Question
I have this code, the only code im allowed to add to or change is within the three segments of Lab7.java, i am very confused
I have this code, the only code im allowed to add to or change is within the three segments of Lab7.java, i am very confused as to what i should do. Movie compiles fine but i get errors when compiling Lab7 or MovieDB.
Lab7:
import java.util.*;
import java.io.*;
public class Lab7
{
public static void main(String [] args) throws IOException
{
MovieDB movies = new MovieDB(10); // Create MovieDB object. The
// size is set at 10, meaning it can hold up to 10
// movies. If we wanted (as discussed in lecture) we
// could allow for it to be resized so it could hold
// an arbitrary number of movies.
loadData(movies); // input movie data from file
getCommands(movies); // interact with user
saveData(movies); // save movie data back to file
}
public static void loadData(MovieDB movies) throws IOException
{
// Note the syntax below for creating a Scanner to a file
Scanner S = new Scanner(new FileInputStream("movieFile.txt"));
// *** CODE SEGMENT 1 *** //
// Complete this method in the following way:
// Read in the number of movies from the file
// For each movie read the data from the file and create a Movie
// object
// Add the Movie to the MovieDB object (movies) using the appropriate
// method (see MovieDB class)
int numMovies=Integer.parseInt(S.nextLine());
for(int i=0; i String movieName=S.nextLine(); String director=S.nextLine(); String studio=S.nextLine(); Double gross=Double.parseDouble(S.nextLine()); Movie movie=new Movie(movieName, director, studio, gross); //created the movie object, now add it to MovieDB object (movies) movies.add(movie); } } public static void getCommands(MovieDB movies) { Scanner inScan = new Scanner(System.in); System.out.println("Enter your choice:"); System.out.println("1. List movies"); System.out.println("2. Add new movie"); System.out.println("3. Find movie"); System.out.println("4. Quit"); String choice = inScan.nextLine(); while (true) { Movie temp; if (choice.equals("1")) { System.out.println(movies.toString()); } else if (choice.equals("2")) { // *** CODE SEGMENT 2 *** // // Complete this choice in the following way: // Prompt for and read in each of the values needed // for the new Movie object (3 strings, 1 double) // Create a new Movie object and then add it to the // MovieDB object (movies) using the correct method. System.out.println(Enter movie name); //prompting the user to enter value String movieName=inScan.nextLine(); System.out.println(Enter director name); String director=inScan.nextLine(); System.out.println(Enter studio name); String studio=inScan.nextLine(); System.out.println(Enter gross amount for movie); Double gross=Double.parseDouble(inScan.nextLine()); Movie newMovie=new Movie(movieName, director, studio, gross); //created the movie object, now add it to MovieDB object (movies) movies.add(newMovie); } else if (choice.equals("3")) { // *** CODE SEGMENT 3 *** // // Complete this choice in the following way: // Ask the user for the movie name and read it in // Call the appropriate method in the MovieDB object // (movies) to find the Movie and return it // Show the movie's info (or indicate it is not found) System.out.println(Enter movie name); String title=inScan.nextLine(); Movie movieFound=movies.findMovie(title); if(movieFound==null) //movie not found System.out.println(Movie with this title not found); else{ System.out.println(Movie information: +movieFound.toString()); } // print formatted information of movie } else { System.out.println("Good-bye"); break; // any other value -- quit } System.out.println("Enter your choice:"); System.out.println("1. List movies"); System.out.println("2. Add new movie"); System.out.println("3. Find movie"); System.out.println("4. Quit"); choice = inScan.nextLine(); } } public static void saveData(MovieDB movies) throws IOException { PrintWriter P = new PrintWriter("movieFile.txt"); // Note that we are printing the entire DB to the file with // one statement. This is because the toStringFile() method // of the MovieDB class calls the toStringFile() method of // each Movie within the DB. P.print(movies.toStringFile()); P.close(); } } Movie: import java.text.*; import java.util.*; public class Movie { private String title; private String director; private String studio; private double gross; // Constructor -- take 4 arguments and make a new Movie public Movie(String t, String d, String s, double g) { title = new String(t); director = new String(d); studio = new String(s); gross = g; } // Return a formatted string version of this Movie public String toString() { StringBuffer B = new StringBuffer(); B.append("Title: " + title + " "); B.append("Director: " + director + " "); B.append("Studio: " + studio + " "); NumberFormat formatter = NumberFormat.getCurrencyInstance(Locale.US); B.append("Gross: " + formatter.format(gross) + " "); return B.toString(); } // Return an unformatted string version of this Movie public String toStringFile() { StringBuffer B = new StringBuffer(); B.append(title + " "); B.append(director + " "); B.append(studio + " "); B.append(gross + " "); return B.toString(); } // Accessor to return title of this Movie public String getTitle() { return title; } } MovieDB: // This class is a simple database of Movie objects. Note the // instance variables and methods and read the comments carefully. public class MovieDB { // Note that we have 2 instance variables here -- an array of Movie and // an int. Since Java arrays are of fixed size once they are created, // we creating the array of a certain (large) size and then using the // int variable to keep track of how many actual movies are in it. We // don't resize here, but we could if we wanted to (as discussed in class). private Movie [] theMovies; private int numMovies; // Initialize this MovieDB public MovieDB(int size) { theMovies = new Movie[size]; numMovies = 0; } // Take already created movie and add it to the DB. This is simply putting // the new movie at the end of the array, and incrementing the int to // indicate that a new movie has been added. If no room is left in the // array, indicate that fact. public void addMovie(Movie m) { if (numMovies < theMovies.length) { theMovies[numMovies] = m; numMovies++; } else System.out.println("No room to add movie"); // Alternatively, as we discussed in lecture, we could resize the // array to make room - feel free to try this as an additional // exercise. } // Iterate through the array until the movie is found or the end of the // array is reached. Note that even though a Movie object has several // components we are searching based on the title alone. We call the // title the "key value" for the Movie. If the Movie is not found we // indicate that fact by returning null. public Movie findMovie(String title) { for (int i = 0; i < numMovies; i++) { if (theMovies[i].getTitle().equals(title)) return theMovies[i]; } return null; } // Return a formatted string containing all of the movies' info. Note // that we are calling the toString() method for each movie in the DB. public String toString() { StringBuffer B = new StringBuffer(); B.append("Movie List: "); for (int i = 0; i < numMovies; i++) B.append(theMovies[i].toString() + " "); return B.toString(); } // Similar to the method above, but now we are not formatting the // string, so we can write the data to the file. public String toStringFile() { StringBuffer B = new StringBuffer(); B.append(numMovies + " "); for (int i = 0; i < numMovies; i++) B.append(theMovies[i].toStringFile()); return B.toString(); } } movieFile.txt: 3 Star Wars George Lucas 20th Century Fox 7.98E8 Jaws Steven Spielberg Universal 4.706E8 The Return of the King Peter Jackson New Line 1.1292E9 need an answer in under 2 hours if possible, sorry for the rush
Step by Step Solution
There are 3 Steps involved in it
Step: 1
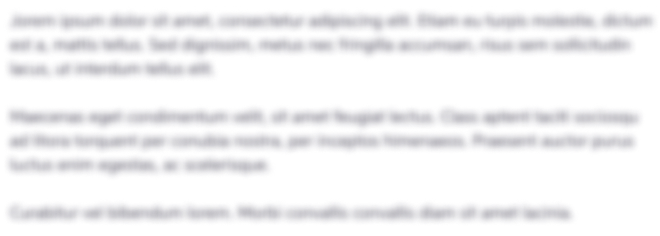
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started