Question
I have completed TASK 1. Update TODO TASK 2 in javascript code below to answer the question. Please DO NOT copy and paste another answer:
I have completed "TASK 1". Update "TODO TASK 2" in javascript code below to answer the question. Please DO NOT copy and paste another answer:
function makeItem(type, data) { var itemObject = { type: type, data: data }; return JSON.stringify(itemObject); }
function addSection(key, entryElement) { // Create a section element to contain the new entry var sectionElement = document.createElement("section");
// Give the section a class to allow styling sectionElement.classList.add("entry");
// Add the element to the section sectionElement.appendChild(entryElement);
// Create a button to delete the entry var deleteButton = document.createElement("button"); deleteButton.innerHTML = "×"; deleteButton.setAttribute("aria-label", "Delete entry");
// Create an event listener to delete the entry function deleteEntry() { console.log("deleteEntry called with variables in scope:", { key, entryElement, sectionElement, deleteButton, });
// Remove the section from the page sectionElement.parentNode.removeChild(sectionElement); localStorage.removeItem(key);
}
// Connect the event listener to the button 'click' event deleteButton.addEventListener("click", deleteEntry);
// Add the delete button to the section sectionElement.appendChild(deleteButton);
// Get a reference to the element containing the diary entries var diaryElement = document.querySelector("main");
// Get a reference to the first button section (Add entry/photo) in the diary element var buttonElement = diaryElement.querySelector("section.button");
// Add the section to the page after existing entries, // but before the buttons diaryElement.insertBefore(sectionElement, buttonElement); }
function addTextEntry(key, initialText, isNewEntry) { // Create a textarea element to edit the entry var textareaElement = document.createElement("textarea"); textareaElement.rows = 5; textareaElement.placeholder = "(new entry)";
// Set the textarea's value to the given text (if any) textareaElement.value = initialText;
// Add a section to the page containing the textarea addSection(key, textareaElement);
// If this is a new entry (added by the user clicking a button) // move the focus to the textarea to encourage typing if (isNewEntry) { textareaElement.focus(); }
function saveEntry() { console.log("saveEntry called with variables in scope:", { key, initialText, isNewEntry, textareaElement, });
var text = textareaElement.value; var item = makeItem("text", text); localStorage.setItem(key, item); }
textareaElement.addEventListener('change', saveEntry); }
/** * Add an image entry to the page * @param key Key of item in local storage * @param data Data URL of image data */ function addImageEntry(key, data) { // Create a image element var imgElement = new Image(); imgElement.alt = "Photo entry";
// Load the image imgElement.src = data;
// Add a section to the page containing the image addSection(key, imgElement); }
/** * Function to handle Add text button 'click' event */ function addEntryClick() { // Add an empty text entry, using the current timestamp to make a key var key = "diary" + Date.now(); var initialText = ""; var isNewEntry = true; addTextEntry(key, initialText, isNewEntry); }
/** * Function to handle Add photo button 'click' event */ function addPhotoClick() { // Trigger the 'click' event of the hidden file input element // (as demonstrated in Block 3 Part 4) var inputElement = document.querySelector("#image input"); inputElement.click(); }
/** * Function to handle file input 'change' event * @param inputChangeEvent file input 'change' event data */ function processFile(inputChangeEvent) { console.log("processFile called with arguments:", { inputChangeEvent, });
// Create a function to add an image entry using a data URL function addImage(data) { console.log("addImage called with arguments:", { data, });
// Add a new image entry, using the current timestamp to make a key var key = "diary" + Date.now(); addImageEntry(key, data);
// TODO: Q1(c)(iv) Task 1 of 2 // Make an image item using the given data URL // (demonstrated elsewhere in this file) // Store the item in local storage using the given key // (demonstrated elsewhere in this file) var imageData = data; var item = makeItem("image", imageData); localStorage.setItem(key, item); }
// Add a 'dummy' image entry addImage(window.DUMMY_DATA_URL);
