalie |
Rest of This | 2015 | Jaimee Lee | BoBo |
Rest of This | 2015 | Gorden Green | Achu |
Rest of This | 2015 | Peter Blue | Mann |
Walk | 2017 | Fred Purple | Grimes |
Walk | 2017 | Whitney White | Joneseson |
Walk | 2017 | Virgil Violet | Mason |
Walk | 2017 | John Harvey | Bull |
Walk | 2017 | Bo Love | Lilly |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
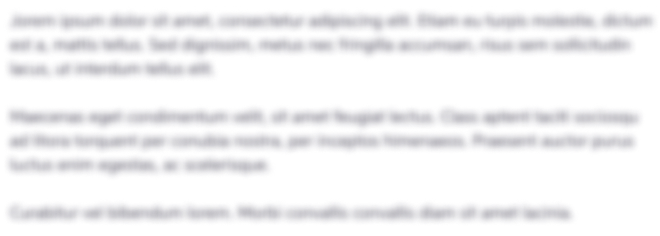
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started