Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I have implemented the diffie - hellman key exchange, Vigenere cipher, and the Playfair cipher algorithms in python and they all worked well. Now I
I have implemented the diffiehellman key exchange, Vigenere cipher, and the Playfair cipher algorithms in python and they all worked well. Now I need to combine them to make a three layer security program but I am having issues with that. Can someone help connocting the codes together? Here is th diffiehellman code: #Following the steps of DiffieHellman Key Exchange Algorithm #Step : All users agree on glodabl prime parameters q and g q intinput Please enter first prime number q: g intinput Please enter second prime number g: #Step : Each user selects random secret key senderkey intinput enter sender public key must be greater than strg: receiverkey intinput enter receiver public key must be greater than strg: #Step : Each user computes respective public keys Ys gXs mod q and Yr gXr mod q senderPublic gsenderkey q receiverPublic greceiverkey q #Step : Compute shared session key Ksr gXsXr mod q #YsXr mod q which receiver can compute, and YrXs mod q which sender can compute senderShared receiverPublicsenderkey q receiverShared senderPublicreceiverkey q print The public key Sender: strsenderPublic print The public key Receiver: strreceiverPublic print The shared key Sender: strsenderShared print The shared key Receiver: strreceiverShared print Here is the vigenere code: alphabet "abcdefghijklmnopqrstuvwxyz lettertoindex dictzipalphabet rangelenalphabet indextoletter dictziprangelenalphabet alphabet # For the decryption proccess add the key letter's index to the message letter's index def encryptmessage key: encrypted splitmessage messagei : i lenkey for i in range lenmessage lenkey for eachsplit in splitmessage: i for letter in eachsplit: number lettertoindexletter lettertoindexkeyi lenalphabet encrypted indextoletternumber i return encrypted # For the decryption proccess subtract the key letter's index from the message letter's index def decryptencryptedmessage, key: decrypted splitmessage encryptedmessagei : i lenkey for i in range lenencryptedmessage lenkey for eachsplit in splitmessage: i for letter in eachsplit: number lettertoindexletter lettertoindexkeyi lenalphabet decrypted indextoletternumber i return decrypted def main: message "i love information security" key "subject" encryptedmessage encryptmessage key decryptedmessage decryptencryptedmessage, key print Original message: message print Encrypted message: encryptedmessage print Decrypted message: decryptedmessage print main And here is the playfair code: import string import itertools def chunkerseq size: it iterseq while True: chunk tupleitertoolsisliceit size if not chunk: return yield chunk def prepareinputdirty: dirty joincupper for c in dirty if c in string.asciiletters clean if lendirty: return dirty for i in rangelendirty: clean dirtyi if dirtyi dirtyi : clean X clean dirty if lenclean & : clean X return clean def generatetablekey: alphabet "ABCDEFGHIKLMNOPQRSTUVWXYZ" table for char in key.upper: if char not in table and char in alphabet: table.appendchar for char in alphabet: if char not in table: table.appendchar return table def encodeplaintext key: table generatetablekey plaintext prepareinputplaintext ciphertext for char char in chunkerplaintext: row col divmodtableindexchar row col divmodtableindexchar if row row: ciphertext tablerowcol ciphertext tablerowcol elif col col: ciphertext tablerow col ciphertext tablerow col else: ciphertext tablerow col ciphertext tablerow col return ciphertext
I have implemented the diffiehellman key exchange, Vigenere cipher, and the Playfair cipher algorithms in python and they all worked well. Now I need to combine them to make a three layer security program but I am having issues with that. Can someone help connocting the codes together?
Here is th diffiehellman code:
#Following the steps of DiffieHellman Key Exchange Algorithm
#Step : All users agree on glodabl prime parameters q and g
q intinput
Please enter first prime number q:
g intinput
Please enter second prime number g:
#Step : Each user selects random secret key
senderkey intinput
enter sender public key must be greater than strg:
receiverkey intinput
enter receiver public key must be greater than strg:
#Step : Each user computes respective public keys Ys gXs mod q and Yr gXr mod q
senderPublic gsenderkey q
receiverPublic greceiverkey q
#Step : Compute shared session key Ksr gXsXr mod q
#YsXr mod q which receiver can compute, and YrXs mod q which sender can compute
senderShared receiverPublicsenderkey q
receiverShared senderPublicreceiverkey q
print
The public key Sender: strsenderPublic
print
The public key Receiver: strreceiverPublic
print
The shared key Sender: strsenderShared
print
The shared key Receiver: strreceiverShared
print
Here is the vigenere code:
alphabet "abcdefghijklmnopqrstuvwxyz
lettertoindex dictzipalphabet rangelenalphabet
indextoletter dictziprangelenalphabet alphabet
# For the decryption proccess add the key letter's index to the message letter's index
def encryptmessage key:
encrypted
splitmessage
messagei : i lenkey for i in range lenmessage lenkey
for eachsplit in splitmessage:
i
for letter in eachsplit:
number lettertoindexletter lettertoindexkeyi lenalphabet
encrypted indextoletternumber
i
return encrypted
# For the decryption proccess subtract the key letter's index from the message letter's index
def decryptencryptedmessage, key:
decrypted
splitmessage
encryptedmessagei : i lenkey for i in range lenencryptedmessage lenkey
for eachsplit in splitmessage:
i
for letter in eachsplit:
number lettertoindexletter lettertoindexkeyi lenalphabet
decrypted indextoletternumber
i
return decrypted
def main:
message "i love information security"
key "subject"
encryptedmessage encryptmessage key
decryptedmessage decryptencryptedmessage, key
print
Original message: message
print
Encrypted message: encryptedmessage
print
Decrypted message: decryptedmessage
print
main
And here is the playfair code:
import string
import itertools
def chunkerseq size:
it iterseq
while True:
chunk tupleitertoolsisliceit size
if not chunk:
return
yield chunk
def prepareinputdirty:
dirty joincupper for c in dirty if c in string.asciiletters
clean
if lendirty:
return dirty
for i in rangelendirty:
clean dirtyi
if dirtyi dirtyi :
clean X
clean dirty
if lenclean & :
clean X
return clean
def generatetablekey:
alphabet "ABCDEFGHIKLMNOPQRSTUVWXYZ"
table
for char in key.upper:
if char not in table and char in alphabet:
table.appendchar
for char in alphabet:
if char not in table:
table.appendchar
return table
def encodeplaintext key:
table generatetablekey
plaintext prepareinputplaintext
ciphertext
for char char in chunkerplaintext:
row col divmodtableindexchar
row col divmodtableindexchar
if row row:
ciphertext tablerowcol
ciphertext tablerowcol
elif col col:
ciphertext tablerow col
ciphertext tablerow col
else:
ciphertext tablerow col
ciphertext tablerow col
return ciphertext
Step by Step Solution
There are 3 Steps involved in it
Step: 1
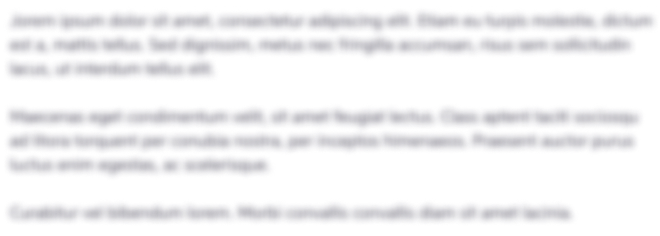
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started