// TODO: Q1(c)(iv) Task 2 of 2 // Complete this function to read a file when it is selected: // (reading files into a data URL using FileReader is demonstrated in Block 3 Part 4) // (imgElement and messageElement do not exist in this app so remove that code) // ...then call addImage using the data URL you obtain // ...and comment out line above using window.DUMMY_DATA_URL
// Clear the file selection (allows selecting the same file again) inputChangeEvent.target.value = ""; }
/** * Show the diary items in local storage as diary entries on the page */ function showEntries() { console.log("Adding items from local storage to the page");
// Build a sorted list of keys for diary items var diaryKeys = [];
// Loop through each key in storage by index for (var index = 0; index
// If the key begins with "diary", assume it is for a diary entry // There may be other data in local storage, so we will ignore that if (key.slice(0, 5) == "diary") { // Append this key to the list of known diary keys diaryKeys.push(key); } }
// Although browser developer tools show items in key order, // their actual order is browser-dependent, so sort the keys, // so that our diary entries are shown in the right order! diaryKeys.sort();
// Loop through each diary item in storage by key for (var index = 0; index
// Assume the item is a JSON string and decode it var item = JSON.parse(localStorage.getItem(key));
if (item.type == "text") { // Assume the data attribute is text addTextEntry(key, item.data, false); } else if (item.type == "image") { // Assume the data attribute is an image URL addImageEntry(key, item.data); } else { // This should never happen - show an error and give up console.error("Unexpected item: " + item); } } }
/** * Function to connect event listeners and start the application */ function initialize() { // A rough check for local storage support if (!window.localStorage) { // This check is not 100% reliable, but is sufficient for our demo, see e.g. // https://developer.mozilla.org/en-US/docs/Web/API/Web_Storage_API/Using_the_Web_Storage_API#Testing_for_availability
// This could be more elegant too, but is sufficient for our demo document.querySelector("main").outerHTML = "
Error: localStorage not supported!
";// Stop the demo return; }
// Connect the Add entry and Add photo button 'click' events var addEntryButton = document.querySelector("#text button"); var addPhotoButton = document.querySelector("#image button"); addEntryButton.addEventListener("click", addEntryClick); addPhotoButton.addEventListener("click", addPhotoClick);
// Connect the input file 'change' event listener // (note this may not trigger if you repeatedly select the same file) var inputElement = document.querySelector("#image input"); inputElement.addEventListener("change", processFile);
// Create some demonstration items createDemoItems();
// Update the page to reflect stored items showEntries(); }
// Connect event listeners and start the application when the page loads window.addEventListener("load", initialize);
Edit the script in the places indicated in 'tma03.js' to read data from the chosen image and store this as image item in local storage. (9 marks) When the 'Add photo' button is clicked, the demonstration application allows users to choose an image file (or take a photo on a mobile device), then adds an image entry to the page using 'dummy' image data. The image is not saved. The prototype application should instead use the image chosen by the guest, and save this as an image item. There is a hidden image file input element in the page to allow a file to be chosen, but its event data is not used. Add or edit JavaScript code, where indicated, to achieve the following: - Task 1: when an image entry is added to the DOM, make an image item containing the image data, and store it in local storage. - Task 2: when the file input element selection changes, use a FileReader object to convert the file to a data URL and add an image entry to the DOM using this data URL. In your solution document, provide your amended JavaScript code. Making and testing this change There are two main outcomes here: for a user to be able to add an image of their choice to the page, not the dummy image every time (Tasks 2), but first to ensure that this data is saved to local storage, in a similar way as you have done for text entries (Task 1)Step by Step Solution
There are 3 Steps involved in it
Step: 1
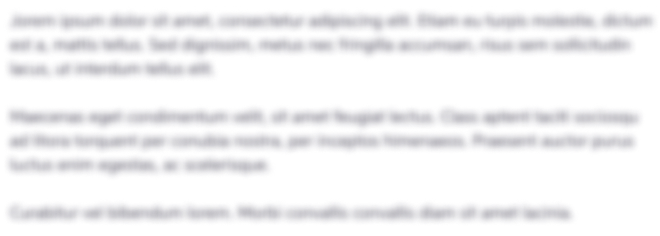
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started