Question
I have done most of the functions in this program. Can someone do the LAST 2 functions please? The code needs to be in C
I have done most of the functions in this program. Can someone do the LAST 2 functions please? The code needs to be in C
#include
#define MAX_STR_LEN 1024
typedef struct MovieReview_struct { } MovieReview;
typedef struct ReviewNode_struct { char movie_title[MAX_STR_LEN]; char movie_studio[MAX_STR_LEN]; int year; float BO_total; int score; struct ReviewNode_struct *next; } ReviewNode;
ReviewNode *newMovieReviewNode(); ReviewNode *findMovieReview(char title[MAX_STR_LEN], char studio[MAX_STR_LEN], int year, ReviewNode *head); ReviewNode *insertMovieReview(char title[MAX_STR_LEN], char studio[MAX_STR_LEN], int year, float BO_total, int score, ReviewNode *head);
ReviewNode *newMovieReviewNode() { /* * This function allocates a new, empty ReviewNode, and initializes the * contents of the MovieReview for this node to empty values. * The fields in the MovieReview should be set to: * movie_title="" * movie_studio="" * year = -1 * BO_total = -1 * score = -1 * * The *next pointer for the new node MUST be set to NULL * * The function must return a pointer to the newly allocated and initialized * node. If something goes wrong, the function returns NULL */ ReviewNode *newNode; newNode = (ReviewNode*)malloc(sizeof(ReviewNode)); strcpy(newNode->movie_title, ""); strcpy(newNode->movie_studio, ""); newNode->year = -1; newNode->BO_total = -1; newNode->score = -1; return newNode; // <--- This should change when after you implement your solution }
ReviewNode *findMovieReview(char title[MAX_STR_LEN], char studio[MAX_STR_LEN], int year, ReviewNode *head) { /* * This function searches through the linked list for a review that matches the input query. * In this case, the movie review must match the title, studio, and year provided in the * parameters for this function. * * If a review matching the query is found, this function returns a pointer to the node that * contains that review. * * If no such review is found, this function returns NULL */ ReviewNode *cur = head; while (cur != NULL) { if (strcmp(title, cur->movie_title) == 0 && strcmp(studio, cur->movie_studio)== 0 && year == cur->year) { return cur; } cur = cur->next; } return NULL; // <--- This should change when after you implement your solution }
ReviewNode *insertMovieReview(char title[MAX_STR_LEN], char studio[MAX_STR_LEN], int year, float BO_total, int score, ReviewNode *head) { /* * This function inserts a new movie review into the linked list. * * The function takes as input parameters the data neede to fill-in the review, * as well as apointer to the current head of the linked list. * * If head==NULL, then the list is still empty. * * The function inserts the new movie review *at the head* of the linked list, * and returns the pointer to the new head node. * * The function MUST check that the movie is not already in the list before * inserting (there should be no duplicate entries). If a movie with matching * title, studio, and year is already in the list, nothing is inserted and the * function returns the current list head. */ ReviewNode *cur = head, *newNode; if (head == NULL) { //insert at head newNode = newMovieReviewNode(); strcpy(newNode->movie_title, title); strcpy(newNode->movie_studio, studio); newNode->year = year; newNode->score = score; newNode->BO_total = BO_total; newNode->next = NULL; head = newNode; return head; } //check if movie is already in list , if not then insert, CHEGGEA if (findMovieReview(title, studio, year, head) != NULL) { return cur; } while (cur->next != NULL) cur = cur->next; newNode = newMovieReviewNode(); strcpy(newNode->movie_title, title); strcpy(newNode->movie_studio, studio); newNode->year = year; newNode->score = score; newNode->BO_total = BO_total; newNode->next = NULL; cur->next = newNode; return head; // <--- This should change when after you implement your solution }
int countReviews(ReviewNode *head) { /* * This function returns the length of the current linked list. This requires * list traversal. */
int count = 0; // Count Variable ReviewNode *cur = head; // Iterate through the node and count the length. while (cur != NULL) { count++; cur = cur->next; } return count; // <--- This should change when after you implement your solution }
void updateMovieReview(char title[MAX_STR_LEN], char studio[MAX_STR_LEN], int year, float BO_total, int score, ReviewNode *head) { /* * This function looks for a review matching the input query [title, studio, year]. * If such a review is found, then the function updates the Box-office total, and the score. * If no such review is found, the function prints out * "Sorry, no such movie exists at this point" */
ReviewNode *cur;
