Question
I have the following java classes that are meant to find and open a text file Date.txt, and output to a GUI the sorted and
I have the following java classes that are meant to find and open a text file Date.txt, and output to a GUI the sorted and unsorted results. The sorted goes in the right column and the unsorted in the left. The sorted output is meant to read a text in the format 20141001 and output in the format October 01, 2014. I can get it to sort, but not output in the required format. I will provide the codes I have.
/*Date212 checks parameters
* of input text to
* see if requirements are met.
*/
public class Date212 {
private String monthsInYear[]=
{"","January","February",
"March","April","May",
"June","July","August",
"September","October",
"November","December"};
private String monthString;
private int day;//Variable day set to private. Can't be accessed or altered by user.
private int month;//Variable month set to private. Can't be accessed or altered by user.
private int year;//Variable year set to private. Can't be accessed or altered by user.
public Date212(int y, int m, int d) {
year = y;
month = m;
day = d;
monthString = monthsInYear[m];
}
public int getDay() {
return day;
}
public int getMonth() {
return month;
}
public int getYear() {
return year;
}
public void setDay(int d) {
day=d;
}
public void setMonth(int m) {
month=m;
}
public void setYear(int y) {
year=y;
}
public Date212 (String date)
{
/*Calling three argument constructor
* Substring reads the data from the textfile
* and separates them, with years being the first
* 4 numbers, months being the fifth and sixth numbers,
* and days being the seventh and eighth numbers.
*/
this(Integer.parseInt(date.substring(0, 4)), Integer.parseInt(date.substring(4, 6)), Integer.parseInt(date.substring(6, 8)));
}
public String getAsString()
{
return monthString + " " + String.format("%02d", day) + ", " + String.format("%04d", year);
}
/*
* Returns the string representation of the Date212 object
*/
public String toString() {
String date = "";
date += + this.year;
date += this.month < 10 ? "0" + this.month : this.month;
date += this.day < 10 ? "0" + this.day : this.day;
return date;
}
public int compareTo(Date212 other) {
if (this.year < other.year) {
return -1;
} else if (this.year > other.year) {
return 1;
}
if (this.month < other.month) {
return -1;
} else if (this.month > other.month) {
return 1;
}
if (this.day < other.day) {
return -1;
} else if (this.day > other.day) {
return 1;
}
return 0;
}
}
________________________________________________________________________
public class Project2 {
public static void main(String[] args) {
DateGui gui = new DateGui();
}
}
_________________________________________________________________________________________
/* This class displays
* the dates entered through a text file
* and displays the sorted and unsorted dates
* on a one row, two column GUI. */
import javax.swing.*;
import java.awt.GridLayout;
import java.awt.TextArea;
public class DateGui extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
TextArea unsortedDates, sortedDates;
public DateGui() {
setTitle("Project 2");//Displays the title of the project.
setSize(400, 400);//Sets the size of the GUI ContentPane to an appropriate width and length.
setLocation(100, 100);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
unsortedDates = new TextArea("Unsorted: " + " ");
sortedDates = new TextArea("Sorted: " + " ");//Allows
setLayout(new GridLayout(1, 2)); // Creates the 1 row, 2 column layout
getContentPane().add(unsortedDates);//Allows the unsorted text to be displayed on the left column.
getContentPane().add(sortedDates);//Allows the sorted text to be displayed on the right column.
createFileMenu();
setVisible(true);
}
private void createFileMenu() {
JMenu fileMenu = new JMenu("File"); //creates drop-down menu, File
JMenuBar menuBar = new JMenuBar();
JMenuItem item = new JMenuItem("Open"); //Creates drop-down menu option, open.