// Find the current node cur = findMovieReview(title, studio, year, head); // Update the current node values if found, else print the message if ( cur != NULL) { cur->BO_total = BO_total; cur->score = score; } else { printf("Sorry, no such movie exists at this point"); } }
ReviewNode *deleteMovieReview(char title[MAX_STR_LEN], char studio[MAX_STR_LEN],int year, ReviewNode *head) { /* * This function removes a review matching the input query from the linked list. If no such review can * be found, it does nothing. * * The function returns a pointer to the head of the linked list (which may have changed as a result * of the deletion process) prev -> Holds the previous node cur -> Holds the current node */ ReviewNode *prev, *cur;
// Initialize the nodes cur = head; prev = head;
// Iterate though the node to find the matching node and delete the current node while (cur != NULL) { if (strcmp(title, cur->movie_title) == 0 && strcmp(studio, cur->movie_studio) == 0 && year == cur->year) { // If current node is the head node, that is, if it is first node in the list if (cur == head) { cur = cur->next; return cur; } // If not first node, assign the previous node to next node of current node prev->next = cur->next; cur->next = NULL; // Delink current node from the list return head; } prev = cur; // Hold the current node as previous node cur = cur->next; // Traverse to next node }
return head; // <--- This should change when after you implement your solution }
void printMovieReviews(ReviewNode *head) { /* * This function prints out all the reviews in the linked list, one after another. * Each field in the review is printed in a separate line, with *no additional text* * (that means, the only thing printed is the value of the corresponding field). * * Reviews are separated from each other by a line of * "*******************" * * See the Assignment handout for a sample of the output that should be produced * by this function */ while(head!=NULL){
printf("%s %s %d %0.6f %d ",head->movie_title,head->movie->studio,head->year,head->BO_total,head->score);
printf("***********************");
head=nead->next;
} }
void queryReviewsByStudio(char studio[MAX_STR_LEN], ReviewNode *head) { /* * This function looks for reviews whose studio matches the input query. * It prints out the contents of all reviews matching the query in exactly * the same format used by the printMovieReviews() function above. */ while(head!=NULL){
if(strcmp(studio,head->movie_studio)==0){
printf("%s %s %d %0.6f %d ",head->movie_title,head->movie->studio,head->year,head->BO_total,head->score);
printf("***********************");
}
head=nead->next;
} }
void queryReviewsByScore(int min_score, ReviewNode *head) { /* * This function looks for reviews whose score is greater than, or equal to * the input 'min_score'. * It prints out the contents of all reviews matching the query in exactly * the same format used by the printMovieReviews() function above. */ /***************************************************************************/ /********** TO DO: Complete this function *********************************/ /***************************************************************************/ if(head==NULL) return;
while(head!=NULL){
if(head->score>=min_score){
printf("%s %s %d %0.6f %d ",head->movie_title,head->movie->studio,head->year,head->BO_total,head->score);
printf("***********************");
}
head=nead->next;
} }
ReviewNode *deleteReviewList(ReviewNode *head) { /* * This function deletes the linked list of movie reviews, releasing all the * memory allocated to the nodes in the linked list. * * Returns a NULL pointer so that the head of the list can be set to NULL * after deletion. */ /***************************************************************************/ /********** TO DO: Complete this function *********************************/ /***************************************************************************/
return NULL; // <--- This should change when after you implement your solution }
ReviewNode *sortReviewsByTitle(ReviewNode *head) { /* * This function sorts the list of movie reviews in ascending order of movie * title. If duplicate movie titles exist, the order is arbitrary (i.e. you * can choose which one goes first). * * However you implement this function, it must return a pointer to the head * node of the sorted list. */
/***************************************************************************/ /********** TO DO: Complete this function *********************************/ /***************************************************************************/
return NULL; // <--- This should change when after you implement your solution }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
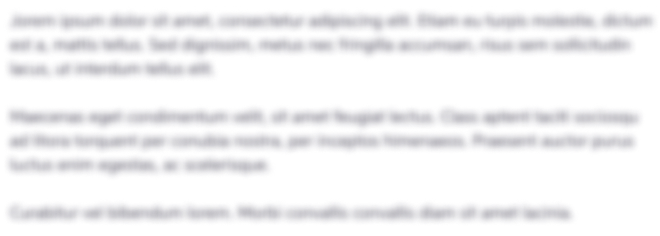
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started