FileMenuHandler fmh = new FileMenuHandler(this);
item.addActionListener(fmh);
fileMenu.add(item);
fileMenu.addSeparator(); //Separates Open and Quit on the drop-down menu.
item = new JMenuItem("Quit"); //Creates drop-down menu option, Quit.
item.addActionListener(fmh);
fileMenu.add(item);
setJMenuBar(menuBar);
menuBar.add(fileMenu);
}
}
_________________________________________________________________________________________
//abstract linkedlist class
//extends to UnsortedDateList and SortedDateList
public abstract class DateList {
protected DateNode first;//First node in linked list
protected DateNode last;//Last node in linked list
protected DateNode d;
protected int length;//Number of data items in the list
public abstract void add(Date212 date);
public String toString() {
String listOfDates = "";
d = first.next;
while (d != null) {
listOfDates += d.date.toString() + " ";
d = d.next;
}
return listOfDates;
}
}
______________________________________________________________________________________________________
//nodes for linkedlists
public class DateNode {
protected Date212 date;
protected DateNode next;
public DateNode(Date212 date) {
this.date = date;
this.next = null;
}//constructor
}
__________________________________________________________________________________
//This class handles the File menu drop-down list of the GUI.
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.*;
import javax.swing.JFileChooser;
import java.util.Scanner;
import java.util.StringTokenizer;
public class FileMenuHandler implements ActionListener {
private DateGui gui;
public FileMenuHandler(DateGui gui) {
this.gui = gui;
}
public void actionPerformed(ActionEvent event) {
String menuName = event.getActionCommand();
if (menuName.equals("Open"))
openFile();//Opens file if open is selected.
else if (menuName.equals("Quit"))
System.exit(0);//Exits GUI if quit is selected.
}
private void openFile() {
JFileChooser chooser = new JFileChooser();
int status = chooser.showOpenDialog(null);
if (status == JFileChooser.APPROVE_OPTION)
readSource(chooser.getSelectedFile());//Gets the selected text file.
}
private void readSource(File chosenFile) {
//to read dates from selected file:
Scanner reader;
DateList unsorted = new UnsortedDateList();
DateList sorted = new SortedDateList();
try {
reader = new Scanner(chosenFile);
while (reader.hasNextLine()) {
String line = reader.nextLine();
StringTokenizer token = new StringTokenizer(line, ", ");
while (token.hasMoreTokens()) {
Date212 dateObject = new Date212(token.nextToken());
System.out.println(dateObject.toString());
unsorted.add(dateObject);
sorted.add(dateObject);
}
}
}
catch (FileNotFoundException ex) {
System.out.println("File not found. " + ex.getMessage());
}
catch (NumberFormatException nfe) {
System.out.println("Date contained non-number chars. " + nfe.getMessage());
}
//catch (IllegalDate212Exception e) {
//System.out.println("Illegal date. " + e.getMessage());
// }
finally
{
//Prints dates to GUI after reading and sorting through the text file.
gui.unsortedDates.append(unsorted.toString());
gui.sortedDates.append(sorted.toString());
}
}
}
______________________________________________________________________________________
//This class prints the sorted information from the text file.
public class SortedDateList extends DateList {
public SortedDateList() {
DateNode ln = new DateNode(null);
first = ln;
last = ln;
length = 0;
}
public void add(Date212 date) {
DateNode newNode = new DateNode(date);
if (first.next == null) {
last.next = newNode;
last = newNode;
length++;
return;
}
d = first;
while (d.next != null && d.next.date.compareTo(date) < 0)
d = d.next;
if (d.next == null) {
last.next = newNode;
last = newNode;
} else {
newNode.next = d.next;
d.next = newNode;
}
d = first;
length++;
}
}
________________________________________________________________________________
//This class prints the date as read from the text file.
public class UnsortedDateList extends DateList {
public UnsortedDateList() {
DateNode ln = new DateNode(null);
first = ln;
last = ln;
length = 0;
}
public void add(Date212 date) {
//adds dates to the end of the linkedlist:
DateNode newNode = new DateNode(date);
last.next = newNode;
last = newNode;
length++;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
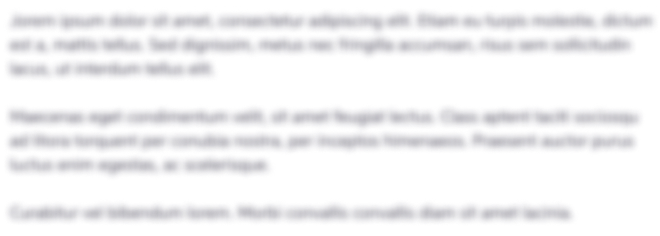
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started